请将上面代码的输出格式去掉’‘
时间: 2024-03-24 15:39:32 浏览: 17
好的,只需要将print语句中的end参数设置为默认值即可,即不使用任何分隔符,代码如下:
```python
# 读取输入的整数列表
nums = list(map(int, input().split(',')))
# 找到最大元素的下标
max_index = find_max_index(nums)
# 打印输出所有的下标
for i in max_index:
print(i)
```
这样修改后,程序将每行输出一个下标,不再有任何分隔符。
相关问题
如何把上面代码输出结果里的单引号去掉
您可以使用字符串的 replace() 方法来去掉单引号,例如:
```
result = result.replace("'", "")
```
这将把结果中的所有单引号替换为空字符串,从而去掉它们。
将上面的代码完整的写出来
下面是一个完整的MATLAB程序,用于将gprMax输出文件中的B-scan图像转换为去除直达波的图像:
```matlab
% 读取.gprMax输出文件
data = load('output.mat');
scan = data.data;
% 定义均值过滤函数
function scan_new = remove_direct_wave(scan, kernel_size)
% 进行水平方向上的平均滤波得到平均图像
scan_hmean = imgaussfilt(scan, [kernel_size, 1]);
% 进行垂直方向上的平均滤波得到全局平均值
scan_vmean = imgaussfilt(scan_hmean, [1, kernel_size]);
global_mean = mean(scan_vmean(:));
% 对原始图像进行剪切
scan_new = max(scan - global_mean, 0);
end
% 对B-scan图像进行去除直达波处理
kernel_size = 5;
scan_new = remove_direct_wave(scan, kernel_size);
% 绘制去除直达波后的B-scan图像
figure;
imagesc(scan_new);
colormap('gray');
axis image;
colorbar;
title('B-scan after removing direct wave');
```
在上面的代码中,`output.mat`表示gprMax的输出文件名,`kernel_size`为均值滤波器的大小,根据具体情况进行调整。函数`remove_direct_wave`将原始B-scan图像进行去除直达波处理,并返回去除直达波后的图像`scan_new`。程序最后会绘制出去除直达波后的B-scan图像,并添加颜色条和标题。
相关推荐
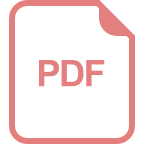
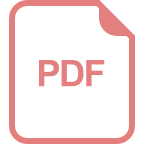
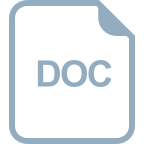












