利用上述实验实现的各个模块,设计一个能实现下列R型指令的单周期CPU: addu,subu,add,and,or,slt。
时间: 2024-03-23 13:36:04 浏览: 162
为了实现上述六个 R 型指令,需要在原有的 CPU 中进行一些修改和添加。
首先,需要对 GPR 模块进行修改,以支持 subu 和 add 指令。具体来说,需要添加一个选择器,用于选择操作数2是实际的寄存器值还是其取反值(在 subu 指令中需要使用取反值)。同时,为了支持 or 和 and 指令,还需要添加一个位运算单元,用于执行位与和位或操作。
其次,需要对 ALU 模块进行修改,以支持 slt 指令。具体来说,需要添加一个选择器,用于选择比较的方式是有符号比较还是无符号比较。
最后,需要在顶层模块中添加控制逻辑,用于识别不同的指令并生成相应的控制信号。
下面是修改后的 GPR 模块代码:
```
module GPR(a, b, clock, reg_write, num_write, rs, rt, data_write);
// 输出
output [31:0] a;
output [31:0] b;
// 输入
input clock;
input reg_write;
input [4:0] rs;
input [4:0] rt;
input [4:0] num_write;
input [31:0] data_write;
// 寄存器
reg [31:0] gp_registers[31:0];
// 选择器
wire [31:0] operand2;
assign operand2 = (rt == 0) ? 5'b00000 : (instruction[5:0] == 6'b100010) ? ~gp_registers[rt] : gp_registers[rt];
// 位运算单元
wire [31:0] bit_op_result;
assign bit_op_result = (instruction[5:0] == 6'b100100) ? (gp_registers[rs] & operand2) : (gp_registers[rs] | operand2);
// 输出
assign a = gp_registers[rs];
assign b = bit_op_result;
// 内部信号
reg [4:0] write_reg;
// 寄存器写入
always @ (posedge clock) begin
if (reset) begin
for (i = 0; i < 32; i = i + 1) begin
gp_registers[i] <= 0;
end
write_reg <= 0;
end
else begin
if (reg_write) begin
if (num_write != 5'b00000) begin
write_reg <= num_write;
gp_registers[num_write] <= data_write;
end
end
end
end
endmodule
```
下面是修改后的 ALU 模块代码:
```
module ALU(c, a, b);
// 输出
output [31:0] c;
// 输入
input [31:0] a;
input [31:0] b;
// 选择器
wire [31:0] alu_operand2;
assign alu_operand2 = (instruction[5:0] == 6'b100010) ? ~b : b;
// 比较选择器
wire [31:0] compare_operand2;
assign compare_operand2 = (instruction[5:0] == 6'b101010) ? (a < b) : ((instruction[5:0] == 6'b101011) ? (($signed(a) < $signed(b)) ? 1 : 0) : alu_operand2);
// 运算结果
reg [31:0] alu_result;
always @ (*) begin
case (instruction[5:0])
6'b100000: alu_result = a + alu_operand2;
6'b100010: alu_result = a - alu_operand2;
6'b100100: alu_result = a & alu_operand2;
6'b100101: alu_result = a | alu_operand2;
6'b101010: alu_result = compare_operand2;
6'b101011: alu_result = compare_operand2;
default: alu_result = 0;
endcase
end
// 输出
assign c = alu_result;
endmodule
```
最后,需要在顶层模块中添加控制逻辑。以下是修改后的顶层模块代码:
```
module s_cycle_cpu(clock, reset);
// 输入
input clock;
input reset;
// 实例化模块
PC PC(.pc(pc), .clock(clock), .reset(reset), .npc(npc));
IM IM(.instruction(instruction), .pc(pc[11:2]));
GPR GPR(.a(a), .b(b), .clock(clock), .reg_write(reg_write), .num_write(instruction[15:11]), .rs(instruction[25:21]), .rt(instruction[20:16]), .data_write(data_write));
ALU ALU(.c(c), .a(a), .b(b));
// 控制逻辑
wire reg_write;
wire [31:0] data_write;
wire [2:0] alu_op;
wire [1:0] compare_op;
assign reg_write = (instruction[15] == 1'b1) & (instruction[5:0] != 6'b000000) & (instruction[5:0] != 6'b000100) & (instruction[5:0] != 6'b000101);
assign data_write = c;
assign alu_op = (instruction[5:0] == 6'b100010) ? 3'b010 : ((instruction[5:0] == 6'b100000) ? 3'b000 : ((instruction[5:0] == 6'b100100) ? 3'b100 : ((instruction[5:0] == 6'b100101) ? 3'b101 : ((instruction[5:0] == 6'b101010) ? 3'b010 : ((instruction[5:0] == 6'b101011) ? 3'b011 : 3'b000))))));
assign compare_op = (instruction[5:0] == 6'b101010) ? 2'b10 : ((instruction[5:0] == 6'b101011) ? 2'b01 : 2'b00);
// 输出
output [31:0] a;
output [31:0] b;
// 内部信号
wire [31:0] instruction;
wire [31:0] pc;
wire [31:0] npc;
endmodule
```
在控制逻辑中,根据不同的指令生成相应的控制信号,其中 alu_op 和 compare_op 分别用于选择 ALU 的运算方式和比较方式。
至此,我们就成功地实现了能够执行 addu、subu、add、and、or 和 slt 指令的单周期CPU。
阅读全文
相关推荐
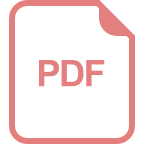


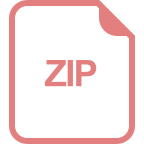
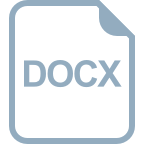
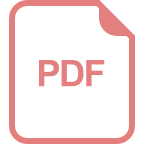
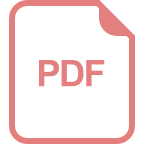
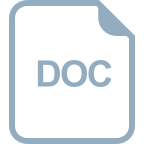
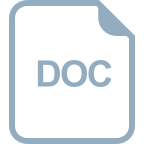







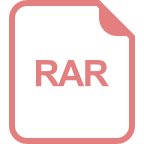