java推箱子小游戏代码
时间: 2023-07-09 21:20:37 浏览: 102
推箱子小游戏是一款经典的益智游戏,以下是 Java 实现的推箱子小游戏代码:
```java
import java.util.Scanner;
public class PushBoxGame {
// 地图
private static char[][] map = {
{'#', '#', '#', '#', '#', '#', '#', '#', '#', '#'},
{'#', ' ', ' ', ' ', '#', ' ', ' ', ' ', ' ', '#'},
{'#', ' ', ' ', ' ', '#', ' ', ' ', ' ', ' ', '#'},
{'#', ' ', ' ', ' ', ' ', ' ', '#', '#', '#', '#'},
{'#', ' ', ' ', ' ', ' ', ' ', '#', ' ', ' ', '#'},
{'#', ' ', ' ', ' ', '#', ' ', ' ', ' ', ' ', '#'},
{'#', ' ', ' ', ' ', '#', ' ', ' ', ' ', ' ', '#'},
{'#', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', '#'},
{'#', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', '#'},
{'#', '#', '#', '#', '#', '#', '#', '#', '#', '#'}
};
// 箱子的位置
private static int boxX = 4;
private static int boxY = 7;
// 人的位置
private static int manX = 4;
private static int manY = 5;
// 游戏是否结束
private static boolean isGameOver = false;
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
while (!isGameOver) {
// 打印地图
printMap();
// 获取用户输入的方向
System.out.print("请输入方向(w:上 s:下 a:左 d:右):");
String direction = scanner.nextLine();
// 移动箱子和人
moveBoxAndMan(direction);
// 判断游戏是否结束
checkGameOver();
}
}
/**
* 打印地图
*/
private static void printMap() {
for (int i = 0; i < 10; i++) {
for (int j = 0; j < 10; j++) {
if (i == manX && j == manY) {
System.out.print("人 ");
} else if (i == boxX && j == boxY) {
System.out.print("箱 ");
} else {
System.out.print(map[i][j] + " ");
}
}
System.out.println();
}
}
/**
* 移动箱子和人
*
* @param direction 移动方向
*/
private static void moveBoxAndMan(String direction) {
int dx = 0, dy = 0; // 箱子的移动距离
switch (direction) {
case "w": // 上
dx = -1;
break;
case "s": // 下
dx = 1;
break;
case "a": // 左
dy = -1;
break;
case "d": // 右
dy = 1;
break;
}
// 判断人的下一个位置是否为墙或者箱子
int nextManX = manX + dx;
int nextManY = manY + dy;
if (map[nextManX][nextManY] == '#' || (nextManX == boxX && nextManY == boxY)) {
return;
}
// 移动箱子
int nextBoxX = boxX + dx;
int nextBoxY = boxY + dy;
if (nextBoxX >= 0 && nextBoxX < 10 && nextBoxY >= 0 && nextBoxY < 10 && map[nextBoxX][nextBoxY] != '#') {
boxX = nextBoxX;
boxY = nextBoxY;
}
// 移动人
manX = nextManX;
manY = nextManY;
}
/**
* 检查游戏是否结束
*/
private static void checkGameOver() {
if (boxX == 2 && boxY == 8) { // 箱子到达目标位置
System.out.println("游戏结束,恭喜你获胜!");
isGameOver = true;
}
}
}
```
在这个实现中,地图使用二维数组表示,人和箱子的位置用变量表示。每次移动时,先判断人的下一个位置是否为墙或者箱子,如果是,则不做任何操作;否则,移动人的位置,并判断箱子是否能够移动,如果可以,则移动箱子的位置。最后,检查游戏是否结束,如果箱子到达目标位置,游戏结束。
阅读全文
相关推荐
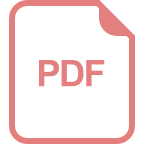
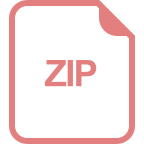
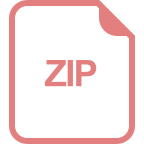













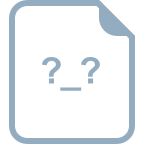
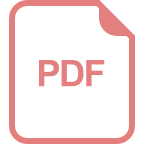
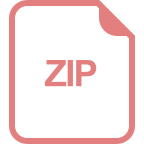