用c++写有15个数按小由到大顺序存放在一个数组中,输入一个数,要求用折半查找法找出该数在数组中的下标。
时间: 2024-02-22 18:59:40 浏览: 89
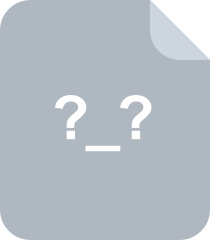
2.有15个数存放在一个数组中,输入一个数,要求用折半查找法找出该数是数组中第几个元素的值。如果该数不在数组中,则输出“无此数”。以15个数用赋初值的方法在程序

好的,下面是使用C++实现的代码:
```c++
#include <iostream>
using namespace std;
int binarySearch(int arr[], int l, int r, int x)
{
while (l <= r) {
int m = l + (r - l) / 2;
// Check if x is present at mid
if (arr[m] == x)
return m;
// If x greater, ignore left half
if (arr[m] < x)
l = m + 1;
// If x is smaller, ignore right half
else
r = m - 1;
}
// if we reach here, then element was not present
return -1;
}
int main()
{
int arr[15] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15};
int n = sizeof(arr) / sizeof(arr[0]);
int x;
cout << "Enter a number to search: ";
cin >> x;
int result = binarySearch(arr, 0, n - 1, x);
(result == -1) ? cout << "Element is not present in array"
: cout << "Element is present at index " << result;
return 0;
}
```
代码中,我们首先定义了一个长度为15的数组,然后输入一个要查找的数x,调用`binarySearch`函数进行折半查找,最后输出结果。`binarySearch`函数使用while循环实现了折半查找算法,如果找到了就返回下标,找不到就返回-1。
阅读全文
相关推荐





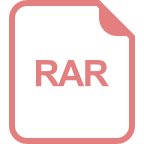
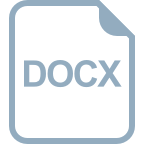
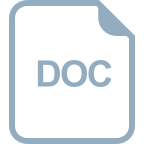
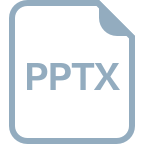
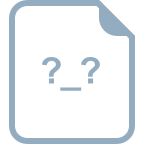
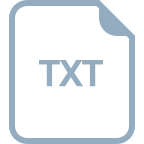
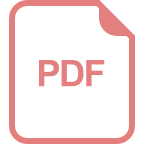
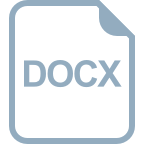
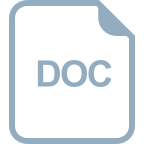
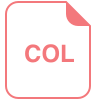