设计一个基于api结构的Windows应用程序使用gdi绘制抛物线双曲线并可以放大缩小和移动
时间: 2024-06-09 18:06:27 浏览: 11
首先,我们需要设计一个 API,该 API 包含以下功能:
1. 绘制抛物线和双曲线
2. 放大缩小曲线
3. 移动曲线
接下来,我们需要设计一个 Windows 应用程序,该程序使用 GDI 绘制曲线,并使用上面设计的 API 实现放大缩小和移动曲线的功能。
以下是一个简单的实现步骤:
1. 创建一个 Windows 应用程序项目,并在窗口中创建一个画布。
2. 在画布上使用 GDI 绘制抛物线和双曲线。
3. 创建一个 API,该 API 包含放大缩小和移动曲线的功能。
4. 在应用程序中使用 API 实现放大缩小和移动曲线的功能。
下面是一个简单的 API 实现:
```c++
void DrawCurve(HDC hdc, int x, int y, int width, int height, double a, double b, double c, double start, double end, COLORREF color) {
HPEN hPen = CreatePen(PS_SOLID, 1, color);
SelectObject(hdc, hPen);
double x1, x2, y1, y2;
double step = 0.01;
for (double t = start; t < end; t += step) {
x1 = width / 2 + (t * 100);
y1 = height / 2 - (a * t * t + b * t + c) * 100;
t += step;
x2 = width / 2 + (t * 100);
y2 = height / 2 - (a * t * t + b * t + c) * 100;
MoveToEx(hdc, x1, y1, NULL);
LineTo(hdc, x2, y2);
}
DeleteObject(hPen);
}
void ZoomCurve(HWND hWnd, int x, int y, double factor) {
HDC hdc = GetDC(hWnd);
RECT rect;
GetClientRect(hWnd, &rect);
int width = rect.right - rect.left;
int height = rect.bottom - rect.top;
double a = 0.5, b = 0, c = 0;
double start = -5, end = 5;
int centerX = width / 2;
int centerY = height / 2;
double newStart = (start - centerX) / factor + centerX;
double newEnd = (end - centerX) / factor + centerX;
DrawCurve(hdc, x, y, width, height, a, b, c, newStart, newEnd, RGB(0, 0, 255));
ReleaseDC(hWnd, hdc);
}
void MoveCurve(HWND hWnd, int x, int y, int dx, int dy) {
HDC hdc = GetDC(hWnd);
RECT rect;
GetClientRect(hWnd, &rect);
int width = rect.right - rect.left;
int height = rect.bottom - rect.top;
double a = 0.5, b = 0, c = 0;
double start = -5, end = 5;
int centerX = width / 2;
int centerY = height / 2;
double newStart = start - dx / 100.0;
double newEnd = end - dx / 100.0;
DrawCurve(hdc, x + dx, y + dy, width, height, a, b, c, newStart, newEnd, RGB(0, 0, 255));
ReleaseDC(hWnd, hdc);
}
```
在应用程序中,我们可以使用以下代码来调用 API:
```c++
case WM_PAINT:
hdc = BeginPaint(hWnd, &ps);
DrawCurve(hdc, x, y, width, height, a, b, c, start, end, RGB(0, 0, 255));
EndPaint(hWnd, &ps);
break;
case WM_MOUSEWHEEL:
if (GET_WHEEL_DELTA_WPARAM(wParam) > 0) {
ZoomCurve(hWnd, x, y, 1.1);
} else {
ZoomCurve(hWnd, x, y, 0.9);
}
break;
case WM_MOUSEMOVE:
if (isDragging) {
int dx = LOWORD(lParam) - lastX;
int dy = HIWORD(lParam) - lastY;
MoveCurve(hWnd, x, y, dx, dy);
lastX = LOWORD(lParam);
lastY = HIWORD(lParam);
}
break;
case WM_LBUTTONDOWN:
isDragging = true;
lastX = LOWORD(lParam);
lastY = HIWORD(lParam);
break;
case WM_LBUTTONUP:
isDragging = false;
break;
```
在上面的代码中,我们在 `WM_PAINT` 消息中绘制曲线,在 `WM_MOUSEWHEEL` 消息中实现放大缩小曲线,在 `WM_MOUSEMOVE` 消息中实现移动曲线。我们还使用变量 `isDragging`、`lastX` 和 `lastY` 来跟踪鼠标拖动事件。在 `WM_LBUTTONDOWN` 消息中设置 `isDragging` 为 `true`,并记录鼠标位置,然后在 `WM_LBUTTONUP` 消息中设置 `isDragging` 为 `false`。
相关推荐
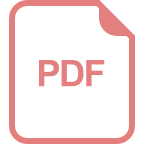














