java开心消消乐代码编写
时间: 2023-07-02 15:07:23 浏览: 172
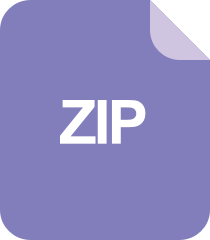
html开心消消乐源码

以下是一个简单的 Java 开心消消乐游戏代码示例:
```java
import java.util.*;
public class HappyEliminationGame {
private static int ROWS = 10;
private static int COLS = 10;
private static int COLORS = 5;
private static int MINIMUM_ELIMINATION = 3;
private int[][] board;
public HappyEliminationGame() {
board = new int[ROWS][COLS];
initializeBoard();
}
private void initializeBoard() {
Random random = new Random();
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
board[i][j] = random.nextInt(COLORS) + 1;
}
}
}
public void play() {
Scanner scanner = new Scanner(System.in);
while (true) {
printBoard();
System.out.print("Enter row and column to select: ");
int row = scanner.nextInt();
int col = scanner.nextInt();
if (!isValidMove(row, col)) {
System.out.println("Invalid move, please try again.");
continue;
}
int color = board[row][col];
int numEliminated = eliminateConnectedColors(row, col, color);
if (numEliminated < MINIMUM_ELIMINATION) {
System.out.println("Not enough colors connected, please try again.");
board[row][col] = color;
continue;
}
shiftDown();
fillEmptySpaces();
if (isGameOver()) {
System.out.println("Game over!");
break;
}
}
scanner.close();
}
private void printBoard() {
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
System.out.print(board[i][j] + " ");
}
System.out.println();
}
}
private boolean isValidMove(int row, int col) {
return row >= 0 && row < ROWS && col >= 0 && col < COLS;
}
private int eliminateConnectedColors(int row, int col, int color) {
int numEliminated = 0;
if (board[row][col] != color) {
return numEliminated;
}
board[row][col] = 0;
numEliminated++;
if (row > 0) {
numEliminated += eliminateConnectedColors(row - 1, col, color);
}
if (row < ROWS - 1) {
numEliminated += eliminateConnectedColors(row + 1, col, color);
}
if (col > 0) {
numEliminated += eliminateConnectedColors(row, col - 1, color);
}
if (col < COLS - 1) {
numEliminated += eliminateConnectedColors(row, col + 1, color);
}
return numEliminated;
}
private void shiftDown() {
for (int j = 0; j < COLS; j++) {
int bottom = ROWS - 1;
for (int i = ROWS - 1; i >= 0; i--) {
if (board[i][j] == 0) {
continue;
}
board[bottom][j] = board[i][j];
if (bottom != i) {
board[i][j] = 0;
}
bottom--;
}
}
}
private void fillEmptySpaces() {
Random random = new Random();
for (int j = 0; j < COLS; j++) {
for (int i = ROWS - 1; i >= 0; i--) {
if (board[i][j] != 0) {
continue;
}
board[i][j] = random.nextInt(COLORS) + 1;
}
}
}
private boolean isGameOver() {
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
int color = board[i][j];
if (color == 0) {
continue;
}
if (eliminateConnectedColors(i, j, color) >= MINIMUM_ELIMINATION) {
return false;
}
}
}
return true;
}
public static void main(String[] args) {
HappyEliminationGame game = new HappyEliminationGame();
game.play();
}
}
```
这个示例游戏具有以下特点:
- 游戏板由 10 行 10 列的方块组成,每个方块有 5 种不同的颜色之一。
- 要玩游戏,玩家需要输入行和列以选择一个方块。
- 玩家所选方块的颜色与相邻相同颜色的方块将被消除。
- 消除后,上方的方块将下移填充空缺,并将新的随机颜色方块填充到顶部。
- 当不能再消除任何方块时,游戏结束。
当然,这只是一个简单的示例,您可以根据自己的需求进行修改或改进。
阅读全文
相关推荐
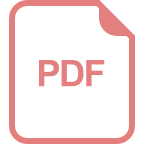
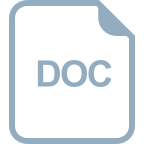





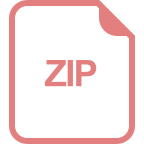
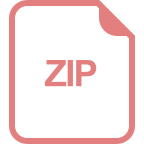
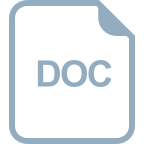

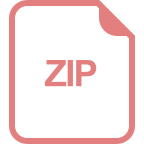
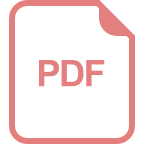
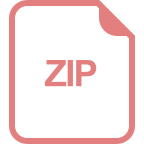