利用pthread_cond_signal和pthread_cond_wait实现c语言代码,功能要求为:创建三个线程,用来交替打印0、基数、偶数,实现打印结果如01020304050607
时间: 2023-11-04 07:40:35 浏览: 88
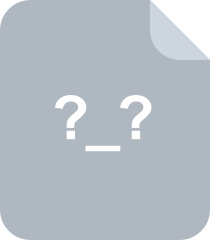
用c语言实现打印函数
下面是一个示例的C语言代码,使用pthread_cond_signal和pthread_cond_wait实现交替打印0、基数和偶数的功能:
```c
#include <stdio.h>
#include <pthread.h>
pthread_mutex_t mutex;
pthread_cond_t cond;
int count = 0;
void* print_number(void* arg) {
int num_type = *(int*)arg;
while (count <= 7) {
pthread_mutex_lock(&mutex);
// 打印0
if (num_type == 0 && count == 0) {
printf("0");
count++;
pthread_cond_signal(&cond);
}
// 打印偶数
else if (num_type == 1 && count % 2 == 0) {
printf("%d", count);
count++;
pthread_cond_signal(&cond);
}
// 打印奇数
else if (num_type == 2 && count % 2 != 0) {
printf("%d", count);
count++;
pthread_cond_signal(&cond);
}
else {
pthread_cond_wait(&cond, &mutex);
}
pthread_mutex_unlock(&mutex);
}
return NULL;
}
int main() {
pthread_t threads[3];
int thread_args[3] = {0, 1, 2};
pthread_mutex_init(&mutex, NULL);
pthread_cond_init(&cond, NULL);
for (int i = 0; i < 3; i++) {
pthread_create(&threads[i], NULL, print_number, &thread_args[i]);
}
for (int i = 0; i < 3; i++) {
pthread_join(threads[i], NULL);
}
pthread_mutex_destroy(&mutex);
pthread_cond_destroy(&cond);
printf("\n");
return 0;
}
```
在这个示例中,我们使用了一个互斥锁mutex和一个条件变量cond来实现线程间的同步。三个线程分别代表打印0、偶数和奇数的功能。在每个线程中,我们使用了互斥锁来保护共享变量count,并使用条件变量来进行线程的阻塞和唤醒。通过调用pthread_cond_wait和pthread_cond_signal来实现线程间的交替打印。
注意,在主线程中,我们使用pthread_join来等待所有子线程的结束,以确保打印完所有数字之后再打印换行符。
阅读全文
相关推荐
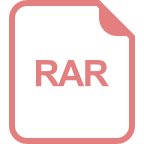

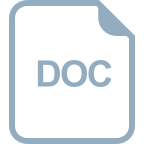













