使用pthread_cond_signal进行线程同步的代码示例和接卸
时间: 2023-12-02 22:36:23 浏览: 107
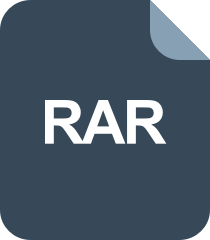
有关线程同步的几个代码实例

下面是一个使用pthread_cond_signal进行线程同步的代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
pthread_mutex_t mutex;
pthread_cond_t cond;
int count = 0;
void* thread_func(void* arg) {
pthread_mutex_lock(&mutex);
count++; // 假设某个线程需要增加count的值
printf("Thread %ld increased count to %d\n", pthread_self(), count);
pthread_cond_signal(&cond); // 发送信号通知其他线程条件已满足
pthread_mutex_unlock(&mutex);
return NULL;
}
int main() {
pthread_t threads[5];
pthread_mutex_init(&mutex, NULL);
pthread_cond_init(&cond, NULL);
for (int i = 0; i < 5; i++) {
pthread_create(&threads[i], NULL, thread_func, NULL);
}
pthread_mutex_lock(&mutex);
while (count < 5) { // 等待count增加到5
pthread_cond_wait(&cond, &mutex); // 阻塞等待条件变量的信号
}
printf("Main thread received signal, count is %d\n", count);
pthread_mutex_unlock(&mutex);
for (int i = 0; i < 5; i++) {
pthread_join(threads[i], NULL);
}
pthread_mutex_destroy(&mutex);
pthread_cond_destroy(&cond);
return 0;
}
```
在上面的示例中,主线程创建了5个子线程,并且每个子线程在临界区内增加count的值。主线程通过等待条件变量的信号来阻塞等待,直到count的值增加到5。子线程通过发送信号通知主线程条件已满足。
注意,在使用条件变量进行线程同步时,需要搭配互斥锁(mutex)使用,以确保多个线程之间的互斥和同步。在示例中,使用了pthread_mutex_lock和pthread_mutex_unlock来保护临界区的操作。
阅读全文
相关推荐


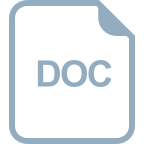












