pytorch实现LDAM损失函数
时间: 2023-10-06 13:14:02 浏览: 139
LDAM(Label-Distribution-Aware Margin)是一种用于不平衡数据集分类的损失函数。它考虑到了类别之间的分布差异,可以有效地提高不平衡数据集分类的性能。
下面是使用PyTorch实现LDAM损失函数的代码:
```python
import torch
import torch.nn.functional as F
class LDAMLoss(torch.nn.Module):
def __init__(self, cls_num_list, max_m=0.5, weight=None, s=30):
super(LDAMLoss, self).__init__()
m_list = 1.0 / torch.sqrt(torch.sqrt(cls_num_list))
m_list = m_list * (max_m / torch.max(m_list))
self.m_list = m_list
self.s = s
self.weight = weight
def forward(self, x, target):
index = torch.zeros_like(x, dtype=torch.uint8)
index.scatter_(1, target.data.view(-1, 1), 1)
batch_size = x.size(0)
x_margin = x - self.m_list.view(1, -1).expand(batch_size, -1)
output = torch.where(index, x_margin, x)
output *= self.s
if self.weight is not None:
output *= self.weight.view(1, -1).expand(batch_size, -1)
loss = F.cross_entropy(output, target)
return loss
```
其中,`cls_num_list`是一个长度为类别数的列表,表示每个类别在训练集中的样本数。`max_m`是一个超参数,控制最大的margin值。`weight`是一个长度为类别数的权重列表,可以用于调整不同类别的重要性。`s`是一个缩放因子,可以用于控制margin的大小。
在`forward`函数中,首先根据`cls_num_list`计算每个类别的margin值。然后根据标签构造一个one-hot向量`index`,并将margin值分别减去到每个类别对应的位置上。接着,将得到的张量乘以缩放因子`s`,再根据权重调整不同类别的重要性。最后,使用交叉熵损失函数计算损失值。
使用示例:
```python
# 假设有5个类别,每个类别的样本数分别为100, 200, 300, 400, 500
cls_num_list = [100, 200, 300, 400, 500]
ldam_loss = LDAMLoss(cls_num_list)
x = torch.randn(10, 5) # 假设有10个样本,每个样本有5个特征
y = torch.LongTensor([0, 1, 2, 3, 4, 0, 1, 2, 3, 4]) # 对应的标签
loss = ldam_loss(x, y)
```
相关推荐
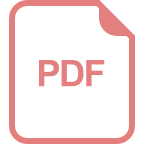
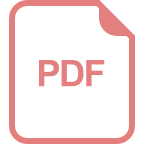
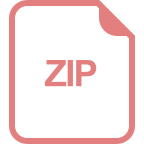














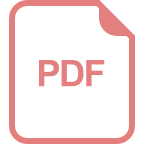