请翻译这段c++语言 bool operator()(const QueuedWire &lhs, const QueuedWire &rhs) const noexcept
时间: 2024-01-18 21:06:11 浏览: 121
这段 C++ 代码定义了一个名为 `operator()` 的函数,它是一个类成员函数,返回值类型为 `bool`,接受两个参数,均为类型为 `QueuedWire` 的常量引用。该函数带有 `const` 限定符,并且不会抛出异常(即 `noexcept` 关键字)。这个函数重载了函数调用运算符 `()`,可以用于对 `QueuedWire` 对象进行比较。
相关问题
如果您的结构体中包含 QMap<自定义枚举类型, QMap<自定义枚举类型, 子结构体>>,则需要分别为该结构体、子结构体以及自定义枚举类型定义等号和不等号运算符。 先来看自定义枚举类型的重载运算符。假设该枚举类型的名称为 MyEnum,需要按照以下方式定义 == 和 != 运算符: 复制 enum class MyEnum { A, B, C }; bool operator==(const MyEnum& lhs, const MyEnum& rhs) { return static_cast<int>(lhs) == static_cast<int>(rhs); } bool operator!=(const MyEnum& lhs, const MyEnum& rhs) { return !(lhs == rhs); } 在上述代码中,我们将 MyEnum 转换为 int 类型进行比较,因为 enum class 默认没有定义等号和不等号运算符。 接下来是子结构体的重载运算符,假设子结构体的名称为 SubStruct,包含两个整数 x 和 y,则需要按照以下方式定义 == 和 != 运算符: 复制 struct SubStruct { int x; int y; bool operator==(const SubStruct& other) const { return x == other.x && y == other.y; } bool operator!=(const SubStruct& other) const { return !(*this == other); } }; 最后是包含 QMap<自定义枚举类型, QMap<自定义枚举类型, SubStruct>> 的结构体的重载运算符,假设该结构体的名称为 MyStruct,需要按照以下方式定义 == 和 != 运算符: 复制 struct MyStruct { QMap<MyEnum, QMap<MyEnum, SubStruct>> aa; bool operator==(const MyStruct& other) const { return aa == other.aa; } bool operator!=(const MyStruct& other) const { return !(*this == other); } }; 在上述代码中,我们直接利用了 QMap 的默认等号运算符,因为其已经对子结构体进行了深度比较。因此,我们只需要为 MyStruct 定义等号和不等号运算符,将其与其他 MyStruct 对象进行比较即可。,你的这种方法系统会报错
& lhs, const MyEnum& rhs) {
return static_cast<int>(lhs) == static_cast<int>(rhs);
}
bool operator!=(const MyEnum& lhs, const MyEnum& rhs) {
return !(lhs == rhs);
}
这样就可以对自定义枚举类型进行 == 和 != 运算符的重载了。接下来是子结构体的重载运算符定义。假设该子结构体的名称为 MyStruct,包含两个成员变量:一个是自定义枚举类型 MyEnum,另一个是 QString 类型的字符串。需要按照以下方式定义 == 和 != 运算符:
复制 struct MyStruct {
MyEnum enumVar;
QString stringVar;
bool operator==(const MyStruct& other) const {
return (enumVar == other.enumVar) && (stringVar == other.stringVar);
}
bool operator!=(const MyStruct& other) const {
return !(*this == other);
}
};
最后是包含 QMap<自定义枚举类型, QMap<自定义枚举类型, 子结构体>> 的结构体的重载运算符定义。假设该结构体的名称为 MyStructWithMap,需要按照以下方式定义 == 和 != 运算符:
复制 struct MyStructWithMap {
QMap<MyEnum, QMap<MyEnum, MyStruct>> mapVar;
bool operator==(const MyStructWithMap& other) const {
return mapVar == other.mapVar;
}
bool operator!=(const MyStructWithMap& other) const {
return !(*this == other);
}
};
编写c++代码:在executor_seq_scan函数中实现函数satisfiesConditions(const Record& record) ,使其能够找到满足条件的记录record, 记录Record为一个结构体,包括: char* data; // 记录的数据 int size; // 记录的大小 bool allocated_ = false; // 是否已经为数据分配空间 条件condition也为一个结构体: struct Condition { TabCol lhs_col; // left-hand side column CompOp op; // comparison operator bool is_rhs_val; // true if right-hand side is a value (not a column) TabCol rhs_col; // right-hand side column Value rhs_val; // right-hand side value };
为了实现函数`satisfiesConditions(const Record& record)`,需要先了解`Record`和`Condition`的定义。假设`TabCol`、`CompOp`和`Value`都已经定义完毕,我将根据这些定义为您编写代码。
```cpp
#include <cstring> // for strcmp
struct Record {
char* data; // 记录的数据
int size; // 记录的大小
bool allocated_; // 是否已经为数据分配空间
};
struct Condition {
TabCol lhs_col; // 左操作数列
CompOp op; // 比较操作符
bool is_rhs_val; // 如果右操作数是值而不是列,则为true
TabCol rhs_col; // 右操作数列
Value rhs_val; // 右操作数值
};
// 满足条件的函数
bool satisfiesConditions(const Record& record, const std::vector<Condition>& conditions) {
for (const auto& condition : conditions) {
// 获取条件的左操作数和右操作数
TabCol lhs_col = condition.lhs_col;
CompOp op = condition.op;
bool is_rhs_val = condition.is_rhs_val;
TabCol rhs_col = condition.rhs_col;
Value rhs_val = condition.rhs_val;
// 根据比较操作符进行比较
if (is_rhs_val) {
// 如果右操作数是值
// 进行相应的比较逻辑
if (op == CompOp::EQ) {
if (strcmp(record.data, rhs_val) != 0) {
return false;
}
} else if (op == CompOp::NEQ) {
if (strcmp(record.data, rhs_val) == 0) {
return false;
}
} // 其他比较操作符类似
} else {
// 如果右操作数是列
// 进行相应的比较逻辑
// 这里需要获取右操作数列的数据,并进行比较
}
}
return true;
}
// 在executor_seq_scan函数中使用satisfiesConditions函数
void executor_seq_scan() {
// 这里是您的代码逻辑
// ...
std::vector<Condition> conditions = {
// 这里是您的条件定义
};
Record record;
record.data = new char[record.size];
record.allocated_ = true;
// 调用satisfiesConditions函数判断记录是否满足条件
bool is_satisfied = satisfiesConditions(record, conditions);
// ...
if (record.allocated_) {
delete[] record.data;
}
}
```
请注意,这只是一个示例代码,您需要根据实际情况进行修改。在比较操作符的逻辑中,我使用了`strcmp`函数来比较字符串类型的数据,您可能需要根据实际需求来进行相应的比较操作。同时,请确保在不再使用`Record`对象时,释放为其分配的内存。
阅读全文
相关推荐
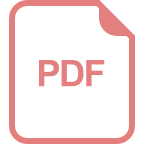
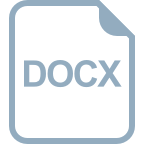
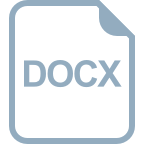
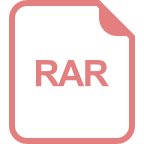
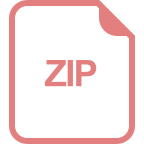
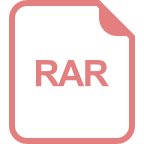
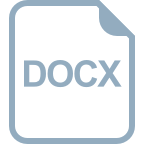
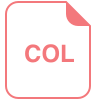
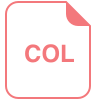





