遗传算法求函数最大值代码
时间: 2023-08-30 13:08:15 浏览: 55
以下是一个使用遗传算法求解函数最大值的示例代码(使用Python语言):
```python
import random
# 定义目标函数
def f(x):
return x**2
# 遗传算法参数设置
POPULATION_SIZE = 100 # 种群大小
CHROMOSOME_LENGTH = 8 # 染色体长度(二进制编码)
CROSSOVER_RATE = 0.8 # 交叉概率
MUTATION_RATE = 0.01 # 变异概率
GENERATIONS = 50 # 迭代次数
# 初始化种群
def create_population():
population = []
for _ in range(POPULATION_SIZE):
chromosome = ''.join(random.choices(['0', '1'], k=CHROMOSOME_LENGTH))
population.append(chromosome)
return population
# 计算染色体对应的函数值
def calculate_fitness(chromosome):
x = int(chromosome, 2)
return f(x)
# 选择操作(轮盘赌选择)
def selection(population):
fitness_values = [calculate_fitness(chromosome) for chromosome in population]
total_fitness = sum(fitness_values)
probabilities = [fitness / total_fitness for fitness in fitness_values]
selected_indices = random.choices(range(POPULATION_SIZE), probabilities, k=POPULATION_SIZE)
selected_population = [population[i] for i in selected_indices]
return selected_population
# 交叉操作(单点交叉)
def crossover(parent1, parent2):
if random.random() < CROSSOVER_RATE:
crossover_point = random.randint(1, CHROMOSOME_LENGTH - 1)
child1 = parent1[:crossover_point] + parent2[crossover_point:]
child2 = parent2[:crossover_point] + parent1[crossover_point:]
return child1, child2
else:
return parent1, parent2
# 变异操作(单点变异)
def mutation(chromosome):
mutated_chromosome = list(chromosome)
for i in range(CHROMOSOME_LENGTH):
if random.random() < MUTATION_RATE:
mutated_chromosome[i] = '0' if chromosome[i] == '1' else '1'
return ''.join(mutated_chromosome)
# 遗传算法主过程
def genetic_algorithm():
population = create_population()
for _ in range(GENERATIONS):
new_population = []
# 选择
selected_population = selection(population)
# 交叉和变异
for i in range(0, POPULATION_SIZE, 2):
parent1 = selected_population[i]
parent2 = selected_population[i + 1]
child1, child2 = crossover(parent1, parent2)
child1 = mutation(child1)
child2 = mutation(child2)
new_population.extend([child1, child2])
population = new_population
# 寻找最优解
best_chromosome = max(population, key=lambda chromosome: calculate_fitness(chromosome))
best_x = int(best_chromosome, 2)
best_fitness = f(best_x)
return best_x, best_fitness
# 运行遗传算法
best_x, best_fitness = genetic_algorithm()
print("最优解 x =", best_x)
print("最大值 f(x) =", best_fitness)
```
上述代码中使用了简单的二进制编码表示染色体,轮盘赌选择、单点交叉和单点变异作为遗传算法的基本操作。通过迭代多次,最终找到函数的最大值。你可以根据自己的需求进行参数调整和算法改进。
相关推荐
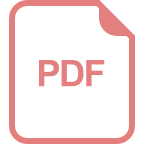
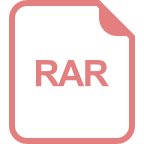














