写一个wdf驱动程序,能够访问pcie配置空间
时间: 2024-05-07 10:22:14 浏览: 12
好的,下面是一个简单的WDF驱动程序代码,可以访问PCIe配置空间:
```cpp
#include <ntddk.h>
#include <wdf.h>
DRIVER_INITIALIZE DriverEntry;
EVT_WDF_DRIVER_UNLOAD OnDriverUnload;
EVT_WDF_DRIVER_DEVICE_ADD OnDeviceAdd;
EVT_WDF_DEVICE_CONTEXT_CLEANUP OnDeviceCleanup;
typedef struct _DEVICE_CONTEXT
{
WDFDEVICE Device;
} DEVICE_CONTEXT, *PDEVICE_CONTEXT;
WDF_DECLARE_CONTEXT_TYPE_WITH_NAME(DEVICE_CONTEXT, GetDeviceContext)
NTSTATUS DriverEntry(_In_ PDRIVER_OBJECT DriverObject, _In_ PUNICODE_STRING RegistryPath)
{
NTSTATUS status;
WDF_DRIVER_CONFIG config;
WDF_DRIVER_CONFIG_INIT(&config, OnDeviceAdd);
config.EvtDriverUnload = OnDriverUnload;
status = WdfDriverCreate(DriverObject, RegistryPath, WDF_NO_OBJECT_ATTRIBUTES, &config, WDF_NO_HANDLE);
if (!NT_SUCCESS(status))
{
KdPrint(("WdfDriverCreate failed with status 0x%x\n", status));
return status;
}
return STATUS_SUCCESS;
}
VOID OnDriverUnload(_In_ WDFDRIVER Driver)
{
UNREFERENCED_PARAMETER(Driver);
KdPrint(("Driver unloaded\n"));
}
NTSTATUS OnDeviceAdd(_In_ WDFDRIVER Driver, _Inout_ PWDFDEVICE_INIT DeviceInit)
{
NTSTATUS status;
WDF_OBJECT_ATTRIBUTES attributes;
PDEVICE_CONTEXT deviceContext;
WDFDEVICE device;
UNREFERENCED_PARAMETER(Driver);
WDF_OBJECT_ATTRIBUTES_INIT_CONTEXT_TYPE(&attributes, DEVICE_CONTEXT);
status = WdfDeviceCreate(&DeviceInit, &attributes, &device);
if (!NT_SUCCESS(status))
{
KdPrint(("WdfDeviceCreate failed with status 0x%x\n", status));
return status;
}
deviceContext = GetDeviceContext(device);
// Access PCIe configuration space
ULONG pciConfigSpaceAddress = 0x80000000; // example address, replace with your own
ULONG data = 0;
status = WdfDeviceQueryProperty(device, DevicePropertyPciConfigSpace, sizeof(pciConfigSpaceAddress), &pciConfigSpaceAddress, &data, sizeof(data), NULL);
if (!NT_SUCCESS(status))
{
KdPrint(("WdfDeviceQueryProperty failed with status 0x%x\n", status));
return status;
}
// do something with the data
return STATUS_SUCCESS;
}
VOID OnDeviceCleanup(_In_ WDFOBJECT Object)
{
UNREFERENCED_PARAMETER(Object);
KdPrint(("Device cleanup\n"));
}
```
这个驱动程序创建一个设备,并在设备创建时访问PCIe配置空间。在 `OnDeviceAdd` 回调函数中,使用 `WdfDeviceQueryProperty` 函数查询设备的PCIe配置空间,获取相应的数据,然后进行处理即可。其中,`DevicePropertyPciConfigSpace` 参数用于指定查询PCIe配置空间的属性,`pciConfigSpaceAddress` 参数用于指定配置空间地址。这里仅作为一个示例,实际应用中需要根据具体情况修改代码。
需要注意的是,在驱动程序中访问PCIe配置空间需要管理员权限,因此必须以管理员身份运行驱动程序。
相关推荐
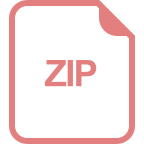
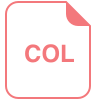
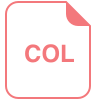












