用java gui编写一个实现加减乘除和求余的计算器,旁边有计算的历史记录上面的保存按钮可以保存到电脑文件里,还可以通过清除按钮清除历史记录
时间: 2024-01-22 11:18:52 浏览: 23
好的,这个任务需要用到 Java 的 GUI 编程和文件操作,我会给出一个参考实现,你可以参考着做。
首先,我们需要定义一个 JFrame 类作为主窗口,包含一个 JTextField 作为显示屏、若干个 JButton 作为数字键和操作键,还有一个 JTextArea 作为历史记录。
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
import java.io.*;
public class Calculator extends JFrame {
private JTextField display;
private JTextArea history;
private JButton saveButton, clearButton;
private double operand1, operand2;
private char operator;
public Calculator() {
super("Calculator");
// 初始化显示屏和历史记录
display = new JTextField(20);
display.setEditable(false);
history = new JTextArea(10, 20);
history.setEditable(false);
// 初始化数字键和操作键
JButton button0 = new JButton("0");
JButton button1 = new JButton("1");
JButton button2 = new JButton("2");
JButton button3 = new JButton("3");
JButton button4 = new JButton("4");
JButton button5 = new JButton("5");
JButton button6 = new JButton("6");
JButton button7 = new JButton("7");
JButton button8 = new JButton("8");
JButton button9 = new JButton("9");
JButton buttonAdd = new JButton("+");
JButton buttonSubtract = new JButton("-");
JButton buttonMultiply = new JButton("*");
JButton buttonDivide = new JButton("/");
JButton buttonMod = new JButton("%");
// 给数字键和操作键添加事件处理器
ActionListener digitListener = new DigitListener();
button0.addActionListener(digitListener);
button1.addActionListener(digitListener);
button2.addActionListener(digitListener);
button3.addActionListener(digitListener);
button4.addActionListener(digitListener);
button5.addActionListener(digitListener);
button6.addActionListener(digitListener);
button7.addActionListener(digitListener);
button8.addActionListener(digitListener);
button9.addActionListener(digitListener);
ActionListener operatorListener = new OperatorListener();
buttonAdd.addActionListener(operatorListener);
buttonSubtract.addActionListener(operatorListener);
buttonMultiply.addActionListener(operatorListener);
buttonDivide.addActionListener(operatorListener);
buttonMod.addActionListener(operatorListener);
// 初始化保存和清除按钮,并添加事件处理器
saveButton = new JButton("保存");
clearButton = new JButton("清除历史记录");
saveButton.addActionListener(new SaveListener());
clearButton.addActionListener(new ClearListener());
// 把数字键和操作键添加到网格布局中
JPanel buttonPanel = new JPanel(new GridLayout(4, 4));
buttonPanel.add(button7);
buttonPanel.add(button8);
buttonPanel.add(button9);
buttonPanel.add(buttonAdd);
buttonPanel.add(button4);
buttonPanel.add(button5);
buttonPanel.add(button6);
buttonPanel.add(buttonSubtract);
buttonPanel.add(button1);
buttonPanel.add(button2);
buttonPanel.add(button3);
buttonPanel.add(buttonMultiply);
buttonPanel.add(button0);
buttonPanel.add(buttonMod);
buttonPanel.add(buttonDivide);
buttonPanel.add(clearButton);
// 把显示屏和历史记录添加到垂直盒子布局中,并添加到主窗口中
Box box = Box.createVerticalBox();
box.add(display);
box.add(history);
box.add(saveButton);
box.add(buttonPanel);
getContentPane().add(box);
// 设置主窗口属性
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
pack();
setVisible(true);
}
// 数字键事件处理器
private class DigitListener implements ActionListener {
public void actionPerformed(ActionEvent e) {
String digit = ((JButton) e.getSource()).getText();
String text = display.getText();
if (text.equals("0")) {
display.setText(digit);
} else {
display.setText(text + digit);
}
}
}
// 操作键事件处理器
private class OperatorListener implements ActionListener {
public void actionPerformed(ActionEvent e) {
operand1 = Double.parseDouble(display.getText());
operator = ((JButton) e.getSource()).getText().charAt(0);
display.setText("0");
}
}
// 保存按钮事件处理器
private class SaveListener implements ActionListener {
public void actionPerformed(ActionEvent e) {
String historyText = history.getText();
if (historyText.equals("")) {
JOptionPane.showMessageDialog(null, "历史记录为空!");
return;
}
JFileChooser chooser = new JFileChooser();
int returnVal = chooser.showSaveDialog(null);
if (returnVal == JFileChooser.APPROVE_OPTION) {
File file = chooser.getSelectedFile();
try {
PrintWriter out = new PrintWriter(file);
out.print(historyText);
out.close();
JOptionPane.showMessageDialog(null, "保存成功!");
} catch (IOException ex) {
JOptionPane.showMessageDialog(null, "保存失败:" + ex.getMessage());
}
}
}
}
// 清除按钮事件处理器
private class ClearListener implements ActionListener {
public void actionPerformed(ActionEvent e) {
history.setText("");
}
}
public static void main(String[] args) {
new Calculator();
}
}
```
以上代码定义了一个 Calculator 类,继承自 JFrame 类,包含了数字键、操作键、保存按钮和清除按钮的事件处理器。
其中,数字键和操作键都绑定了 ActionListener,当用户点击它们时,会触发对应的事件处理器,将数字或操作符显示在显示屏上。
保存按钮绑定了 SaveListener,当用户点击它时,会弹出一个文件选择器,让用户选择要保存历史记录的文件,然后将历史记录写入文件。
清除按钮绑定了 ClearListener,当用户点击它时,会清除历史记录。
注意,在计算器中,我们需要记录当前操作数和操作符,因为用户可能会多次按数字键,最后才按操作键,所以我们需要在操作键事件处理器中记录下来当前的操作数和操作符,等到用户按下等于键时再进行计算。
还有一个需要注意的地方是,我们使用了 Box 布局来将显示屏、历史记录、保存按钮和数字键、操作键、清除按钮排列起来,这样可以更好地控制它们之间的间距和尺寸。
参考这个实现,你可以尝试自己写一个计算器并加入保存历史记录和清除历史记录的功能。
相关推荐
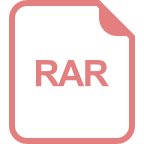














