3. 定义一个学生类, 包含学生的学号、 姓名和年龄等基本信息, 将学生信息写入二进 制文件 student. dat 中, 实现对学生信息的显示、 查询和删除等基本功能。
时间: 2024-02-13 16:00:52 浏览: 28
好的,这是一个比较具体的编程问题。下面给出一个简单的 C++ 代码实现:
```cpp
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
// 学生类
class Student {
public:
Student(int id, const string& name, int age) :
m_id(id), m_name(name), m_age(age) {}
int getId() const { return m_id; }
const string& getName() const { return m_name; }
int getAge() const { return m_age; }
private:
int m_id;
string m_name;
int m_age;
};
// 学生管理类
class StudentManager {
public:
// 添加学生信息到文件
void addStudent(const Student& student) {
ofstream ofs("student.dat", ios::binary | ios::app);
ofs.write(reinterpret_cast<const char*>(&student), sizeof(Student));
ofs.close();
}
// 显示学生信息
void showStudents() {
ifstream ifs("student.dat", ios::binary);
if (!ifs) {
cout << "Failed to open file!" << endl;
return;
}
Student student(0, "", 0);
while (ifs.read(reinterpret_cast<char*>(&student), sizeof(Student))) {
cout << "ID: " << student.getId() << ", Name: " << student.getName() << ", Age: " << student.getAge() << endl;
}
ifs.close();
}
// 查询学生信息
void queryStudent(int id) {
ifstream ifs("student.dat", ios::binary);
if (!ifs) {
cout << "Failed to open file!" << endl;
return;
}
Student student(0, "", 0);
bool found = false;
while (ifs.read(reinterpret_cast<char*>(&student), sizeof(Student))) {
if (student.getId() == id) {
cout << "ID: " << student.getId() << ", Name: " << student.getName() << ", Age: " << student.getAge() << endl;
found = true;
break;
}
}
if (!found) {
cout << "Student not found!" << endl;
}
ifs.close();
}
// 删除学生信息
void deleteStudent(int id) {
ifstream ifs("student.dat", ios::binary);
if (!ifs) {
cout << "Failed to open file!" << endl;
return;
}
ofstream ofs("temp.dat", ios::binary);
Student student(0, "", 0);
bool found = false;
while (ifs.read(reinterpret_cast<char*>(&student), sizeof(Student))) {
if (student.getId() != id) {
ofs.write(reinterpret_cast<const char*>(&student), sizeof(Student));
} else {
found = true;
}
}
ifs.close();
ofs.close();
if (found) {
remove("student.dat");
rename("temp.dat", "student.dat");
cout << "Student deleted!" << endl;
} else {
remove("temp.dat");
cout << "Student not found!" << endl;
}
}
};
int main() {
StudentManager sm;
sm.addStudent(Student(1, "Tom", 18));
sm.addStudent(Student(2, "Jerry", 20));
sm.showStudents();
sm.queryStudent(2);
sm.deleteStudent(1);
sm.showStudents();
return 0;
}
```
上述代码中,`Student` 类表示一个学生对象,包含学生的学号、姓名和年龄等基本信息。`StudentManager` 类表示学生管理器,其中的 `addStudent` 方法可以将一个学生对象写入二进制文件中,`showStudents` 方法可以显示所有学生信息,`queryStudent` 方法可以根据学号查询学生信息,`deleteStudent` 方法可以根据学号删除学生信息。在 `main` 函数中,我们创建一个 `StudentManager` 对象,添加两个学生信息,然后显示所有学生信息,查询学号为 2 的学生信息,最后删除学号为 1 的学生信息。
相关推荐
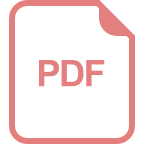
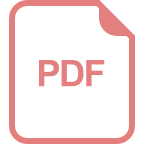
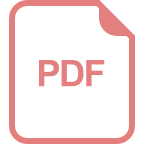














