用c语言写一个求二叉树深度的算法
时间: 2023-05-17 22:07:57 浏览: 147
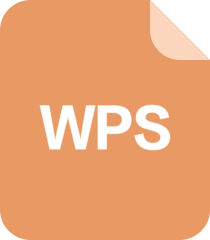
用c语言实现二叉树的深度,宽度,相似度的编程

以下是用 C 语言编写求二叉树深度的算法:
```c
#include <stdio.h>
#include <stdlib.h>
struct TreeNode {
int val;
struct TreeNode *left;
struct TreeNode *right;
};
int maxDepth(struct TreeNode* root) {
if (root == NULL) {
return 0;
}
int leftDepth = maxDepth(root->left);
int rightDepth = maxDepth(root->right);
return leftDepth > rightDepth ? leftDepth + 1 : rightDepth + 1;
}
int main() {
// 构造一棵二叉树
struct TreeNode* root = (struct TreeNode*)malloc(sizeof(struct TreeNode));
root->val = 1;
root->left = (struct TreeNode*)malloc(sizeof(struct TreeNode));
root->left->val = 2;
root->left->left = NULL;
root->left->right = NULL;
root->right = (struct TreeNode*)malloc(sizeof(struct TreeNode));
root->right->val = 3;
root->right->left = (struct TreeNode*)malloc(sizeof(struct TreeNode));
root->right->left->val = 4;
root->right->left->left = NULL;
root->right->left->right = NULL;
root->right->right = NULL;
// 计算二叉树深度
int depth = maxDepth(root);
printf("Depth of the binary tree is %d\n", depth);
// 释放内存
free(root->left);
free(root->right->left);
free(root->right);
free(root);
return 0;
}
```
这个算法的时间复杂度是 O(n),其中 n 是二叉树中节点的个数。
阅读全文
相关推荐
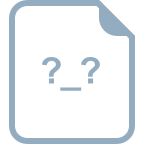
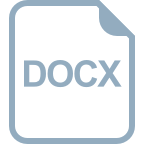
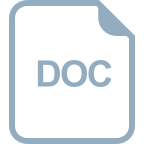




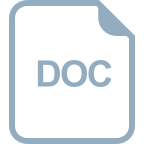
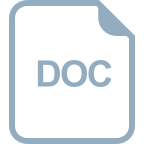
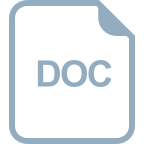
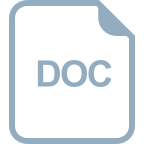
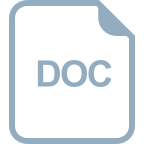
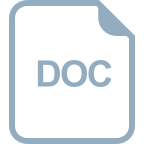


