导入pyspark.conf,pyspark.sparkcontext,pyspark.mllib,实现SVM对于新闻的分类。数据集为多个按照类别分类的文件夹,每个文件夹下为新闻的中文正文内容,采用tf-idf对数据集进行清洗和处理,得到RDD。
时间: 2023-12-14 09:38:43 浏览: 91
首先,需要安装并配置好PySpark环境。然后,可以按照以下步骤实现SVM对于新闻的分类:
1. 导入必要的库和模块:
```
from pyspark.conf import SparkConf
from pyspark.context import SparkContext
from pyspark.mllib.feature import HashingTF, IDF
from pyspark.mllib.regression import LabeledPoint
from pyspark.mllib.classification import SVMWithSGD
import jieba
import os
```
2. 创建SparkContext:
```
conf = SparkConf().setAppName("News Classification").setMaster("local")
sc = SparkContext(conf=conf)
```
3. 定义函数用于读取数据集和分词处理:
```
def read_file(path):
with open(path, 'r', encoding='utf-8') as f:
text = f.read()
return text
def jieba_cut(text):
words = list(jieba.cut(text))
return words
```
4. 加载数据集并进行分词处理:
```
data = []
for category in os.listdir('data'):
for file in os.listdir(os.path.join('data', category)):
path = os.path.join('data', category, file)
text = read_file(path)
words = jieba_cut(text)
data.append((category, words))
```
5. 使用HashingTF和IDF对文本进行特征提取:
```
hashingTF = HashingTF()
tf = hashingTF.transform(data.map(lambda x: x[1]))
tf.cache()
idf = IDF().fit(tf)
tfidf = idf.transform(tf)
```
6. 将特征向量和标签打包成LabeledPoint:
```
def label_point(x):
category = x[0]
features = x[1]
label = 0
if category == 'business':
label = 0
elif category == 'entertainment':
label = 1
elif category == 'sports':
label = 2
elif category == 'tech':
label = 3
return LabeledPoint(label, features)
labeled_data = tfidf.zip(data.map(lambda x: x[0])).map(label_point)
```
7. 将数据集划分为训练集和测试集:
```
train_data, test_data = labeled_data.randomSplit([0.8, 0.2])
```
8. 训练SVM模型:
```
model = SVMWithSGD.train(train_data)
```
9. 在测试集上评估模型性能:
```
predictions = model.predict(test_data.map(lambda x: x.features))
labels_and_preds = test_data.map(lambda x: x.label).zip(predictions)
accuracy = labels_and_preds.filter(lambda x: x[0] == x[1]).count() / float(test_data.count())
print("Accuracy = " + str(accuracy))
```
以上就是使用PySpark实现SVM对于新闻的分类的步骤。
阅读全文
相关推荐
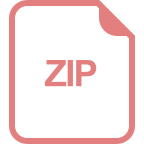
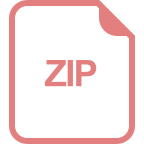
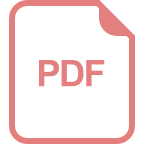


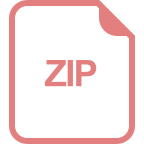








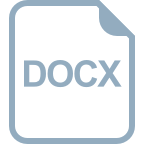
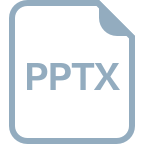
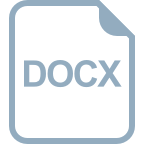
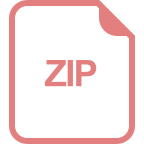
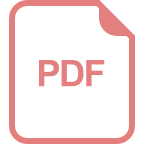