将seg格式的点云文件转为其他格式的代码
时间: 2024-06-08 12:09:40 浏览: 15
Seg格式是一种二进制格式的点云数据文件,可以使用以下代码将其转换为其他格式(如PLY、OBJ等):
```python
import struct
def read_seg_file(file_path):
with open(file_path, 'rb') as f:
# read header
header = f.read(4)
if header != b'SEG\n':
raise ValueError('Invalid SEG file')
# read number of points
num_points = struct.unpack('<I', f.read(4))[0]
# read point data
points = []
for i in range(num_points):
x, y, z, label = struct.unpack('<fffI', f.read(16))
points.append([x, y, z, label])
return points
def write_ply_file(file_path, points):
with open(file_path, 'w') as f:
f.write('ply\n')
f.write('format ascii 1.0\n')
f.write(f'element vertex {len(points)}\n')
f.write('property float x\n')
f.write('property float y\n')
f.write('property float z\n')
f.write('property uchar red\n')
f.write('property uchar green\n')
f.write('property uchar blue\n')
f.write('element face 0\n')
f.write('end_header\n')
for point in points:
x, y, z, label = point
r = (label >> 16) & 0xff
g = (label >> 8) & 0xff
b = label & 0xff
f.write(f'{x:.6f} {y:.6f} {z:.6f} {r} {g} {b}\n')
# example usage
seg_file_path = 'example.seg'
points = read_seg_file(seg_file_path)
ply_file_path = 'example.ply'
write_ply_file(ply_file_path, points)
```
此代码将SEG文件的点云数据读取到一个列表中,然后将其写入PLY文件。如果需要将其转换为其他格式,请更改`write_ply_file`函数中的文件头和输出格式。
相关推荐
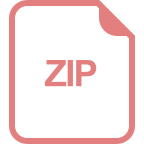










