C语言:如何实现在终端输入某个字符或文,自动刷新前面已经显示的文字
时间: 2024-03-22 15:37:40 浏览: 130
在C语言中,可以使用特殊字符来实现终端的控制,比如使用"\r"来回到行首,使用"\b"来退格,使用"\033[K"来清除当前光标到行尾的内容。如果要实现在终端输入某个字符或文,自动刷新前面已经显示的文字,可以使用"\r"回到行首,然后再输出新的内容即可。
例如,以下代码可以实现每隔一秒输出当前时间,并在下一秒更新时间:
```c
#include <stdio.h>
#include <time.h>
int main() {
while (1) {
time_t t = time(NULL);
struct tm* tm = localtime(&t);
printf("\r%s", asctime(tm));
fflush(stdout);
sleep(1);
}
return 0;
}
```
在这段代码中,"\r"用来回到行首,然后输出当前时间的字符串,再使用fflush函数来强制刷新输出缓冲区,使得输出立即显示在终端上。然后使用sleep函数来暂停1秒,等待下一次更新。
相关问题
C语言 如何在终端中实现输入某个字符,自动清除前面已经显示的文字或字符。
在 C 语言中,可以使用 ANSI Escape Sequences 实现在终端中清除前面已经显示的文字或字符。
具体来说,可以使用以下代码清除终端中已经显示的文字或字符:
```c
#include <stdio.h>
int main() {
printf("Please enter a character: ");
fflush(stdout); // 刷新输出缓冲区
char c = getchar(); // 获取用户输入的字符
printf("\033[2K"); // 清除当前行
printf("You entered: %c\n", c);
return 0;
}
```
在上述代码中,`\033[2K` 是 ANSI Escape Sequences 中的一种,表示清除当前行(即将光标移动到行首并清除整行内容)。使用这个代码可以在终端中清除前面已经显示的文字或字符。
用C语言的graphics库做一个在图形窗口的输入程序。当用户按键时,在图形窗口出现按下的键所代表的字符,并像图形界面的输入框一样自动向右递进,按下回车键结束输入,将用户输入的内容在终端窗口显示出来。并在此基础上,实现当前位置光标闪烁,并支持左右方向键和两个删除键。给出代码
以下是一个简单的实现,使用了graphics.h库和conio.h库。
```c
#include <stdio.h>
#include <conio.h>
#include <graphics.h>
int main() {
int gd = DETECT, gm;
initgraph(&gd, &gm, "");
cleardevice();
int x = 100, y = 100;
char str[100] = "";
int len = 0;
int cursor = 0;
while (1) {
if (kbhit()) {
char c = getch();
if (c == '\r') {
break;
} else if (c == '\b') {
if (cursor > 0) {
cursor--;
len--;
str[cursor] = '\0';
}
} else if (c == 224) {
c = getch();
if (c == 75 && cursor > 0) { // left arrow
cursor--;
} else if (c == 77 && cursor < len) { // right arrow
cursor++;
} else if (c == 83 && cursor < len) { // delete
for (int i = cursor; i < len; i++) {
str[i] = str[i+1];
}
len--;
str[len] = '\0';
}
} else if (len < 99) {
for (int i = len; i >= cursor; i--) {
str[i+1] = str[i];
}
str[cursor] = c;
cursor++;
len++;
str[len] = '\0';
}
}
settextstyle(DEFAULT_FONT, HORIZ_DIR, 2);
outtextxy(x, y, str);
if (cursor < len) {
int w = textwidth(str);
setcolor(getbkcolor());
line(x+w, y, x+w, y+textheight("A"));
} else {
int w = textwidth("_");
setcolor(gettextcolor());
line(x+textwidth(str), y, x+textwidth(str), y+textheight("A"));
outtextxy(x+textwidth(str), y, "_");
}
delay(100);
cleardevice();
}
closegraph();
printf("%s\n", str);
return 0;
}
```
这个程序使用了initgraph函数初始化图形窗口,然后使用outtextxy函数在指定位置输出文本。当用户按下键盘时,根据按键进行相应操作,比如添加字符、删除字符、移动光标等。为了支持光标闪烁,我们在光标所在位置输出一个下划线,并定时刷新窗口实现闪烁效果。最后,将用户输入的内容输出到终端窗口。
阅读全文
相关推荐
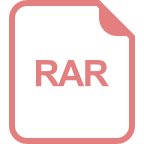
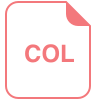
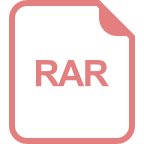
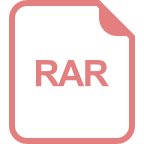
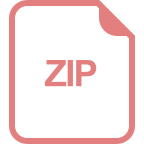
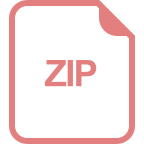
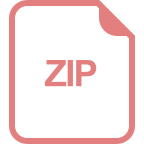
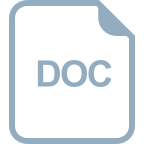
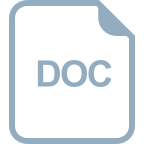
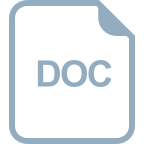
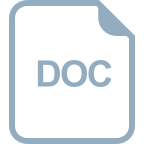



