给扫雷的Python代码
时间: 2024-02-09 22:02:01 浏览: 25
以下是一个简单的扫雷游戏的 Python 代码示例:
```python
import random
class Minesweeper:
def __init__(self, width=10, height=10, bombs=10):
self.width = width
self.height = height
self.bombs = bombs
self.board = [[0 for i in range(width)] for j in range(height)]
self.generate_board()
def generate_board(self):
bombs = 0
while bombs < self.bombs:
x = random.randint(0, self.width-1)
y = random.randint(0, self.height-1)
if self.board[y][x] == 0:
self.board[y][x] = -1
bombs += 1
for i in range(self.height):
for j in range(self.width):
if self.board[i][j] == -1:
continue
neighbors = self.get_neighbors(j, i)
neighbor_bombs = sum([1 for n in neighbors if self.board[n[1]][n[0]] == -1])
self.board[i][j] = neighbor_bombs
def get_neighbors(self, x, y):
neighbors = []
for i in range(max(y-1, 0), min(y+2, self.height)):
for j in range(max(x-1, 0), min(x+2, self.width)):
neighbors.append((j, i))
return neighbors
def print_board(self):
for i in range(self.height):
for j in range(self.width):
if self.board[i][j] == -1:
print('*', end=' ')
else:
print(self.board[i][j], end=' ')
print()
def reveal(self, x, y):
if self.board[y][x] == -1:
return False
elif self.board[y][x] > 0:
return True
else:
self.board[y][x] = -2
neighbors = self.get_neighbors(x, y)
for n in neighbors:
self.reveal(n[0], n[1])
return True
game = Minesweeper()
game.print_board()
while True:
x = int(input('Enter X coordinate: '))
y = int(input('Enter Y coordinate: '))
if not game.reveal(x, y):
print('Game over')
game.print_board()
break
else:
game.print_board()
if all([all([cell != 0 for cell in row]) for row in game.board]):
print('You win!')
break
```
这个代码创建了一个 Minesweeper 类来处理扫雷游戏的逻辑。在初始化时,它生成一个二维数组作为游戏板,并在随机位置放置炸弹。然后,它通过计算每个方格周围的炸弹数量来计算出每个方格的值。这个类还定义了一个 get_neighbors 方法来获取一个方格周围的所有邻居,并定义了一个 reveal 方法来展示一个方格的值。最后,它使用一个 while 循环来交互式地玩扫雷游戏。
相关推荐
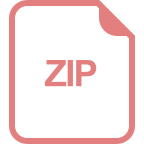
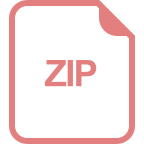












