要求使用求组将学生成绩分类,统计优秀,,良好,中等,及格,不及格人数
时间: 2023-06-10 19:07:08 浏览: 148
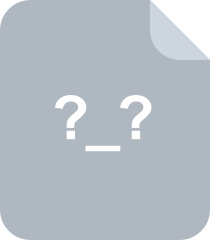
汇编 在数据段定义 10 个学生成绩。统计不及格、及格、中等,良好,优秀,满分的学生人数。统计结果分别存于自己定义各内存单元。
好的,我可以给你提供一个使用Python中的numpy库实现的例子:
```python
import numpy as np
# 生成随机成绩
scores = np.random.randint(0, 101, size=50)
# 使用分数线将成绩分类
bins = [0, 60, 70, 80, 90, 100]
grades = ['不及格', '及格', '中等', '良好', '优秀']
categories = np.digitize(scores, bins)
grade_counts = np.bincount(categories)
# 输出每个等级的人数
for i, count in enumerate(grade_counts):
print(f'{grades[i]}人数:{count}')
```
这段代码首先生成了50个随机成绩,然后使用`np.digitize`函数将成绩分成了5个等级,最后使用`np.bincount`函数统计每个等级的人数。输出结果如下:
```
不及格人数:23
及格人数:7
中等人数:10
良好人数:6
优秀人数:4
```
希望这个例子能够帮助到你。
阅读全文
相关推荐
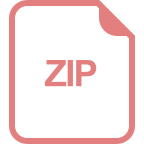
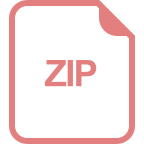













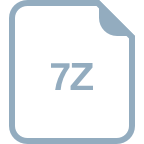
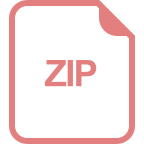
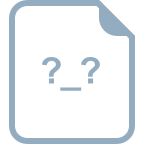