区域生长c++代码
时间: 2023-07-09 11:18:14 浏览: 46
以下是一个简单的基于C++的区域生长算法实现:
```c++
#include <iostream>
#include <vector>
#include <queue>
#include <opencv2/opencv.hpp>
using namespace std;
using namespace cv;
// 区域生长算法
void regionGrowing(const Mat& src, Mat& dst, Point seed, int threshold) {
int rows = src.rows;
int cols = src.cols;
// 创建一个标记图像,用于记录每个像素是否已经被访问
Mat flag = Mat::zeros(rows, cols, CV_8U);
// 创建一个队列,用于存储需要进行生长的像素点
queue<Point> q;
// 将种子点加入队列
q.push(seed);
// 记录生长区域的像素坐标
vector<Point> region;
while (!q.empty()) {
// 取出队列中的像素点
Point p = q.front();
q.pop();
// 如果该点已经被访问,则跳过
if (flag.at<uchar>(p.y, p.x) != 0) {
continue;
}
// 计算该点与种子点之间的距离
int dist = abs(src.at<uchar>(p.y, p.x) - src.at<uchar>(seed.y, seed.x));
// 如果该点与种子点之间的距离小于阈值,则加入生长区域
if (dist <= threshold) {
region.push_back(p);
flag.at<uchar>(p.y, p.x) = 255;
// 将该点的8邻域加入队列
if (p.x > 0) {
q.push(Point(p.x - 1, p.y));
}
if (p.x < cols - 1) {
q.push(Point(p.x + 1, p.y));
}
if (p.y > 0) {
q.push(Point(p.x, p.y - 1));
}
if (p.y < rows - 1) {
q.push(Point(p.x, p.y + 1));
}
if (p.x > 0 && p.y > 0) {
q.push(Point(p.x - 1, p.y - 1));
}
if (p.x > 0 && p.y < rows - 1) {
q.push(Point(p.x - 1, p.y + 1));
}
if (p.x < cols - 1 && p.y > 0) {
q.push(Point(p.x + 1, p.y - 1));
}
if (p.x < cols - 1 && p.y < rows - 1) {
q.push(Point(p.x + 1, p.y + 1));
}
}
}
// 将生长区域中的像素点赋值给目标图像
dst = Mat::zeros(rows, cols, CV_8U);
for (int i = 0; i < region.size(); i++) {
dst.at<uchar>(region[i].y, region[i].x) = 255;
}
}
int main() {
// 读入图像
Mat src = imread("test.jpg", IMREAD_GRAYSCALE);
// 显示原始图像
imshow("src", src);
// 进行区域生长
Mat dst;
Point seed(100, 100);
int threshold = 10;
regionGrowing(src, dst, seed, threshold);
// 显示生长结果
imshow("dst", dst);
waitKey(0);
return 0;
}
```
其中,`src`表示原始图像,`dst`表示目标图像,`seed`表示种子点的坐标,`threshold`表示阈值。在实现过程中,使用了一个标记图像`flag`来记录每个像素是否已经被访问,使用一个队列`q`来存储需要进行生长的像素点。算法的核心部分是在循环中不断取出队列中的像素点,计算其与种子点之间的距离,如果小于阈值则将其加入生长区域,并将其8邻域中未被访问的像素点加入队列中,直到队列为空为止。最后将生长区域中的像素点赋值给目标图像即可。
相关推荐
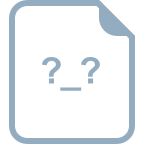














