用Qt的QGraphicsScene、QGraphicsItem与QGraphicsView写一份用于绘制板块热力图的代码,要求代码完整且详细
时间: 2024-01-24 12:18:50 浏览: 32
好的,我会尽力为您解答。首先,让我们来了解一下Qt的QGraphicsScene、QGraphicsItem和QGraphicsView。
QGraphicsScene是一个二维图形场景,可以在其中添加QGraphicsItem,QGraphicsItem是图形场景中的图形项,可以是简单的图形元素,如线、椭圆、矩形等,也可以是复杂的自定义图形元素。QGraphicsView则是场景的视图,可以在其中显示场景中的图形项,还可以进行缩放、平移等交互操作。
要实现绘制板块热力图的功能,我们需要先创建一个QGraphicsScene对象,然后添加QGraphicsItem,最后在QGraphicsView中显示出来。具体的代码实现如下:
1. 创建QGraphicsScene对象:
```cpp
QGraphicsScene *scene = new QGraphicsScene(this);
```
2. 创建一个自定义的QGraphicsItem子类,用于表示每个板块的热度:
```cpp
class HeatMapItem : public QGraphicsItem
{
public:
HeatMapItem(QRectF rect, qreal value, qreal maxValue, QColor baseColor, QColor hotColor, QGraphicsItem *parent = nullptr);
QRectF boundingRect() const override;
void paint(QPainter *painter, const QStyleOptionGraphicsItem *option, QWidget *widget = nullptr) override;
void setValue(qreal value);
private:
QRectF m_rect;
qreal m_value;
qreal m_maxValue;
QColor m_baseColor;
QColor m_hotColor;
};
```
3. 在自定义的QGraphicsItem子类中实现boundingRect()函数,用于返回图形项的边界矩形:
```cpp
QRectF HeatMapItem::boundingRect() const
{
return m_rect;
}
```
4. 在自定义的QGraphicsItem子类中实现paint()函数,用于绘制图形项的热度:
```cpp
void HeatMapItem::paint(QPainter *painter, const QStyleOptionGraphicsItem *option, QWidget *widget)
{
// 根据热度值计算颜色
qreal ratio = m_value / m_maxValue;
QColor color = m_baseColor.lighter(100 - ratio * 100);
color.setAlphaF(ratio * 0.6);
// 绘制矩形
painter->fillRect(m_rect, color);
painter->drawRect(m_rect);
}
```
5. 在自定义的QGraphicsItem子类中实现setValue()函数,用于设置图形项的热度值:
```cpp
void HeatMapItem::setValue(qreal value)
{
m_value = value;
update();
}
```
6. 在自定义的QGraphicsItem子类的构造函数中初始化相关变量:
```cpp
HeatMapItem::HeatMapItem(QRectF rect, qreal value, qreal maxValue, QColor baseColor, QColor hotColor, QGraphicsItem *parent)
: QGraphicsItem(parent)
, m_rect(rect)
, m_value(value)
, m_maxValue(maxValue)
, m_baseColor(baseColor)
, m_hotColor(hotColor)
{
}
```
7. 在主窗口中添加QGraphicsView控件,并设置其场景为上面创建的QGraphicsScene对象:
```cpp
QGraphicsView *view = new QGraphicsView(this);
view->setScene(scene);
```
8. 在主窗口中添加多个自定义的QGraphicsItem子类对象,用于表示不同板块的热度:
```cpp
HeatMapItem *item1 = new HeatMapItem(QRectF(0, 0, 100, 100), 50, 100, Qt::blue, Qt::red);
HeatMapItem *item2 = new HeatMapItem(QRectF(100, 0, 100, 100), 80, 100, Qt::blue, Qt::red);
HeatMapItem *item3 = new HeatMapItem(QRectF(0, 100, 100, 100), 20, 100, Qt::blue, Qt::red);
HeatMapItem *item4 = new HeatMapItem(QRectF(100, 100, 100, 100), 90, 100, Qt::blue, Qt::red);
scene->addItem(item1);
scene->addItem(item2);
scene->addItem(item3);
scene->addItem(item4);
```
9. 最后,在主窗口中添加交互控件,如缩放、平移等功能,可以通过设置QGraphicsView的属性来实现。
完整代码如下:
```cpp
#include <QApplication>
#include <QGraphicsScene>
#include <QGraphicsView>
#include <QGraphicsItem>
#include <QPainter>
class HeatMapItem : public QGraphicsItem
{
public:
HeatMapItem(QRectF rect, qreal value, qreal maxValue, QColor baseColor, QColor hotColor, QGraphicsItem *parent = nullptr);
QRectF boundingRect() const override;
void paint(QPainter *painter, const QStyleOptionGraphicsItem *option, QWidget *widget = nullptr) override;
void setValue(qreal value);
private:
QRectF m_rect;
qreal m_value;
qreal m_maxValue;
QColor m_baseColor;
QColor m_hotColor;
};
HeatMapItem::HeatMapItem(QRectF rect, qreal value, qreal maxValue, QColor baseColor, QColor hotColor, QGraphicsItem *parent)
: QGraphicsItem(parent)
, m_rect(rect)
, m_value(value)
, m_maxValue(maxValue)
, m_baseColor(baseColor)
, m_hotColor(hotColor)
{
}
QRectF HeatMapItem::boundingRect() const
{
return m_rect;
}
void HeatMapItem::paint(QPainter *painter, const QStyleOptionGraphicsItem *option, QWidget *widget)
{
// 根据热度值计算颜色
qreal ratio = m_value / m_maxValue;
QColor color = m_baseColor.lighter(100 - ratio * 100);
color.setAlphaF(ratio * 0.6);
// 绘制矩形
painter->fillRect(m_rect, color);
painter->drawRect(m_rect);
}
void HeatMapItem::setValue(qreal value)
{
m_value = value;
update();
}
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
QGraphicsScene *scene = new QGraphicsScene();
QGraphicsView *view = new QGraphicsView();
view->setScene(scene);
HeatMapItem *item1 = new HeatMapItem(QRectF(0, 0, 100, 100), 50, 100, Qt::blue, Qt::red);
HeatMapItem *item2 = new HeatMapItem(QRectF(100, 0, 100, 100), 80, 100, Qt::blue, Qt::red);
HeatMapItem *item3 = new HeatMapItem(QRectF(0, 100, 100, 100), 20, 100, Qt::blue, Qt::red);
HeatMapItem *item4 = new HeatMapItem(QRectF(100, 100, 100, 100), 90, 100, Qt::blue, Qt::red);
scene->addItem(item1);
scene->addItem(item2);
scene->addItem(item3);
scene->addItem(item4);
view->show();
return a.exec();
}
```
希望这份代码能够对您有所帮助!
相关推荐
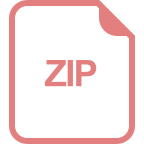














