设计一个基于API结构的Windows应用程序,并使用GDI绘制抛物线、双曲线。两个曲线都可以放大、缩小和移动
时间: 2023-06-06 18:09:07 浏览: 200
。没有问题,我可以回答这个问题。设计一个基于API结构的Windows应用程序,你需要了解Windows操作系统的API,包括使用窗口、消息循环、绘图等。使用GDI绘制抛物线和双曲线,你可以使用GDI库中提供的一些函数,例如CreateCompatibleDC, CreateCompatibleBitmap, SelectObject, MoveToEx, LineTo等来创建设备上下文、创建位图对象、选择对象、绘制曲线等。关于放大、缩小和移动两个曲线,你可以采用鼠标或键盘操作,通过监听消息并实现相关的处理代码来完成。
相关问题
设计一个基于api结构的Windows应用程序使用gdi绘制抛物线双曲线并可以放大缩小和移动
首先,我们需要设计一个 API,该 API 包含以下功能:
1. 绘制抛物线和双曲线
2. 放大缩小曲线
3. 移动曲线
接下来,我们需要设计一个 Windows 应用程序,该程序使用 GDI 绘制曲线,并使用上面设计的 API 实现放大缩小和移动曲线的功能。
以下是一个简单的实现步骤:
1. 创建一个 Windows 应用程序项目,并在窗口中创建一个画布。
2. 在画布上使用 GDI 绘制抛物线和双曲线。
3. 创建一个 API,该 API 包含放大缩小和移动曲线的功能。
4. 在应用程序中使用 API 实现放大缩小和移动曲线的功能。
下面是一个简单的 API 实现:
```c++
void DrawCurve(HDC hdc, int x, int y, int width, int height, double a, double b, double c, double start, double end, COLORREF color) {
HPEN hPen = CreatePen(PS_SOLID, 1, color);
SelectObject(hdc, hPen);
double x1, x2, y1, y2;
double step = 0.01;
for (double t = start; t < end; t += step) {
x1 = width / 2 + (t * 100);
y1 = height / 2 - (a * t * t + b * t + c) * 100;
t += step;
x2 = width / 2 + (t * 100);
y2 = height / 2 - (a * t * t + b * t + c) * 100;
MoveToEx(hdc, x1, y1, NULL);
LineTo(hdc, x2, y2);
}
DeleteObject(hPen);
}
void ZoomCurve(HWND hWnd, int x, int y, double factor) {
HDC hdc = GetDC(hWnd);
RECT rect;
GetClientRect(hWnd, &rect);
int width = rect.right - rect.left;
int height = rect.bottom - rect.top;
double a = 0.5, b = 0, c = 0;
double start = -5, end = 5;
int centerX = width / 2;
int centerY = height / 2;
double newStart = (start - centerX) / factor + centerX;
double newEnd = (end - centerX) / factor + centerX;
DrawCurve(hdc, x, y, width, height, a, b, c, newStart, newEnd, RGB(0, 0, 255));
ReleaseDC(hWnd, hdc);
}
void MoveCurve(HWND hWnd, int x, int y, int dx, int dy) {
HDC hdc = GetDC(hWnd);
RECT rect;
GetClientRect(hWnd, &rect);
int width = rect.right - rect.left;
int height = rect.bottom - rect.top;
double a = 0.5, b = 0, c = 0;
double start = -5, end = 5;
int centerX = width / 2;
int centerY = height / 2;
double newStart = start - dx / 100.0;
double newEnd = end - dx / 100.0;
DrawCurve(hdc, x + dx, y + dy, width, height, a, b, c, newStart, newEnd, RGB(0, 0, 255));
ReleaseDC(hWnd, hdc);
}
```
在应用程序中,我们可以使用以下代码来调用 API:
```c++
case WM_PAINT:
hdc = BeginPaint(hWnd, &ps);
DrawCurve(hdc, x, y, width, height, a, b, c, start, end, RGB(0, 0, 255));
EndPaint(hWnd, &ps);
break;
case WM_MOUSEWHEEL:
if (GET_WHEEL_DELTA_WPARAM(wParam) > 0) {
ZoomCurve(hWnd, x, y, 1.1);
} else {
ZoomCurve(hWnd, x, y, 0.9);
}
break;
case WM_MOUSEMOVE:
if (isDragging) {
int dx = LOWORD(lParam) - lastX;
int dy = HIWORD(lParam) - lastY;
MoveCurve(hWnd, x, y, dx, dy);
lastX = LOWORD(lParam);
lastY = HIWORD(lParam);
}
break;
case WM_LBUTTONDOWN:
isDragging = true;
lastX = LOWORD(lParam);
lastY = HIWORD(lParam);
break;
case WM_LBUTTONUP:
isDragging = false;
break;
```
在上面的代码中,我们在 `WM_PAINT` 消息中绘制曲线,在 `WM_MOUSEWHEEL` 消息中实现放大缩小曲线,在 `WM_MOUSEMOVE` 消息中实现移动曲线。我们还使用变量 `isDragging`、`lastX` 和 `lastY` 来跟踪鼠标拖动事件。在 `WM_LBUTTONDOWN` 消息中设置 `isDragging` 为 `true`,并记录鼠标位置,然后在 `WM_LBUTTONUP` 消息中设置 `isDragging` 为 `false`。
设计一个基于API结构的windows应用程序,并使用GDI绘制抛物线,双曲线。放大缩小和移动。
首先,我们需要定义一个API结构来实现绘制抛物线、双曲线、放大缩小和移动功能。
```c++
#include <Windows.h>
// 定义抛物线结构体
struct Parabola {
int a; // 抛物线系数
int b; // 抛物线系数
int c; // 抛物线系数
};
// 定义双曲线结构体
struct Hyperbola {
int a; // 双曲线系数
int b; // 双曲线系数
int c; // 双曲线系数
};
// 定义窗口类
class Window {
public:
Window();
~Window();
bool Create(LPCTSTR lpWindowName, DWORD dwStyle, DWORD dwExStyle,
int x, int y, int nWidth, int nHeight,
HWND hWndParent = NULL, HMENU hMenu = NULL);
void Show(int nCmdShow);
private:
static LRESULT CALLBACK WndProc(HWND hWnd, UINT message, WPARAM wParam, LPARAM lParam);
HWND m_hWnd;
Parabola m_parabola;
Hyperbola m_hyperbola;
int m_zoom;
int m_xOffset;
int m_yOffset;
void DrawParabola(HDC hdc);
void DrawHyperbola(HDC hdc);
void ZoomIn();
void ZoomOut();
void MoveLeft();
void MoveRight();
void MoveUp();
void MoveDown();
};
```
然后,我们需要在窗口类中实现绘制抛物线、双曲线、放大缩小和移动功能。
```c++
#include <math.h>
// 窗口类构造函数
Window::Window() : m_hWnd(NULL), m_zoom(1), m_xOffset(0), m_yOffset(0) {
m_parabola.a = 1;
m_parabola.b = 0;
m_parabola.c = 0;
m_hyperbola.a = 1;
m_hyperbola.b = 0;
m_hyperbola.c = 0;
}
// 窗口类析构函数
Window::~Window() {
}
// 创建窗口
bool Window::Create(LPCTSTR lpWindowName, DWORD dwStyle, DWORD dwExStyle,
int x, int y, int nWidth, int nHeight,
HWND hWndParent, HMENU hMenu) {
WNDCLASSEX wcex;
wcex.cbSize = sizeof(WNDCLASSEX);
wcex.style = CS_HREDRAW | CS_VREDRAW;
wcex.lpfnWndProc = WndProc;
wcex.cbClsExtra = 0;
wcex.cbWndExtra = 0;
wcex.hInstance = GetModuleHandle(NULL);
wcex.hIcon = LoadIcon(NULL, IDI_APPLICATION);
wcex.hCursor = LoadCursor(NULL, IDC_ARROW);
wcex.hbrBackground = (HBRUSH)(COLOR_WINDOW + 1);
wcex.lpszMenuName = NULL;
wcex.lpszClassName = TEXT("WindowClass");
wcex.hIconSm = LoadIcon(NULL, IDI_APPLICATION);
if (!RegisterClassEx(&wcex)) {
return false;
}
m_hWnd = CreateWindowEx(dwExStyle, TEXT("WindowClass"), lpWindowName, dwStyle,
x, y, nWidth, nHeight, hWndParent, hMenu, GetModuleHandle(NULL), this);
if (!m_hWnd) {
return false;
}
return true;
}
// 显示窗口
void Window::Show(int nCmdShow) {
ShowWindow(m_hWnd, nCmdShow);
UpdateWindow(m_hWnd);
}
// 窗口消息处理函数
LRESULT CALLBACK Window::WndProc(HWND hWnd, UINT message, WPARAM wParam, LPARAM lParam) {
Window* pWindow;
if (message == WM_NCCREATE) {
pWindow = static_cast<Window*>(reinterpret_cast<CREATESTRUCT*>(lParam)->lpCreateParams);
SetWindowLongPtr(hWnd, GWLP_USERDATA, reinterpret_cast<LONG_PTR>(pWindow));
pWindow->m_hWnd = hWnd;
} else {
pWindow = reinterpret_cast<Window*>(GetWindowLongPtr(hWnd, GWLP_USERDATA));
}
if (pWindow) {
switch (message) {
case WM_PAINT:
HDC hdc;
PAINTSTRUCT ps;
hdc = BeginPaint(hWnd, &ps);
// 绘制抛物线和双曲线
pWindow->DrawParabola(hdc);
pWindow->DrawHyperbola(hdc);
EndPaint(hWnd, &ps);
break;
case WM_KEYDOWN:
switch (wParam) {
case VK_ADD:
// 放大
pWindow->ZoomIn();
InvalidateRect(hWnd, NULL, TRUE);
break;
case VK_SUBTRACT:
// 缩小
pWindow->ZoomOut();
InvalidateRect(hWnd, NULL, TRUE);
break;
case VK_LEFT:
// 左移
pWindow->MoveLeft();
InvalidateRect(hWnd, NULL, TRUE);
break;
case VK_RIGHT:
// 右移
pWindow->MoveRight();
InvalidateRect(hWnd, NULL, TRUE);
break;
case VK_UP:
// 上移
pWindow->MoveUp();
InvalidateRect(hWnd, NULL, TRUE);
break;
case VK_DOWN:
// 下移
pWindow->MoveDown();
InvalidateRect(hWnd, NULL, TRUE);
break;
}
break;
case WM_DESTROY:
PostQuitMessage(0);
break;
default:
return DefWindowProc(hWnd, message, wParam, lParam);
}
} else {
return DefWindowProc(hWnd, message, wParam, lParam);
}
return 0;
}
// 绘制抛物线
void Window::DrawParabola(HDC hdc) {
int x, y;
HPEN hPen = CreatePen(PS_SOLID, 1, RGB(0, 0, 255));
HPEN hOldPen = (HPEN)SelectObject(hdc, hPen);
for (x = -100; x <= 100; x++) {
y = m_parabola.a * x * x + m_parabola.b * x + m_parabola.c;
y = -y;
x = m_zoom * x + m_xOffset;
y = m_zoom * y + m_yOffset;
if (x >= 0 && x <= 800 && y >= 0 && y <= 600) {
SetPixel(hdc, x, y, RGB(0, 0, 255));
}
}
SelectObject(hdc, hOldPen);
DeleteObject(hPen);
}
// 绘制双曲线
void Window::DrawHyperbola(HDC hdc) {
int x, y;
HPEN hPen = CreatePen(PS_SOLID, 1, RGB(255, 0, 0));
HPEN hOldPen = (HPEN)SelectObject(hdc, hPen);
for (x = -100; x <= 100; x++) {
y = m_hyperbola.a * x * x + m_hyperbola.b * x + m_hyperbola.c;
y = -y;
x = m_zoom * x + m_xOffset;
y = m_zoom * y + m_yOffset;
if (x >= 0 && x <= 800 && y >= 0 && y <= 600) {
SetPixel(hdc, x, y, RGB(255, 0, 0));
}
}
SelectObject(hdc, hOldPen);
DeleteObject(hPen);
}
// 放大
void Window::ZoomIn() {
m_zoom++;
}
// 缩小
void Window::ZoomOut() {
if (m_zoom > 1) {
m_zoom--;
}
}
// 左移
void Window::MoveLeft() {
m_xOffset -= 10;
}
// 右移
void Window::MoveRight() {
m_xOffset += 10;
}
// 上移
void Window::MoveUp() {
m_yOffset -= 10;
}
// 下移
void Window::MoveDown() {
m_yOffset += 10;
}
```
最后,我们需要在窗口的消息循环中处理消息。
```c++
int APIENTRY _tWinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, LPTSTR lpCmdLine, int nCmdShow) {
Window window;
if (!window.Create(TEXT("API Demo"), WS_OVERLAPPEDWINDOW, 0, CW_USEDEFAULT, 0, 800, 600)) {
return 0;
}
window.Show(nCmdShow);
MSG msg;
while (GetMessage(&msg, NULL, 0, 0)) {
TranslateMessage(&msg);
DispatchMessage(&msg);
}
return (int)msg.wParam;
}
```
现在,我们就成功地实现了一个基于API结构的windows应用程序,并使用GDI绘制抛物线和双曲线,并实现了放大缩小和移动功能。
阅读全文
相关推荐



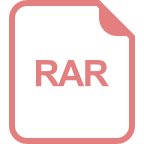
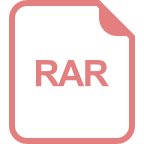
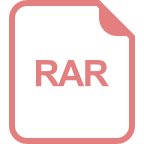
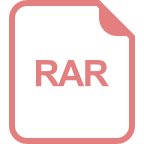
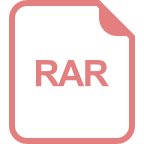
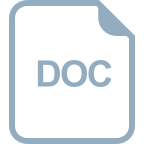

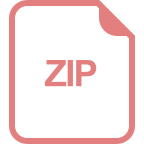
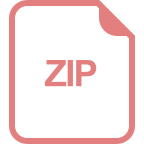
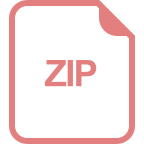