生命游戏使用C++类和对象以及easyx图形库实现
时间: 2023-12-13 10:05:40 浏览: 33
生命游戏(Conway's Game of Life)是一种细胞自动机,它由英国数学家约翰·康威于1970年发明。玩家可以通过简单的规则控制细胞的生命周期,从而观察细胞的演化。下面是使用C++类和对象以及easyx图形库实现生命游戏的示例代码:
首先,我们需要创建一个细胞类Cell,它包含细胞的状态(存活或死亡)以及其在屏幕上的位置。
```c++
class Cell {
public:
Cell(int x, int y, int size) : alive(false), x(x), y(y), size(size) {}
void draw() {
if (alive) {
setfillcolor(RGB(255, 255, 255));
} else {
setfillcolor(RGB(0, 0, 0));
}
fillrectangle(x, y, x + size, y + size);
}
bool alive;
int x, y, size;
};
```
接下来,我们需要创建一个游戏类Game,它包含游戏的状态(进行中或结束)以及细胞的二维数组。
```c++
class Game {
public:
Game(int width, int height, int size) : width(width), height(height), size(size), running(false), cells(nullptr) {
init();
}
~Game() {
for (int i = 0; i < height; i++) {
delete[] cells[i];
}
delete[] cells;
}
void start() {
running = true;
while (running) {
update();
draw();
Sleep(100);
}
}
private:
void init() {
cells = new Cell*[height];
for (int i = 0; i < height; i++) {
cells[i] = new Cell[width];
for (int j = 0; j < width; j++) {
cells[i][j] = Cell(j * size, i * size, size);
}
}
}
void update() {
// 更新每个细胞的状态
}
void draw() {
for (int i = 0; i < height; i++) {
for (int j = 0; j < width; j++) {
cells[i][j].draw();
}
}
}
int width, height, size;
bool running;
Cell** cells;
};
```
在Game类的update()函数中,我们可以根据生命游戏的规则更新每个细胞的状态。具体实现可以参考以下代码:
```c++
void update() {
Cell** newCells = new Cell*[height];
for (int i = 0; i < height; i++) {
newCells[i] = new Cell[width];
for (int j = 0; j < width; j++) {
int count = 0;
for (int k = -1; k <= 1; k++) {
for (int l = -1; l <= 1; l++) {
if (k == 0 && l == 0) {
continue;
}
int x = (j + l + width) % width;
int y = (i + k + height) % height;
if (cells[y][x].alive) {
count++;
}
}
}
if (cells[i][j].alive) {
if (count == 2 || count == 3) {
newCells[i][j].alive = true;
} else {
newCells[i][j].alive = false;
}
} else {
if (count == 3) {
newCells[i][j].alive = true;
} else {
newCells[i][j].alive = false;
}
}
newCells[i][j].x = cells[i][j].x;
newCells[i][j].y = cells[i][j].y;
newCells[i][j].size = cells[i][j].size;
}
}
for (int i = 0; i < height; i++) {
delete[] cells[i];
}
delete[] cells;
cells = newCells;
}
```
最后,在主函数中创建游戏对象并启动游戏。
```c++
int main() {
int width = 80;
int height = 60;
int size = 10;
initgraph(width * size, height * size);
Game game(width, height, size);
game.start();
closegraph();
return 0;
}
```
以上是使用C++类和对象以及easyx图形库实现生命游戏的示例代码。
相关推荐
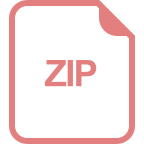
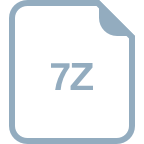













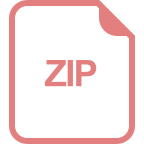
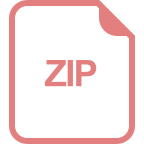