int sum=0; if(flag==1){ for(int i=0;i<month-1;i++){//因为如果是12月的某一天,整数月有11个再加上12月的多少天 sum+=a[i]; } } else{ for(int i=0;i<month-1;i++){ sum+=b[i]; } }
时间: 2023-06-26 13:02:35 浏览: 50
这段代码是一个计算某个日期之前所有天数的程序段。变量sum初始值为0,flag为1时表示是闰年,flag为0时表示是平年。程序使用了两个数组a和b,分别存储了闰年和平年每个月的天数。根据输入的日期的月份和年份,选择相应的数组,然后将该日期之前每个月的天数累加到sum中,最后返回sum即可。需要注意的是,如果输入的月份是12月,那么需要加上12月的天数。
相关问题
#日历程序 Lunar=(1,3,5,7,8,10,12) def IsleapYear(year): flag =False if(year%4==0 and year%100!=0) or year%400==0: flag = True return flag def calculation(year,month): sum = 0 s_year=1990 while s_year < year-1: s_year +=1 if IsleapYear(s_year): sum+=366 else: sum+=365 s_month=1 while s_month<month: if s_month in Lunar: sum += 31 elif s_month==2: if IsleapYear(year): sum+=29 else: sum+=28 else: sum+=30 s_month+=1 return sum def display(sum,year,month): week=(sum+1)%7 if month in Lunar: day=31 elif month==2: if IsleapYear(year): day=29 else: day=28 else: day=30 print("日 一 二 三 四 五 六") count = 0 space = 0 while space<=week: space+=1 count+=1 print(" ",end="") if count%7==0: print(" ",end="") days=1 while days<=day: print(days," ",end="") days+=1 count+=1 if count %7 ==0: print("") def main(): year =int( input("输入年:")) if year<1990 or year>9999: print("year输入错误") main() month = int(input("输入月:")) if month<1 or month > 12: print("month输入错误") main() sums=calculation(year,month) display(sums,year,month) if __name__=="__main__": while True: main() print() choose=input("是否继续:(y/n)") if choose in("n","N"): break怎么用C语言表示
以下是用 C 语言实现该日历程序的示例代码:
#include <stdio.h>
int IsLeapYear(int year) {
int flag = 0;
if ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0) {
flag = 1;
}
return flag;
}
int Calculation(int year, int month) {
int sum = 0;
int s_year = 1990;
while (s_year < year - 1) {
s_year++;
if (IsLeapYear(s_year)) {
sum += 366;
} else {
sum += 365;
}
}
int s_month = 1;
while (s_month < month) {
if (s_month == 1 || s_month == 3 || s_month == 5 || s_month == 7 || s_month == 8 || s_month == 10 || s_month == 12) {
sum += 31;
} else if (s_month == 2) {
if (IsLeapYear(year)) {
sum += 29;
} else {
sum += 28;
}
} else {
sum += 30;
}
s_month++;
}
return sum;
}
void Display(int sum, int year, int month) {
int week = (sum + 1) % 7;
int day;
if (month == 1 || month == 3 || month == 5 || month == 7 || month == 8 || month == 10 || month == 12) {
day = 31;
} else if (month == 2) {
if (IsLeapYear(year)) {
day = 29;
} else {
day = 28;
}
} else {
day = 30;
}
printf("日 一 二 三 四 五 六\n");
int count = 0;
int space = 0;
while (space <= week) {
space++;
count++;
printf(" ");
if (count % 7 == 0) {
printf(" ");
}
}
int days = 1;
while (days <= day) {
printf("%-3d ", days);
days++;
count++;
if (count % 7 == 0) {
printf("\n");
}
}
}
int main() {
int year, month;
char choose;
do {
printf("输入年:");
scanf("%d", &year);
if (year < 1990 || year > 9999) {
printf("year输入错误\n");
continue;
}
printf("输入月:");
scanf("%d", &month);
if (month < 1 || month > 12) {
printf("month输入错误\n");
continue;
}
int sums = Calculation(year, month);
Display(sums, year, month);
printf("\n是否继续:(y/n)");
scanf(" %c", &choose);
} while (choose == 'y' || choose == 'Y');
return 0;
}
用c编写一个,#include <stdio.h> #include <string.h> #define N 100 struct birth { int year; int month; int day; }; struct person { char ID[19]; char name[20]; struct birth birthday; int flag;//校验位是否正确, 1代表正确,0代表错误 }; int read(struct person p[]) { FILE *fp; int count=0; fp=fopen("person.txt",'r'); if(fp==NULL){ printf("Failed to open file.\n"); return -1; } while(fscanf(fp,"%s%s%d%d%d%d",p[count].ID,p[count].name, &p[count].birthday.year,&p[count].birthday.month, &p[count].birthday.day,&p[count].flag)!=EOF){ count++; } fclose(fp); return count; } int checkID(char *ID) { int weight[18]={7,9,10,5,8,4,2,1,6,3,7,9,10,5,8,4,2};//ID号码权重 char checkcode[11]={1,0,'X',9,8,7,6,5,4,3,2};//校验码字符 int sum=0;//加权和 int i; if(strlen(ID)!=18){ return 0; } for(i=0;i<17;i++){ if(!isdigit(ID[i])){//isdigit=? return 0; } } for(i=0;i<17;i++){ // sum+=ID[i]*weight[i]; sum+=(ID[i]-'0')*weight[i]; } int code; code=sum%11; if(ID[17]==checkcode[code]){ return 1; } else{ return 0; } } struct birth get_birth(char *ID) { int i,j; struct birth b; char year_s[5]={0}, month_s[3]={0}, day_s[3]={0}; for(i=6,j=0;j<4;i++,j++){ year_s[j]=ID[i]; } for(i=10,j=0;j<2;i++,j++){ month_s[j]=ID[i]; } for(i=12,j=0;j<2;i++,j++){ day_s[j]=ID[i]; } //将字符串转换为整型 b.year=(year_s[0]-'0')*1000+(year_s[1]-'0')*100+ (year_s[2]-'0')*10+(year_s[3]-'0'); b.month=(month_s[0]-'0')*10+(month_s[1]-'0'); b.day=(day_s[0]-'0')*10+(day_s[1]-'0'); return b; } void get_all_person(struct person p[], int n) { int i; for(i=0;i<n;i++){ struct birth b=get_birth(p[i].ID); if(checkID(p[i].ID)){ p[i].birthday=b; p[i].flag=1; } else{ p[i].flag=0; } } }void save(struct person p[],int n); 将身份证号码正确的数据(身份证号码、姓名、出生年月日)写入文件person_checked.txt的函数。其中数组 p 中存放 n 个公民信息。
void save(struct person p[], int n) {
FILE *fp;
fp = fopen("person_checked.txt", "w");
if (fp == NULL) {
printf("Failed to open file.\n");
return;
}
int i;
for (i = 0; i < n; i++) {
if (p[i].flag == 1) {
fprintf(fp, "%s %s %d-%02d-%02d\n", p[i].ID, p[i].name, p[i].birthday.year, p[i].birthday.month, p[i].birthday.day);
}
}
fclose(fp);
}
相关推荐
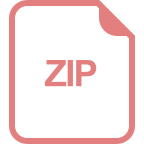
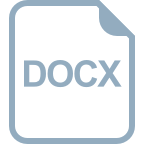









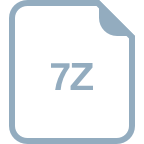
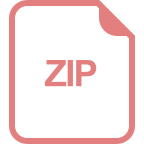
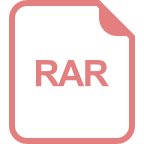