c++插值拟合散点,插值结果曲线可视化
时间: 2023-08-16 16:07:02 浏览: 45
要在C++中实现插值拟合散点并可视化结果曲线,你可以使用第三方库(如gnuplot)来绘制图形。下面是一个示例代码,它使用了gnuplot来绘制插值结果曲线:
```cpp
#include <iostream>
#include <vector>
#include <fstream>
// 引入gnuplot库
#include "gnuplot-iostream.h"
// 拉格朗日插值函数
double lagrangeInterpolation(const std::vector<double>& x, const std::vector<double>& y, double xi) {
double result = 0.0;
int n = x.size();
for (int i = 0; i < n; i++) {
double term = y[i];
for (int j = 0; j < n; j++) {
if (j != i) {
term *= (xi - x[j]) / (x[i] - x[j]);
}
}
result += term;
}
return result;
}
int main() {
// 输入散点数据
std::vector<double> x = {1.0, 2.0, 3.0, 4.0, 5.0};
std::vector<double> y = {1.0, 4.0, 9.0, 16.0, 25.0};
// 使用插值算法得到更多的点
int numPoints = 100;
std::vector<double> xi(numPoints);
std::vector<double> yi(numPoints);
double xMin = x.front();
double xMax = x.back();
double step = (xMax - xMin) / (numPoints - 1);
for (int i = 0; i < numPoints; i++) {
xi[i] = xMin + i * step;
yi[i] = lagrangeInterpolation(x, y, xi[i]);
}
// 使用gnuplot进行可视化
Gnuplot gp;
// 绘制散点
gp << "set style data points" << std::endl;
gp << "plot '-' with points title 'Scatter Plot'" << std::endl;
gp.send1d(boost::make_tuple(x, y));
// 绘制插值曲线
gp << "set style data lines" << std::endl;
gp << "plot '-' with lines title 'Interpolation Curve'" << std::endl;
gp.send1d(boost::make_tuple(xi, yi));
return 0;
}
```
在上述代码中,我们首先使用gnuplot库来创建一个Gnuplot对象`gp`。然后,我们使用插值算法计算出更多的插值点,存储在`xi`和`yi`向量中。接下来,我们使用`gp`对象来绘制散点和插值曲线。最后,我们调用`gp.send1d`函数将数据发送给gnuplot进行绘图。
请确保将gnuplot库正确地安装在你的系统上,并将其头文件和库文件与代码正确链接。此外,你可能还需要根据你的系统和编译器设置进行相应的调整。
运行程序后,将会弹出一个窗口显示散点和插值曲线的图形。
相关推荐
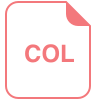

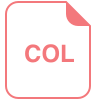











