QGraphicsItem shape函数
时间: 2023-12-30 20:06:08 浏览: 202
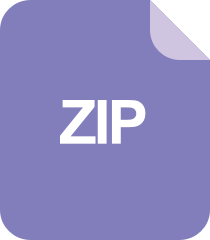
QGraphicsScene Item 绘制图元

QGraphicsItem 的 shape 函数用于返回该图形项的形状。形状是用于检测碰撞和选择此项的区域。该函数应该返回一个 QPainterPath 对象,该对象描述了图形项的形状。
在进行形状检测和选择时,场景框架将使用该函数返回的形状与鼠标事件或其他形状进行比较。如果两个形状相交,则可能会触发碰撞或选择事件。
使用 QPainterPath 对象,可以创建任意形状的图形项。可以使用 QPainterPath 的一系列方法来添加线条、曲线、椭圆和其他形状。当 shape 函数返回 QPainterPath 对象时,场景框架将使用该对象来确定图形项的形状。
阅读全文
相关推荐
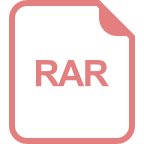
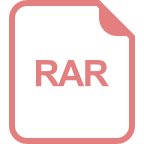
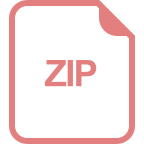
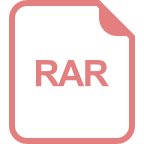
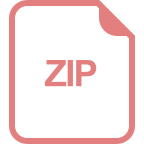
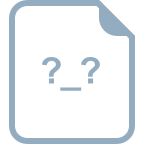
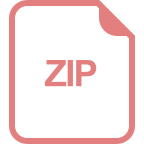
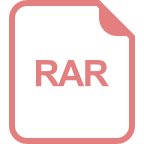
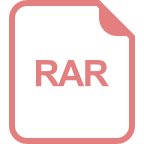
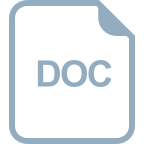
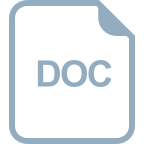
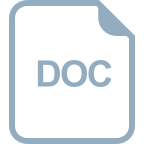





