已知一棵树的节点间关系,请编程实现该树的先根遍历(用C++)
时间: 2024-03-17 20:43:19 浏览: 25
假设树的节点定义如下:
```
struct TreeNode {
int val;
vector<TreeNode*> children;
TreeNode(int x) : val(x) {}
};
```
先根遍历的递归实现如下:
```
void preOrder(TreeNode* root) {
if (root == nullptr) return;
cout << root->val << " ";
for (auto child : root->children) {
preOrder(child);
}
}
```
非递归实现可以借助栈来实现,具体代码如下:
```
void preOrder(TreeNode* root) {
if (root == nullptr) return;
stack<TreeNode*> st;
st.push(root);
while (!st.empty()) {
TreeNode* cur = st.top();
st.pop();
cout << cur->val << " ";
for (int i = cur->children.size() - 1; i >= 0; --i) {
if (cur->children[i] != nullptr) {
st.push(cur->children[i]);
}
}
}
}
```
以上两种方法都可以实现树的先根遍历,具体选择哪种方法取决于实际应用场景。
相关推荐













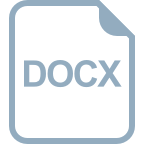