用java Implement the function to reverse an array.
时间: 2024-03-09 16:48:55 浏览: 89
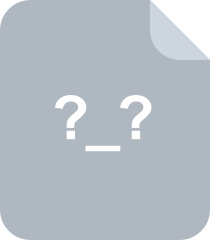
java实现反向输出整数
Sure, here's an example implementation of a function to reverse an array in Java:
```java
public static void reverseArray(int[] arr) {
int left = 0;
int right = arr.length - 1;
while (left < right) {
// Swap the elements at the left and right indices
int temp = arr[left];
arr[left] = arr[right];
arr[right] = temp;
// Move the left and right indices inwards
left++;
right--;
}
}
```
To use this function, you would pass in an array of integers like this:
```java
int[] arr = {1, 2, 3, 4, 5};
reverseArray(arr);
System.out.println(Arrays.toString(arr)); // Output: [5, 4, 3, 2, 1]
```
This function works by initializing two pointers, `left` and `right`, at opposite ends of the array. It then swaps the elements at these indices and moves the pointers inwards until they meet in the middle. This effectively reverses the order of the elements in the array.
阅读全文
相关推荐



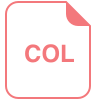
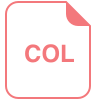
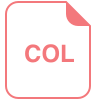
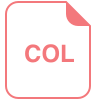
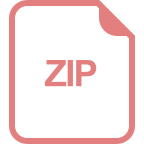
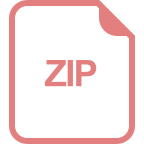
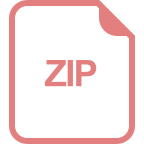
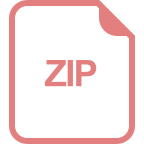