可视化带有RGB信息的ply文件的python代码
时间: 2024-06-10 12:04:55 浏览: 295
以下是一个可视化带有RGB信息的PLY文件的简单Python代码示例:
```python
import open3d as o3d
# 加载带有RGB信息的PLY文件
mesh = o3d.io.read_triangle_mesh("example.ply")
pcd = mesh.vertices
pcd.colors = mesh.vertex_colors
# 可视化点云
o3d.visualization.draw_geometries([pcd])
```
这段代码使用Open3D库加载带有RGB信息的PLY文件,并将每个点的颜色设置为对应的RGB值。然后,它将点云可视化为一个窗口。注意,请替换文件名以匹配您自己的PLY文件。
相关问题
使用 pyvista可视化带有RGB信息的ply文件的python代码
以下是使用 pyvista 库来可视化带有 RGB 信息的 PLY 文件的 Python 代码:
```
import pyvista as pv
import numpy as np
# Load PLY file with RGB scalar field
filename = 'your_filename.ply'
mesh = pv.read(filename)
# Extract RGB arrays
red_array = mesh.point_arrays['red']
green_array = mesh.point_arrays['green']
blue_array = mesh.point_arrays['blue']
rgb_array = np.column_stack((red_array, green_array, blue_array))
# Add RGB array as scalars
mesh['RGB'] = rgb_array
# Plot mesh with RGB information
p = pv.Plotter()
p.add_mesh(mesh, scalars='RGB')
p.show()
```
如何使ply文件的mesh的指定网格变色
在使用Ply(PLY Format)文件处理三维模型时,如果想要给指定网格区域变色,通常需要借助一些三维图形库,如Python的PyOpenGL、Trimesh或者其他可视化工具。以下是基本步骤:
1. **导入所需库**:
首先,你需要安装`trimesh`库来读取和操作ply文件,以及`matplotlib`或`pyplot`来显示颜色变化。
```python
import trimesh
from matplotlib.collections import PolyCollection
```
2. **加载Ply文件**:
使用`trimesh.load_mesh`函数读取ply文件。
```python
mesh = trimesh.load('path_to_your_ply_file.ply')
```
3. **选择并标记网格**:
根据你的需求,找出你要改变颜色的特定网格部分。你可以通过索引、标签或几何条件来选取这部分。
```python
selected_face_indices = [your_selection_logic] # 例如,根据顶点坐标或face属性筛选
```
4. **创建彩色网格**:
创建一个新的PolyCollection对象,并将选定面的颜色设置为你想要的效果。
```python
face_colors = plt.cm.get_cmap('viridis')([0., 0.5]) # 用颜色映射设置颜色
colored_faces = mesh.faces[selected_face_indices]
vertex_colors = face_colors[:, :3] # 取出RGB值
collection = PolyCollection(vertex_colors, facecolors=vertex_colors)
```
5. **添加到渲染场景**:
将这个PolyCollection对象添加到你的渲染图中。
```python
ax = mesh.plot() # 如果使用trimesh自带的渲染
ax.add_collection(collection) # 或者在matplotlib环境中
# 如果需要保存图片,可以使用ax.figure.savefig()
```
6. **显示结果**:
最后显示整个图形。
```python
plt.show()
```
阅读全文
相关推荐
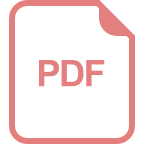
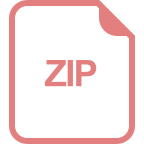
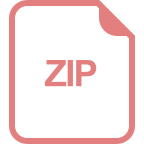
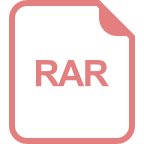
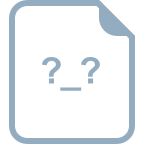
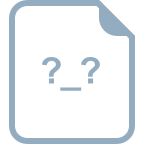
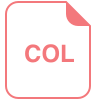





