Java代码实现Excel文件的导入,并且记录导入成功多少条,导入失败多少条,导入失败的数据记录在文件中
时间: 2023-06-02 11:06:25 浏览: 58
可以使用 Apache POI 库来实现 Java 代码导入 Excel 文件,记录导入成功和失败的条目数。具体步骤如下:
1. 引入 Apache POI 的依赖。
2. 读取 Excel 文件,遍历每一行数据。
3. 对于每一行数据,进行相应的数据处理,如类型转换等。
4. 对于处理后的数据,进行业务逻辑判断,判断是否满足导入条件。
5. 记录导入成功和失败的条目数。
6. 对于导入失败的数据,将其记录在文件中。
下面是一个示例代码:
```
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class ExcelImportExample {
public static void main(String[] args) {
String excelFilePath = "path/to/excel/file";
String failedDataFile = "path/to/failed/data/file";
int successCount = 0, failedCount = 0;
List<String> failedData = new ArrayList<>();
try (FileInputStream inputStream = new FileInputStream(new File(excelFilePath))) {
Workbook workbook = new XSSFWorkbook(inputStream);
Sheet sheet = workbook.getSheetAt(0);
Iterator<Row> iterator = sheet.iterator();
while (iterator.hasNext()) {
Row currentRow = iterator.next();
Iterator<Cell> cellIterator = currentRow.iterator();
// read data from cells and process
// ...
if (isDataValid(data)) {
// import data
successCount++;
} else {
// record failed data
failedData.add(data);
failedCount++;
}
}
workbook.close();
} catch (IOException e) {
e.printStackTrace();
}
// write failed data to file
try (FileOutputStream outputStream = new FileOutputStream(new File(failedDataFile))) {
for (String data : failedData) {
outputStream.write(data.getBytes());
outputStream.write(System.lineSeparator().getBytes());
}
} catch (IOException e) {
e.printStackTrace();
}
System.out.println("Import finished: " + successCount + " records imported successfully, " + failedCount + " records failed to import.");
}
private static boolean isDataValid(Data data) {
// perform necessary validation checks
// ...
}
}
```
相关推荐
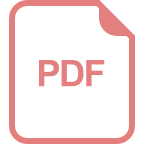














