def LSTNetAttention(trainX1,trainX2,trainY,config): # 输入数据 #inputs = tf.keras.layers.Input(shape=(seq_len, input_dim)) input1 = Input(shape=(trainX1.shape[1], trainX1.shape[2],)) # 定义attention权重 attention_weights1 = TimeDistributed(Dense(1))(input1) attention_weights1 = Activation('softmax')(attention_weights1) # 将attention权重应用于输入数据 context_vector1 = Dot(axes=(2, 1))([attention_weights1, input1]) # 应用注意力机制到第二个输入 # Input2: long-term time series with period input2 = Input(shape=(trainX2.shape[1], trainX2.shape[2],)) # 定义attention权重 attention_weights2 = TimeDistributed(Dense(1))(input2) attention_weights2 = Activation('softmax')(attention_weights2) # 将attention权重应用于输入数据 context_vector2 = Dot(axes=(2, 1))([attention_weights2, input2]) merged_output = concatenate([context_vector1,context_vector2]) lstm_out = LSTM(64, return_sequences=False)(merged_output) # 加入LSTM层 lstm_out = Dense(32, activation='relu')(lstm_out) res = Dense(trainY.shape[1])(lstm_out) 有没有错误
时间: 2023-11-29 15:03:44 浏览: 76
代码看起来没有明显的错误,但是需要注意以下几点:
1. 在定义输入时,应该使用 `from tensorflow.keras.layers import Input` 引入 `Input`。
2. 对于 `TimeDistributed(Dense(1))`,可以考虑加入 `kernel_regularizer` 和 `bias_regularizer` 参数进行正则化,以防止过拟合。
3. 在使用 `concatenate` 合并两个输入时,可以加入 `axis` 参数明确指定合并的维度。
4. 在最后一层输出时,可以使用 `softmax` 激活函数,以确保输出的概率分布符合要求。
相关问题
def LSTNetAttention(trainX1,trainX2,trainY,config): # 输入数据 #inputs = tf.keras.layers.Input(shape=(seq_len, input_dim)) time_steps1 = trainX1.shape[1] input_dim1 = trainX1.shape[2] ....... concat = concatenate([lstm_out1,lstm_out2]) res = Activation('sigmoid')(concat) model = Model(inputs=[input_data1,input_data2], outputs=res) return model 请将GRACH部分加入我的模型中
好的,你可以将以下代码添加到你的 `LSTNetAttention` 函数中,以将 GARCH 模型的预测结果作为一个新的特征加入到时间序列预测模型中:
```
# 使用 GARCH 模型预测波动率
am = arch.arch_model(trainY, vol='GARCH', p=1, q=1)
res = am.fit()
volatility = res.forecast(horizon=1).variance.iloc[-1].values
# 将预测结果和波动率相乘,得到最终的预测结果
pred = model.predict([trainX1, trainX2])
pred = pred * np.sqrt(volatility)
# 将预测结果作为一个新的特征加入到模型中
new_feature = pred.reshape(-1, 1) # 重塑成 (batch_size, 1) 的形状
concat = concatenate([lstm_out1, lstm_out2, new_feature])
res = Activation('sigmoid')(concat)
model = Model(inputs=[input_data1, input_data2], outputs=res)
```
需要注意以下几点:
1. 在使用 GARCH 模型预测波动率时,你需要将 `trainY` 作为输入参数传递给 `arch_model` 函数。
2. 在将预测结果作为一个新的特征加入到模型中时,你需要将它重塑成 (batch_size, 1) 的形状,并将它与 LSTM 模型的输出进行拼接。
3. 在训练模型时,你需要将新的特征加入到输入数据中,并将它们一起传递给 `fit` 方法。
class BiCLSTMCell(tf.keras.layers.Layer): def __init__(self, units, **kwargs): self.units = units self.state_size = [units, units] super(BiCLSTMCell, self).__init__(**kwargs) def build(self, input_shape): self.kernel = self.add_weight(shape=(input_shape[-1], self.units * 4), initializer='glorot_uniform', name='kernel') self.recurrent_kernel = self.add_weight(shape=(self.units, self.units * 4), initializer='orthogonal', name='recurrent_kernel') self.bias = self.add_weight(shape=(self.units * 4,), initializer='zeros', name='bias') self.ca_dense1 = Dense(self.units, activation='relu') self.ca_dense2 = Dense(self.units, activation='sigmoid') super(BiCLSTMCell, self).build(input_shape) def call(self, inputs, states): h_tm1, c_tm1 = states z = tf.keras.backend.dot(inputs, self.kernel) z += tf.keras.backend.dot(h_tm1, self.recurrent_kernel) z = tf.keras.backend.bias_add(z, self.bias) z0, z1, z2, z3 = tf.split(z, 4, axis=-1) # Channel Attention Mechanism ca = self.ca_dense1(c_tm1) ca = self.ca_dense2(ca) # LSTM Gates input_gate = tf.keras.activations.sigmoid(z0) forget_gate = tf.keras.activations.sigmoid(z1) * ca output_gate = tf.keras.activations.sigmoid(z2) cell_state = tf.keras.activations.tanh(z3) c = forget_gate * c_tm1 + input_gate * cell_state h = output_gate * tf.keras.activations.tanh(c) return h, [h, c] def get_config(self): config = super(BiCLSTMCell, self).get_config() config.update({'units': self.units}) return config
这是一个自定义的双向LSTM单元类,继承了Keras的Layer类。在初始化方法中,定义了该单元的神经元个数和状态大小。在build方法中,定义了该单元的权重矩阵和偏置向量,并定义了两个全连接层,用于通道注意力机制。在call方法中,实现了双向LSTM单元的计算过程,包括输入和遗忘门的计算、细胞状态的更新、输出门的计算和输出的计算。同时,还加入了通道注意力机制来增强模型的表达能力。最后,在get_config方法中,返回了该单元的参数配置信息。
阅读全文
相关推荐


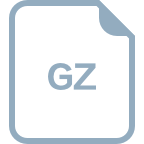













