Javaswing 实现开心消消乐,代码
时间: 2023-12-14 07:40:11 浏览: 131
以下是一个简单的 Java Swing 开心消消乐游戏的代码示例。
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class HappyElimination extends JFrame implements ActionListener {
private static final long serialVersionUID = 1L;
private static final int ROWS = 8;
private static final int COLS = 8;
private static final int BLOCK_SIZE = 50;
private static final int GAP_SIZE = 5;
private static final int BOARD_WIDTH = COLS * (BLOCK_SIZE + GAP_SIZE) + GAP_SIZE;
private static final int BOARD_HEIGHT = ROWS * (BLOCK_SIZE + GAP_SIZE) + GAP_SIZE;
private static final int MIN_BLOCKS_TO_MATCH = 3;
private static final Color[] COLORS = { Color.RED, Color.GREEN, Color.BLUE };
private JButton[][] blocks;
private JButton newGameButton;
private JLabel scoreLabel;
private int[][] board;
private int score;
private boolean isDragging;
private int dragStartRow, dragStartCol, dragEndRow, dragEndCol;
public HappyElimination() {
super("Happy Elimination");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(BOARD_WIDTH, BOARD_HEIGHT + 50);
setResizable(false);
setLocationRelativeTo(null);
JPanel boardPanel = new JPanel();
boardPanel.setLayout(new GridLayout(ROWS, COLS, GAP_SIZE, GAP_SIZE));
boardPanel.setPreferredSize(new Dimension(BOARD_WIDTH, BOARD_HEIGHT));
blocks = new JButton[ROWS][COLS];
for (int row = 0; row < ROWS; row++) {
for (int col = 0; col < COLS; col++) {
JButton block = new JButton();
block.setPreferredSize(new Dimension(BLOCK_SIZE, BLOCK_SIZE));
block.setFocusPainted(false);
block.setBorderPainted(false);
block.addActionListener(this);
block.addMouseMotionListener(new MouseMotionAdapter() {
public void mouseDragged(MouseEvent e) {
if (!isDragging) {
isDragging = true;
dragStartRow = row;
dragStartCol = col;
}
dragEndRow = (e.getY() - block.getY()) / BLOCK_SIZE;
dragEndCol = (e.getX() - block.getX()) / BLOCK_SIZE;
if (dragEndRow < 0) {
dragEndRow = 0;
} else if (dragEndRow >= ROWS) {
dragEndRow = ROWS - 1;
}
if (dragEndCol < 0) {
dragEndCol = 0;
} else if (dragEndCol >= COLS) {
dragEndCol = COLS - 1;
}
if (dragStartRow != dragEndRow || dragStartCol != dragEndCol) {
JButton startBlock = blocks[dragStartRow][dragStartCol];
JButton endBlock = blocks[dragEndRow][dragEndCol];
swapBlocks(startBlock, endBlock);
dragStartRow = dragEndRow;
dragStartCol = dragEndCol;
}
}
});
blocks[row][col] = block;
boardPanel.add(block);
}
}
newGameButton = new JButton("New Game");
newGameButton.addActionListener(this);
scoreLabel = new JLabel("Score: 0");
scoreLabel.setHorizontalAlignment(SwingConstants.CENTER);
scoreLabel.setPreferredSize(new Dimension(BOARD_WIDTH, 50));
Container contentPane = getContentPane();
contentPane.setLayout(new BorderLayout());
contentPane.add(boardPanel, BorderLayout.CENTER);
contentPane.add(newGameButton, BorderLayout.NORTH);
contentPane.add(scoreLabel, BorderLayout.SOUTH);
board = new int[ROWS][COLS];
score = 0;
isDragging = false;
startNewGame();
}
public void actionPerformed(ActionEvent e) {
Object source = e.getSource();
if (source == newGameButton) {
startNewGame();
} else {
JButton block = (JButton) source;
int row = -1, col = -1;
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
if (blocks[i][j] == block) {
row = i;
col = j;
break;
}
}
if (row != -1) {
break;
}
}
if (!isDragging) {
if (isValidBlock(row, col)) {
selectBlock(row, col);
}
} else {
if (isValidBlock(row, col) && isAdjacent(row, col, dragStartRow, dragStartCol)) {
selectBlock(row, col);
eliminateSelectedBlocks();
} else {
swapBlocks(blocks[dragStartRow][dragStartCol], blocks[dragEndRow][dragEndCol]);
}
isDragging = false;
}
}
}
private void startNewGame() {
for (int row = 0; row < ROWS; row++) {
for (int col = 0; col < COLS; col++) {
board[row][col] = (int) (Math.random() * COLORS.length);
blocks[row][col].setBackground(COLORS[board[row][col]]);
blocks[row][col].setOpaque(true);
}
}
score = 0;
updateScoreLabel();
}
private boolean isValidBlock(int row, int col) {
return board[row][col] != -1;
}
private void selectBlock(int row, int col) {
resetSelectedBlocks();
int color = board[row][col];
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
if (board[i][j] == color) {
blocks[i][j].setBackground(Color.YELLOW);
blocks[i][j].setOpaque(true);
}
}
}
}
private void resetSelectedBlocks() {
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
if (board[i][j] != -1) {
blocks[i][j].setBackground(COLORS[board[i][j]]);
blocks[i][j].setOpaque(true);
}
}
}
}
private boolean isAdjacent(int row1, int col1, int row2, int col2) {
int rowDiff = Math.abs(row1 - row2);
int colDiff = Math.abs(col1 - col2);
return (rowDiff == 1 && colDiff == 0) || (rowDiff == 0 && colDiff == 1);
}
private void swapBlocks(JButton block1, JButton block2) {
int row1 = -1, col1 = -1, row2 = -1, col2 = -1;
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
if (blocks[i][j] == block1) {
row1 = i;
col1 = j;
} else if (blocks[i][j] == block2) {
row2 = i;
col2 = j;
}
}
}
int temp = board[row1][col1];
board[row1][col1] = board[row2][col2];
board[row2][col2] = temp;
block1.setBackground(COLORS[board[row1][col1]]);
block2.setBackground(COLORS[board[row2][col2]]);
block1.setOpaque(true);
block2.setOpaque(true);
}
private void eliminateSelectedBlocks() {
int color = board[dragStartRow][dragStartCol];
boolean[][] visited = new boolean[ROWS][COLS];
int blocksToMatch = 0;
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
if (board[i][j] == color && !visited[i][j]) {
blocksToMatch++;
if (blocksToMatch >= MIN_BLOCKS_TO_MATCH) {
break;
}
if (isEliminatable(i, j, visited)) {
eliminateBlocks(i, j, visited);
score += blocksToMatch;
updateScoreLabel();
shiftBlocksDown();
fillEmptyBlocks();
return;
}
}
}
if (blocksToMatch >= MIN_BLOCKS_TO_MATCH) {
break;
}
}
resetSelectedBlocks();
}
private boolean isEliminatable(int row, int col, boolean[][] visited) {
if (row < 0 || row >= ROWS || col < 0 || col >= COLS || board[row][col] == -1 || visited[row][col]) {
return false;
}
visited[row][col] = true;
return board[row][col] == board[dragStartRow][dragStartCol]
&& (isEliminatable(row - 1, col, visited) || isEliminatable(row + 1, col, visited)
|| isEliminatable(row, col - 1, visited) || isEliminatable(row, col + 1, visited));
}
private void eliminateBlocks(int row, int col, boolean[][] visited) {
if (row < 0 || row >= ROWS || col < 0 || col >= COLS || board[row][col] != board[dragStartRow][dragStartCol]
|| visited[row][col]) {
return;
}
visited[row][col] = true;
blocks[row][col].setBackground(Color.BLACK);
blocks[row][col].setOpaque(true);
board[row][col] = -1;
eliminateBlocks(row - 1, col, visited);
eliminateBlocks(row + 1, col, visited);
eliminateBlocks(row, col - 1, visited);
eliminateBlocks(row, col + 1, visited);
}
private void shiftBlocksDown() {
for (int col = 0; col < COLS; col++) {
int offset = 0;
for (int row = ROWS - 1; row >= 0; row--) {
if (board[row][col] != -1) {
if (offset > 0) {
board[row + offset][col] = board[row][col];
board[row][col] = -1;
blocks[row][col].setBackground(COLORS[board[row + offset][col]]);
blocks[row][col].setOpaque(true);
}
} else {
offset++;
}
}
}
}
private void fillEmptyBlocks() {
for (int col = 0; col < COLS; col++) {
int numEmptyBlocks = 0;
for (int row = ROWS - 1; row >= 0; row--) {
if (board[row][col] == -1) {
numEmptyBlocks++;
}
}
for (int i = 0; i < numEmptyBlocks; i++) {
int color = (int) (Math.random() * COLORS.length);
board[ROWS - 1 - i][col] = color;
blocks[ROWS - 1 - i][col].setBackground(COLORS[color]);
blocks[ROWS - 1 - i][col].setOpaque(true);
}
}
}
private void updateScoreLabel() {
scoreLabel.setText("Score: " + score);
}
public static void main(String[] args) {
new HappyElimination().setVisible(true);
}
}
```
这个游戏与其他消消乐游戏类似,你可以使用鼠标拖动相邻的方块来交换它们的位置,如果有三个或以上的同色方块连在一起,它们就会消除并得分。游戏中还有一个“New Game”按钮,可以开始一局新游戏。
该代码利用了 Java Swing 的窗口和按钮组件,并使用了 GridLayout 和 MouseMotionListener 来管理游戏板上的方块。游戏逻辑被封装在 actionPerformed 方法中,负责处理用户操作和更新游戏状态。
该代码只是一个简单的示例,你可以根据需要对它进行修改和扩展,以实现更复杂的游戏逻辑和用户界面。
阅读全文
相关推荐

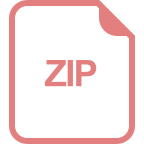
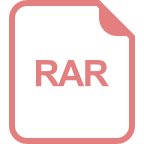
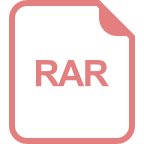
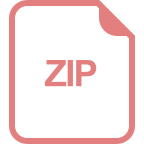
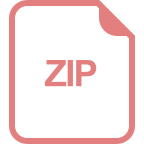
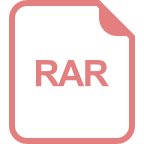
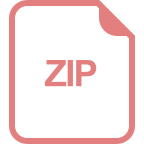
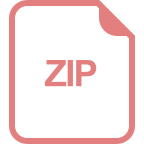
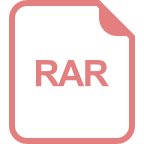
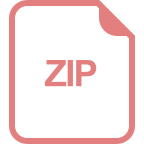