#include <iostream> using namespace std; int main() { for (int f = 0; f <= 300; f += 20) { float c = 5.0 / 9.0 * (f - 32.0); printf("%4d%10.1f\n", f, c); } return 0; },用C语言编程
时间: 2024-01-24 14:01:53 浏览: 105
下面是用C语言实现该功能的代码:
```c
#include <stdio.h>
int main() {
for (int f = 0; f <= 300; f += 20) {
float c = 5.0 / 9.0 * (f - 32.0);
printf("%4d%10.1f\n", f, c);
}
return 0;
}
```
输出结果和C++版本一样:
```
0 -17.8
20 -6.7
40 4.4
60 15.6
80 26.7
100 37.8
120 48.9
140 60.0
160 71.1
180 82.2
200 93.3
220 104.4
240 115.6
260 126.7
280 137.8
300 148.9
```
相关问题
#include <iostream> #include <vector> using namespace std; int main() { int n; cin >> n; vector<int> a; for(int i = 0; i < n;i++) { cin >> a[i]; } return 0; }
这段代码存在问题,vector 容器在使用前需要先分配空间,可以使用 resize() 函数或者在构造函数中指定大小。否则会出现访问越界的错误。可以将代码改成如下:
```
#include <iostream>
#include <vector>
using namespace std;
int main() {
int n;
cin >> n;
vector<int> a(n); //分配空间
for(int i = 0; i < n;i++) {
cin >> a[i];
}
return 0;
}
```
#include <iostream>#include<string> using namespace std; int main() { int a = 12; int& r; r = a; r=10; cout << a; return 0:
这段代码有错误,因为在第7行中,声明了一个引用r,但是没有初始化,即没有指向任何一个变量。正确的代码应该是:
```
#include <iostream>
using namespace std;
int main() {
int a = 12;
int& r = a; // 将r初始化为a的引用
r = 10; // 修改r的值,实际上也会修改a的值
cout << a; // 输出a的值
return 0;
}
```
输出结果为10。
阅读全文
相关推荐
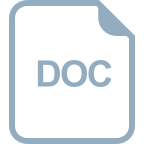
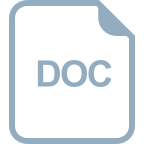
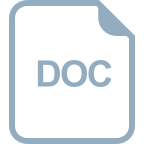














