如何在迭代过程中加锁:Iterator<Map.Entry<String, Long>>
时间: 2023-11-11 07:29:05 浏览: 147
在迭代过程中加锁可以使用synchronized关键字来实现。具体来说,可以将需要保护的代码块用synchronized关键字包裹起来,这样就能够确保在任意时刻只有一个线程能够访问该代码块,避免了并发访问的问题。对于Iterator<Map.Entry<String, Long>>迭代器来说,可以通过以下方式来实现加锁:
```
Map<String, Long> map = new HashMap<>();
synchronized (map) {
Iterator<Map.Entry<String, Long>> iterator = map.entrySet().iterator();
while (iterator.hasNext()) {
Map.Entry<String, Long> entry = iterator.next();
synchronized (entry) {
// 在这里对entry进行操作
}
}
}
```
在上述代码中,使用synchronized关键字对map进行加锁,避免了并发访问的问题。同时,在迭代器的每个元素entry上也加了锁,确保每个元素的访问是线程安全的。需要注意的是,加锁的粒度应该尽可能细,避免锁的竞争过于激烈,导致程序性能下降。
相关问题
如何在迭代过程中加锁:Iterator<Map.Entry<String, Long>> entries = concurrentHashMap.entrySet().iterator();
在迭代过程中加锁可以使用synchronized关键字或者ConcurrentHashMap自带的锁机制来实现。对于Iterator<Map.Entry<String, Long>> entries = concurrentHashMap.entrySet().iterator()迭代器来说,可以通过以下两种方式来实现加锁:
1. 使用synchronized关键字
```
synchronized (concurrentHashMap) {
Iterator<Map.Entry<String, Long>> entries = concurrentHashMap.entrySet().iterator();
while (entries.hasNext()) {
Map.Entry<String, Long> entry = entries.next();
synchronized (entry) {
// 在这里对entry进行操作
}
}
}
```
在上述代码中,使用synchronized关键字对concurrentHashMap进行加锁,确保在任意时刻只有一个线程能够访问concurrentHashMap,避免了并发访问的问题。同时,在迭代器的每个元素entry上也加了锁,确保每个元素的访问是线程安全的。
2. 使用ConcurrentHashMap自带的锁机制
```
ConcurrentHashMap<String, Long> concurrentHashMap = new ConcurrentHashMap<>();
Iterator<Map.Entry<String, Long>> entries = concurrentHashMap.entrySet().iterator();
while (entries.hasNext()) {
Map.Entry<String, Long> entry = entries.next();
concurrentHashMap.lock(entry);
try {
// 在这里对entry进行操作
} finally {
concurrentHashMap.unlock(entry);
}
}
```
在上述代码中,使用ConcurrentHashMap自带的锁机制来加锁,调用lock方法对entry进行加锁,使用try-finally语句块确保在任何情况下都能够正确释放锁。需要注意的是,ConcurrentHashMap的锁机制是非公平的,可能会导致一些线程长时间等待锁的释放,影响程序的性能。因此,如果需要公平锁,请使用java.util.concurrent包中的公平锁。
Iterator<Map.Entry<String, Long>>下有一个remove方法,作用是什么
remove方法的作用是将迭代器当前指向的元素从迭代器所在的集合中移除。具体来说,如果在迭代器调用remove方法之前,调用了一次next方法,返回了一个Map.Entry<String, Long>对象,那么调用remove方法就会将这个对象对应的键值对从Map中移除。需要注意的是,remove方法只能在调用了一次next方法之后才能被调用,否则会抛出IllegalStateException异常。此外,如果在迭代器调用remove方法之前,集合中的元素已经被移除了,那么调用remove方法也会抛出IllegalStateException异常。
阅读全文
相关推荐
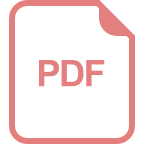
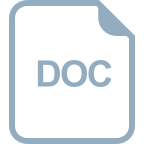
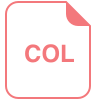
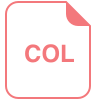
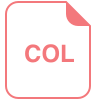
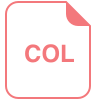
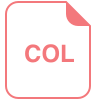
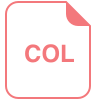
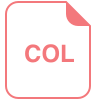
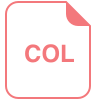
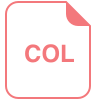
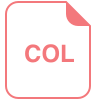
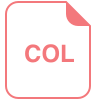
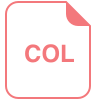