使用vscode设计一个基于API结构的Windows应用程序,并使用GDI绘制抛物线,双曲线。放大缩小和移动,给出代码实现
时间: 2024-05-05 14:21:44 浏览: 14
首先,我们需要创建一个基于API结构的Windows应用程序。以下是一个简单的代码示例:
```c++
#include <windows.h>
LRESULT CALLBACK WndProc(HWND hwnd, UINT message, WPARAM wParam, LPARAM lParam);
int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, LPSTR lpCmdLine, int nCmdShow) {
// 创建窗口类
WNDCLASSEX wc;
ZeroMemory(&wc, sizeof(WNDCLASSEX));
wc.cbSize = sizeof(WNDCLASSEX);
wc.style = CS_HREDRAW | CS_VREDRAW;
wc.lpfnWndProc = WndProc;
wc.hInstance = hInstance;
wc.hCursor = LoadCursor(NULL, IDC_ARROW);
wc.lpszClassName = "MyClass";
RegisterClassEx(&wc);
// 创建窗口
HWND hwnd = CreateWindowEx(0, "MyClass", "My Window", WS_OVERLAPPEDWINDOW, 100, 100, 800, 600, NULL, NULL, hInstance, NULL);
ShowWindow(hwnd, nCmdShow);
// 消息循环
MSG msg;
while (GetMessage(&msg, NULL, 0, 0)) {
TranslateMessage(&msg);
DispatchMessage(&msg);
}
return msg.wParam;
}
LRESULT CALLBACK WndProc(HWND hwnd, UINT message, WPARAM wParam, LPARAM lParam) {
switch (message) {
case WM_DESTROY:
PostQuitMessage(0);
break;
default:
return DefWindowProc(hwnd, message, wParam, lParam);
}
return 0;
}
```
在这个示例中,我们创建了一个窗口类和一个窗口,并使用消息循环来处理窗口消息。现在我们需要添加绘图代码来绘制抛物线和双曲线,并实现放大缩小和移动功能。
我们可以使用GDI来绘制图形。以下是一个简单的函数,用于绘制一条抛物线:
```c++
void DrawParabola(HDC hdc, int x, int y, int width, int height) {
int a = width / 4;
int b = height / 4;
int cx = x + width / 2;
int cy = y + height / 2;
for (int i = -width / 2; i <= width / 2; i++) {
int j = cy - (b * i * i) / a / a;
SetPixel(hdc, cx + i, j, RGB(255, 0, 0));
}
}
```
这个函数接受一个设备上下文(HDC)、一个坐标点(x,y)和一个宽度和高度。它使用这些参数来计算抛物线的参数,并在屏幕上绘制它。
类似地,我们可以编写一个函数来绘制双曲线:
```c++
void DrawHyperbola(HDC hdc, int x, int y, int width, int height) {
int a = width / 4;
int b = height / 4;
int cx = x + width / 2;
int cy = y + height / 2;
for (int i = -width / 2; i <= width / 2; i++) {
int j = cy + (b * sqrt(i * i / (double)a / a + 1));
SetPixel(hdc, cx + i, j, RGB(0, 0, 255));
j = cy - (b * sqrt(i * i / (double)a / a + 1));
SetPixel(hdc, cx + i, j, RGB(0, 0, 255));
}
}
```
现在我们需要实现放大缩小和移动功能。我们可以添加一些按钮和滑块来控制这些操作。以下是一个简单的代码片段,用于创建一个滑块和两个按钮:
```c++
HWND hSlider = CreateWindowEx(0, "msctls_trackbar32", "", WS_CHILD | WS_VISIBLE | TBS_HORZ, 10, 10, 200, 30, hwnd, (HMENU)IDC_SLIDER, NULL, NULL);
HWND hZoomInButton = CreateWindow("BUTTON", "Zoom In", WS_CHILD | WS_VISIBLE, 220, 10, 100, 30, hwnd, (HMENU)IDC_ZOOM_IN_BUTTON, NULL, NULL);
HWND hZoomOutButton = CreateWindow("BUTTON", "Zoom Out", WS_CHILD | WS_VISIBLE, 330, 10, 100, 30, hwnd, (HMENU)IDC_ZOOM_OUT_BUTTON, NULL, NULL);
```
我们还需要添加一些代码来处理这些控件的消息。以下是一个简单的函数,用于缩放图形:
```c++
void Scale(HWND hwnd, int scale) {
RECT rect;
GetClientRect(hwnd, &rect);
int width = (rect.right - rect.left) * scale / 100;
int height = (rect.bottom - rect.top) * scale / 100;
MoveWindow(hParabola, (rect.right - width) / 2, (rect.bottom - height) / 2, width, height, TRUE);
MoveWindow(hHyperbola, (rect.right - width) / 2, (rect.bottom - height) / 2, width, height, TRUE);
}
```
这个函数接受一个窗口句柄和一个缩放因子,并使用它来计算新的图形大小和位置。我们还需要添加一些代码来处理按钮和滑块的消息:
```c++
LRESULT CALLBACK WndProc(HWND hwnd, UINT message, WPARAM wParam, LPARAM lParam) {
switch (message) {
case WM_CREATE:
hParabola = CreateWindow("STATIC", NULL, WS_CHILD | WS_VISIBLE, 10, 50, 300, 300, hwnd, NULL, NULL, NULL);
hHyperbola = CreateWindow("STATIC", NULL, WS_CHILD | WS_VISIBLE, 320, 50, 300, 300, hwnd, NULL, NULL, NULL);
break;
case WM_SIZE:
Scale(hwnd, SendMessage(hSlider, TBM_GETPOS, 0, 0));
break;
case WM_HSCROLL:
if ((HWND)lParam == hSlider) {
Scale(hwnd, SendMessage(hSlider, TBM_GETPOS, 0, 0));
}
break;
case WM_COMMAND:
switch (LOWORD(wParam)) {
case IDC_ZOOM_IN_BUTTON:
SendMessage(hSlider, TBM_SETPOS, TRUE, SendMessage(hSlider, TBM_GETPOS, 0, 0) + 10);
break;
case IDC_ZOOM_OUT_BUTTON:
SendMessage(hSlider, TBM_SETPOS, TRUE, SendMessage(hSlider, TBM_GETPOS, 0, 0) - 10);
break;
}
break;
case WM_PAINT:
PAINTSTRUCT ps;
HDC hdc = BeginPaint(hwnd, &ps);
DrawParabola(GetDC(hParabola), 0, 0, 300, 300);
DrawHyperbola(GetDC(hHyperbola), 0, 0, 300, 300);
EndPaint(hwnd, &ps);
break;
case WM_DESTROY:
PostQuitMessage(0);
break;
default:
return DefWindowProc(hwnd, message, wParam, lParam);
}
return 0;
}
```
在这个示例中,我们使用CreateWindow函数创建两个静态控件来显示抛物线和双曲线。我们还在WM_SIZE消息中调用Scale函数来缩放图形。在WM_HSCROLL消息中,我们检查滑块控件是否发送了消息,并执行相应的缩放操作。在WM_COMMAND消息中,我们处理Zoom In和Zoom Out按钮的单击事件。在WM_PAINT消息中,我们调用DrawParabola和DrawHyperbola函数来绘制图形。
最后,我们需要在WinMain函数中创建滑块和按钮,并添加一些样式:
```c++
HWND hSlider = CreateWindowEx(0, "msctls_trackbar32", "", WS_CHILD | WS_VISIBLE | TBS_HORZ | TBS_AUTOTICKS, 10, 10, 200, 30, hwnd, (HMENU)IDC_SLIDER, NULL, NULL);
SendMessage(hSlider, TBM_SETRANGE, TRUE, MAKELPARAM(0, 200));
SendMessage(hSlider, TBM_SETPOS, TRUE, 100);
SendMessage(hSlider, TBM_SETTICFREQ, 10, 0);
HWND hZoomInButton = CreateWindow("BUTTON", "Zoom In", WS_CHILD | WS_VISIBLE | BS_PUSHBUTTON, 220, 10, 100, 30, hwnd, (HMENU)IDC_ZOOM_IN_BUTTON, NULL, NULL);
HWND hZoomOutButton = CreateWindow("BUTTON", "Zoom Out", WS_CHILD | WS_VISIBLE | BS_PUSHBUTTON, 330, 10, 100, 30, hwnd, (HMENU)IDC_ZOOM_OUT_BUTTON, NULL, NULL);
```
现在我们已经完成了一个基于API结构的Windows应用程序,它可以绘制抛物线和双曲线,并实现放大缩小和移动功能。以下是完整的代码示例:
相关推荐
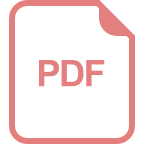














