gcnet代码tensorflow
时间: 2023-07-05 13:35:31 浏览: 49
以下是使用 TensorFlow 实现的 GCNet 代码示例:
```python
import tensorflow as tf
def spatial_attention(x, ratio=8):
# 获取输入张量的尺寸
W, H, C = x.get_shape().as_list()[1:]
# 计算压缩尺寸
squeeze = tf.reduce_mean(x, axis=[1, 2], keepdims=True)
# 计算空间注意力张量
excitation = tf.layers.dense(inputs=squeeze, units=C // ratio)
excitation = tf.nn.relu(excitation)
excitation = tf.layers.dense(inputs=excitation, units=C)
excitation = tf.nn.sigmoid(excitation)
# 将空间注意力张量广播到原始张量的尺寸
excitation = tf.reshape(excitation, [-1, 1, 1, C])
excitation = tf.tile(excitation, [1, W, H, 1])
# 计算加权后的特征图
return tf.multiply(x, excitation)
def channel_attention(x, ratio=8):
# 计算平均值和最大值特征图
avg_pool = tf.reduce_mean(x, axis=[1, 2], keepdims=True)
max_pool = tf.reduce_max(x, axis=[1, 2], keepdims=True)
# 计算通道注意力张量
mlp = tf.layers.dense(inputs=tf.concat([avg_pool, max_pool], axis=-1), units=C // ratio)
mlp = tf.nn.relu(mlp)
mlp = tf.layers.dense(inputs=mlp, units=C)
mlp = tf.nn.sigmoid(mlp)
# 计算加权后的特征图
return tf.multiply(x, mlp)
def gc_block(x):
# 计算空间注意力
x_sa = spatial_attention(x)
# 计算通道注意力
x_ca = channel_attention(x)
# 将两个注意力加权后相加
x = tf.add(x_sa, x_ca)
# 使用 1x1 卷积进行特征压缩
x = tf.layers.conv2d(inputs=x, filters=C, kernel_size=1, strides=1, padding='same')
# 应用 BN 和 ReLU 激活函数
x = tf.layers.batch_normalization(inputs=x)
x = tf.nn.relu(x)
# 返回输出特征图
return x
```
以上代码实现了 GCNet 中的两种注意力机制(空间注意力和通道注意力),并使用 1x1 卷积进行特征压缩。完整的 GCNet 模型可以使用多个 GC Block 进行堆叠来实现。
相关推荐
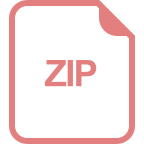
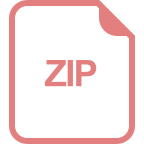
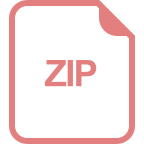













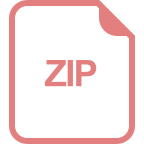