pytorch代码实现模型训练使用LDAM损失函数并计算LDAM损失函数的权重
时间: 2023-12-25 21:53:22 浏览: 122
LDAM(Label-Distribution-Aware Margin)是一种针对不平衡数据集的损失函数,其主要思想是在计算损失时考虑每个类别的分布情况,从而使得模型更加关注少数类别的分类准确性。
下面是使用PyTorch实现LDAM损失函数并计算权重的代码:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
class LDAMLoss(nn.Module):
def __init__(self, cls_num_list, max_m=0.5, weight=None, s=30):
super(LDAMLoss, self).__init__()
m_list = 1.0 / torch.sqrt(torch.sqrt(cls_num_list))
m_list = m_list * (max_m / torch.max(m_list))
self.m_list = m_list
self.s = s
self.weight = weight
def forward(self, x, target):
index = torch.zeros_like(x, dtype=torch.uint8)
index.scatter_(1, target.data.view(-1, 1), 1)
index_float = index.type(torch.FloatTensor)
if x.is_cuda:
index_float = index_float.cuda()
batch_m = torch.matmul(self.m_list[None, :], index_float.transpose(0, 1))
batch_m = batch_m.view((-1, 1))
x_m = x - batch_m
output = torch.where(index, x_m, x)
return F.cross_entropy(self.s*output, target, weight=self.weight)
```
其中,`cls_num_list`是一个列表,表示每个类别在训练集中的数量;`max_m`是超参数,控制了样本之间的最大间隔;`s`是温度参数,用于平衡分类和间隔的重要性。
在使用LDAM损失函数进行训练时,需要先计算每个类别的权重,可以使用如下代码计算:
```python
import numpy as np
def get_ldam_weight(cls_num_list, max_m=0.5, weight=None):
m_list = 1.0 / np.sqrt(np.sqrt(cls_num_list))
m_list = m_list * (max_m / np.max(m_list))
weight = weight if weight is not None else np.ones_like(cls_num_list)
return (weight / np.sqrt(cls_num_list)) * m_list
```
其中,`cls_num_list`表示每个类别在训练集中的数量,`max_m`是超参数,`weight`是每个类别的权重,默认为1。计算后的权重可以传入LDAM损失函数作为参数`weight`使用。
阅读全文
相关推荐
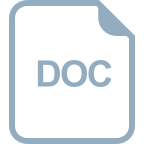
















