用c语言写一个飞机大战的代码
时间: 2023-12-31 09:04:49 浏览: 18
这里是一个简单的飞机大战游戏的 C 语言代码,仅供参考:
```c
#include <stdio.h>
#include <conio.h>
#include <stdlib.h>
#include <windows.h>
#include <time.h>
#define WIDTH 50
#define HEIGHT 30
int score = 0; // 记录得分
char ch; // 存储用户输入的字符
// 定义飞机结构体
struct plane {
int x;
int y;
int life;
} my_plane;
// 定义子弹结构体
struct bullet {
int x;
int y;
int life;
} my_bullet;
// 定义敌机结构体
struct enemy_plane {
int x;
int y;
int life;
} enemy[5];
// 初始化游戏
void init_game() {
srand((unsigned)time(NULL)); // 设置随机数种子
my_plane.x = WIDTH / 2;
my_plane.y = HEIGHT - 3;
my_plane.life = 1;
my_bullet.life = 0;
for (int i = 0; i < 5; i++) {
enemy[i].x = rand() % WIDTH;
enemy[i].y = rand() % 5;
enemy[i].life = 1;
}
}
// 绘制游戏界面
void draw_game() {
system("cls"); // 清屏
for (int i = 0; i < WIDTH + 2; i++) {
printf("-");
}
printf("\n");
for (int i = 0; i < HEIGHT; i++) {
printf("|");
for (int j = 0; j < WIDTH; j++) {
if (i == my_plane.y && j == my_plane.x) {
printf("*");
}
else if (i == my_bullet.y && j == my_bullet.x && my_bullet.life == 1) {
printf("|");
}
else {
int flag = 0;
for (int k = 0; k < 5; k++) {
if (i == enemy[k].y && j == enemy[k].x && enemy[k].life == 1) {
printf("E");
flag = 1;
break;
}
}
if (flag == 0) {
printf(" ");
}
}
}
printf("|\n");
}
for (int i = 0; i < WIDTH + 2; i++) {
printf("-");
}
printf("\n");
printf("Score: %d\n", score);
}
// 移动飞机
void move_plane(char ch) {
switch (ch) {
case 'a':
if (my_plane.x > 0) {
my_plane.x--;
}
break;
case 'd':
if (my_plane.x < WIDTH - 1) {
my_plane.x++;
}
break;
case 'w':
if (my_plane.y > 0) {
my_plane.y--;
}
break;
case 's':
if (my_plane.y < HEIGHT - 1) {
my_plane.y++;
}
break;
}
}
// 发射子弹
void fire() {
if (my_bullet.life == 0) {
my_bullet.x = my_plane.x;
my_bullet.y = my_plane.y - 1;
my_bullet.life = 1;
}
}
// 移动子弹
void move_bullet() {
if (my_bullet.life == 1) {
my_bullet.y--;
if (my_bullet.y < 0) {
my_bullet.life = 0;
}
}
}
// 移动敌机
void move_enemy() {
for (int i = 0; i < 5; i++) {
if (enemy[i].life == 1) {
enemy[i].y++;
if (enemy[i].y >= HEIGHT) {
enemy[i].x = rand() % WIDTH;
enemy[i].y = rand() % 5;
}
}
}
}
// 检测碰撞
void check_collision() {
for (int i = 0; i < 5; i++) {
if (enemy[i].life == 1 && my_bullet.life == 1 && my_bullet.x == enemy[i].x && my_bullet.y == enemy[i].y) {
enemy[i].life = 0;
my_bullet.life = 0;
score += 10;
}
if (enemy[i].life == 1 && my_plane.x == enemy[i].x && my_plane.y == enemy[i].y) {
enemy[i].life = 0;
my_plane.life = 0;
break;
}
}
}
// 游戏主函数
int main() {
init_game();
while (my_plane.life) {
draw_game();
if (_kbhit()) {
ch = _getch();
move_plane(ch);
if (ch == ' ') {
fire();
}
}
move_bullet();
move_enemy();
check_collision();
Sleep(50); // 延时50毫秒
}
return 0;
}
```
这个游戏比较简单,只有一个玩家飞机和五个敌机,玩家飞机可以移动和发射子弹,敌机随机移动。当子弹击中敌机时,得分加10分;当敌机撞到玩家飞机时,游戏结束。
阅读全文
相关推荐







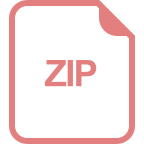





