netty websocket传输图片代码
时间: 2023-08-31 13:23:10 浏览: 85
### 回答1:
要在 Netty 中使用 WebSocket 传输图片,需要执行以下步骤:
1. 创建 WebSocket 服务端和客户端。
2. 在服务端处理器中,当收到新的 WebSocket 连接请求时,调用 `Channel.pipeline().addLast(new HttpObjectAggregator(65536))` 方法将 HTTP 消息聚合器添加到处理器链中。
3. 在客户端处理器中,当收到 WebSocket 握手响应消息时,调用 `Channel.pipeline().addLast(new HttpObjectAggregator(65536))` 方法将 HTTP 消息聚合器添加到处理器链中。
4. 在服务端处理器中,当收到 WebSocket 文本消息时,调用 `String encoded = Base64.getEncoder().encodeToString(bytes);` 将图片转换为 base64 编码的字符串。
5. 在客户端处理器中,当收到 WebSocket 文本消息时,调用 `byte[] decoded = Base64.getDecoder().decode(encoded);` 将 base64 编码的字符串转换为图片。
以下是一个简单的示例代码:
```java
// 服务端代码
public class WebSocketServer {
public static void main(String[] args) throws Exception {
EventLoopGroup bossGroup = new NioEventLoopGroup();
EventLoopGroup workerGroup = new NioEventLoopGroup();
try {
ServerBootstrap b = new ServerBootstrap();
b.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new WebSocketServerInitializer());
Channel ch = b.bind(8888).sync().channel();
ch.closeFuture().sync();
} finally {
bossGroup.shutdownGracefully();
workerGroup.shutdownGracefully();
}
}
}
class WebSocketServerInitializer extends ChannelInitializer<SocketChannel> {
@Override
public void initChannel(Socket
### 回答2:
open jdk11:
```java
import io.netty.bootstrap.ServerBootstrap;
import io.netty.buffer.ByteBuf;
import io.netty.channel.ChannelHandlerContext;
import io.netty.channel.ChannelInitializer;
import io.netty.channel.ChannelOption;
import io.netty.channel.SimpleChannelInboundHandler;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.SocketChannel;
import io.netty.channel.socket.nio.NioServerSocketChannel;
import io.netty.handler.codec.http.*;
import io.netty.handler.codec.http.websocketx.*;
import io.netty.handler.stream.ChunkedWriteHandler;
import io.netty.util.CharsetUtil;
import javax.imageio.ImageIO;
import java.awt.image.BufferedImage;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.nio.file.Files;
public class NettyWebSocketImageServer {
private final int port;
public NettyWebSocketImageServer(int port) {
this.port = port;
}
public void run() throws Exception {
NioEventLoopGroup bossGroup = new NioEventLoopGroup();
NioEventLoopGroup workerGroup = new NioEventLoopGroup();
try {
ServerBootstrap b = new ServerBootstrap();
b.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
public void initChannel(SocketChannel ch) {
ch.pipeline().addLast(new HttpServerCodec());
ch.pipeline().addLast(new HttpObjectAggregator(64 * 1024));
ch.pipeline().addLast(new ChunkedWriteHandler());
ch.pipeline().addLast(new WebSocketServerProtocolHandler("/image"));
ch.pipeline().addLast(new WebSocketImageHandler());
}
})
.option(ChannelOption.SO_BACKLOG, 128)
.childOption(ChannelOption.SO_KEEPALIVE, true);
System.out.println("Server started");
b.bind(port).sync().channel().closeFuture().sync();
} finally {
bossGroup.shutdownGracefully();
workerGroup.shutdownGracefully();
}
}
public static void main(String[] args) throws Exception {
int port = 8080;
NettyWebSocketImageServer server = new NettyWebSocketImageServer(port);
server.run();
}
public static class WebSocketImageHandler extends SimpleChannelInboundHandler<WebSocketFrame> {
private static final String IMAGE_PATH = "path_of_your_image"; // Replace with actual image path
@Override
protected void channelRead0(ChannelHandlerContext ctx, WebSocketFrame frame) throws Exception {
if (frame instanceof TextWebSocketFrame) {
// Handle text frame
String request = ((TextWebSocketFrame) frame).text();
System.out.println("Received request: " + request);
// Load image and send as binary data
File file = new File(IMAGE_PATH);
if (file.exists() && file.isFile()) {
byte[] imageBytes = Files.readAllBytes(file.toPath());
BufferedImage image = ImageIO.read(file);
ByteArrayOutputStream baos = new ByteArrayOutputStream();
ImageIO.write(image, "jpg", baos);
byte[] encodedImage = baos.toByteArray();
BinaryWebSocketFrame response = new BinaryWebSocketFrame(Unpooled.wrappedBuffer(encodedImage));
ctx.writeAndFlush(response);
}
} else if (frame instanceof CloseWebSocketFrame) {
ctx.close();
}
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) {
cause.printStackTrace();
ctx.close();
}
}
}
```
客户端代码:
```javascript
const WebSocket = require('ws');
const fs = require('fs');
const ws = new WebSocket('ws://localhost:8080/image');
ws.on('open', function open() {
ws.send('Please send the image');
});
ws.on('message', function incoming(data) {
const buffer = new Uint8Array(data);
fs.writeFileSync('receivedImage.jpg', buffer);
});
ws.on('close', function close() {
console.log('Connection closed');
});
```
这段代码创建了一个基于Netty的WebSocket服务器和一个WebSocket客户端。服务器使用8080端口监听WebSocket连接,当接收到客户端发送的请求后,会读取特定路径下的图片并将二进制数据发送到客户端。
JavaScript的WebSocket客户端会连接到服务器,并发送"Please send the image"消息。当客户端接收到服务器发送的数据后,会将收到的二进制数据写入本地磁盘上的"receivedImage.jpg"文件中。
请注意替换服务器代码中的图片路径为实际的图片路径。
### 回答3:
netty是一个高效、可扩展、异步事件驱动的网络应用框架,它提供了对TCP和UDP传输协议的支持,并且还支持HTTP和WebSocket等高层协议。下面是一个使用netty实现WebSocket传输图片的示例代码:
```java
import io.netty.bootstrap.ServerBootstrap;
import io.netty.channel.ChannelFuture;
import io.netty.channel.ChannelHandlerContext;
import io.netty.channel.ChannelInboundHandlerAdapter;
import io.netty.channel.ChannelInitializer;
import io.netty.channel.ChannelPipeline;
import io.netty.channel.SimpleChannelInboundHandler;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.SocketChannel;
import io.netty.channel.socket.nio.NioServerSocketChannel;
import io.netty.handler.codec.http.HttpObjectAggregator;
import io.netty.handler.codec.http.HttpRequest;
import io.netty.handler.codec.http.HttpRequestDecoder;
import io.netty.handler.codec.http.HttpResponseEncoder;
import io.netty.handler.stream.ChunkedWriteHandler;
import io.netty.handler.codec.http.websocketx.WebSocketFrame;
import io.netty.handler.codec.http.websocketx.WebSocketFrameDecoder;
import io.netty.handler.codec.http.websocketx.WebSocketFrameEncoder;
import io.netty.handler.codec.http.websocketx.WebSocketHandshakeException;
import io.netty.handler.codec.http.websocketx.WebSocketServerHandshaker;
import io.netty.handler.codec.http.websocketx.WebSocketServerHandshakerFactory;
import io.netty.handler.codec.http.websocketx.BinaryWebSocketFrame;
import io.netty.handler.codec.http.websocketx.TextWebSocketFrame;
import io.netty.handler.codec.http.websocketx.WebSocketServerProtocolHandler;
public class WebSocketServer {
public static void main(String[] args) throws InterruptedException {
NioEventLoopGroup bossGroup = new NioEventLoopGroup();
NioEventLoopGroup workerGroup = new NioEventLoopGroup();
try {
ServerBootstrap serverBootstrap = new ServerBootstrap();
serverBootstrap.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new ChannelInitializer<SocketChannel>() {
protected void initChannel(SocketChannel ch) throws Exception {
ChannelPipeline pipeline = ch.pipeline();
pipeline.addLast(new HttpRequestDecoder());
pipeline.addLast(new HttpObjectAggregator(65536));
pipeline.addLast(new HttpResponseEncoder());
pipeline.addLast(new ChunkedWriteHandler());
pipeline.addLast(new WebSocketServerProtocolHandler("/websocket"));
pipeline.addLast(new WebSocketFrameDecoder());
pipeline.addLast(new WebSocketFrameEncoder());
pipeline.addLast(new SimpleChannelInboundHandler<WebSocketFrame>() {
protected void channelRead0(ChannelHandlerContext ctx, WebSocketFrame frame) throws Exception {
if (frame instanceof BinaryWebSocketFrame) {
BinaryWebSocketFrame binaryFrame = (BinaryWebSocketFrame) frame;
// 在这里处理接收到的二进制图片数据
// ...
}
}
public void userEventTriggered(ChannelHandlerContext ctx, Object evt) throws Exception {
if (evt instanceof HttpRequest) {
HttpRequest request = (HttpRequest) evt;
WebSocketServerHandshakerFactory factory =
new WebSocketServerHandshakerFactory("ws://localhost:8080/websocket", null, false);
WebSocketServerHandshaker handshaker = factory.newHandshaker(request);
if (handshaker == null) {
WebSocketServerHandshakerFactory.sendUnsupportedVersionResponse(ctx.channel());
} else {
try {
handshaker.handshake(ctx.channel(), request);
} catch (WebSocketHandshakeException e) {
e.printStackTrace();
}
}
}
}
});
}
});
ChannelFuture channelFuture = serverBootstrap.bind(8080).sync();
channelFuture.channel().closeFuture().sync();
} finally {
bossGroup.shutdownGracefully();
workerGroup.shutdownGracefully();
}
}
}
```
上述示例代码创建了一个WebSocket服务器,在端口8080上监听传入的WebSocket连接。当接受到二进制的WebSocket帧时,通过`channelRead0`方法进行图片数据的接收和处理。
请注意,以上只是一个简化的示例,实际上在处理图片数据时可能需要进行一些额外的处理,比如解码、保存等,具体情况需要根据自己的需求进行适当的修改和扩展。
相关推荐
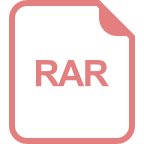
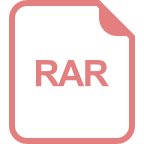
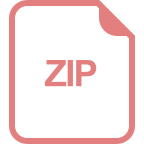














