Write a JavaScript function named reverse, which is used as an input parameter of the “sort" function, l.e., sori (reverse), to implement the following requirements: Input: A line of text, using prompt. Output: The words of the input text, in alphabetical reverse order. Note: you are only required to write the reverse function, rather than the entire html code
时间: 2023-11-27 16:53:11 浏览: 41
Here is the JavaScript function named `reverse` that can be used as an input parameter of the `sort` function:
```
function reverse(a, b) {
if (a < b) {
return 1;
} else if (a > b) {
return -1;
} else {
return 0;
}
}
```
To use this `reverse` function with the `sort` function to output the words of an input text in alphabetical reverse order, you can follow these steps:
1. Use the `prompt` function to get a line of text from the user and store it in a variable:
```
let inputText = prompt("Enter a line of text:");
```
2. Split the input text into an array of words using the `split` function:
```
let words = inputText.split(" ");
```
3. Sort the words in alphabetical order using the `sort` function and pass the `reverse` function as an input parameter:
```
words.sort(reverse);
```
4. Join the sorted words back into a string using the `join` function:
```
let outputText = words.join(" ");
```
5. Output the sorted words in alphabetical reverse order to the user:
```
alert(outputText);
```
Putting it all together, here's the full code:
```
function reverse(a, b) {
if (a < b) {
return 1;
} else if (a > b) {
return -1;
} else {
return 0;
}
}
let inputText = prompt("Enter a line of text:");
let words = inputText.split(" ");
words.sort(reverse);
let outputText = words.join(" ");
alert(outputText);
```
相关推荐
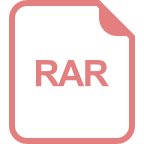














