用Python写出俄罗斯方块游戏
时间: 2023-11-16 11:09:46 浏览: 85
好的,以下是用Python实现俄罗斯方块游戏的示例代码:
```python
import pygame
import random
# 初始化pygame
pygame.init()
# 游戏区域大小
screen_width = 400
screen_height = 400
play_width = 300 # 游戏区域宽度
play_height = 600 # 游戏区域高度
block_size = 30 # 方块大小
# 定义颜色
white = (255, 255, 255)
black = (0, 0, 0)
gray = (128, 128, 128)
red = (255, 0, 0)
green = (0, 255, 0)
blue = (0, 0, 255)
yellow = (255, 255, 0)
# 初始化游戏区域
window = pygame.display.set_mode((screen_width, screen_height))
pygame.display.set_caption("俄罗斯方块")
# 定义字体
font = pygame.font.SysFont(None, 30)
# 方块类
class Block:
def __init__(self, x, y, color):
self.x = x
self.y = y
self.color = color
# 方块组合类
class Shape:
def __init__(self, x, y):
self.x = x
self.y = y
self.blocks = []
self.color = None
# 移动方块组合
def move(self, dx, dy):
for block in self.blocks:
block.x += dx
block.y += dy
# 旋转方块组合
def rotate(self):
if self.color == yellow:
return
center_x = self.blocks[0].x
center_y = self.blocks[0].y
for block in self.blocks:
x = block.x - center_x
y = block.y - center_y
block.x = center_x - y
block.y = center_y + x
# 创建方块组合
def create(self):
self.color = random.choice([red, green, blue, yellow])
shape = random.randint(0, 6)
if shape == 0: # I
self.blocks.append(Block(self.x, self.y, self.color))
self.blocks.append(Block(self.x + block_size, self.y, self.color))
self.blocks.append(Block(self.x + 2 * block_size, self.y, self.color))
self.blocks.append(Block(self.x + 3 * block_size, self.y, self.color))
elif shape == 1: # J
self.blocks.append(Block(self.x, self.y, self.color))
self.blocks.append(Block(self.x, self.y + block_size, self.color))
self.blocks.append(Block(self.x + block_size, self.y + block_size, self.color))
self.blocks.append(Block(self.x + 2 * block_size, self.y + block_size, self.color))
elif shape == 2: # L
self.blocks.append(Block(self.x + 2 * block_size, self.y, self.color))
self.blocks.append(Block(self.x, self.y + block_size, self.color))
self.blocks.append(Block(self.x + block_size, self.y + block_size, self.color))
self.blocks.append(Block(self.x + 2 * block_size, self.y + block_size, self.color))
elif shape == 3: # O
self.blocks.append(Block(self.x, self.y, self.color))
self.blocks.append(Block(self.x + block_size, self.y, self.color))
self.blocks.append(Block(self.x, self.y + block_size, self.color))
self.blocks.append(Block(self.x + block_size, self.y + block_size, self.color))
elif shape == 4: # S
self.blocks.append(Block(self.x + block_size, self.y, self.color))
self.blocks.append(Block(self.x + 2 * block_size, self.y, self.color))
self.blocks.append(Block(self.x, self.y + block_size, self.color))
self.blocks.append(Block(self.x + block_size, self.y + block_size, self.color))
elif shape == 5: # T
self.blocks.append(Block(self.x + block_size, self.y, self.color))
self.blocks.append(Block(self.x, self.y + block_size, self.color))
self.blocks.append(Block(self.x + block_size, self.y + block_size, self.color))
self.blocks.append(Block(self.x + 2 * block_size, self.y + block_size, self.color))
elif shape == 6: # Z
self.blocks.append(Block(self.x, self.y, self.color))
self.blocks.append(Block(self.x + block_size, self.y, self.color))
self.blocks.append(Block(self.x + block_size, self.y + block_size, self.color))
self.blocks.append(Block(self.x + 2 * block_size, self.y + block_size, self.color))
# 初始化游戏
def init_game():
global play_area, current_shape, next_shape, score, game_over
play_area = [[black for _ in range(10)] for _ in range(20)]
current_shape = Shape(3 * block_size, 0)
current_shape.create()
next_shape = Shape(play_width + block_size, block_size)
next_shape.create()
score = 0
game_over = False
# 绘制游戏界面
def draw_game():
window.fill(gray)
# 绘制游戏区域
for i in range(20):
for j in range(10):
pygame.draw.rect(window, play_area[i][j], (j * block_size, i * block_size, block_size, block_size), 0)
# 绘制当前方块组合
for block in current_shape.blocks:
pygame.draw.rect(window, block.color, (block.x, block.y, block_size, block_size), 0)
# 绘制下一个方块组合
for block in next_shape.blocks:
pygame.draw.rect(window, block.color, (block.x, block.y, block_size, block_size), 0)
# 绘制得分
score_text = font.render("Score: " + str(score), True, white)
window.blit(score_text, (play_width + block_size, 2 * block_size))
# 绘制游戏结束提示
if game_over:
game_over_text = font.render("Game Over", True, red)
window.blit(game_over_text, (play_width + block_size, 5 * block_size))
pygame.display.update()
# 检查是否可以移动方块组合
def can_move(dx, dy):
for block in current_shape.blocks:
x = block.x + dx
y = block.y + dy
if x < 0 or x >= play_width or y >= play_height or play_area[y // block_size][x // block_size] != black:
return False
return True
# 占用游戏区域
def occupy_area():
for block in current_shape.blocks:
play_area[block.y // block_size][block.x // block_size] = block.color
# 消除满行
def clear_rows():
global play_area, score
new_area = [[black for _ in range(10)] for _ in range(20)]
i = 19
for row in range(19, -1, -1):
if black not in play_area[row]:
score += 10
else:
new_area[i] = play_area[row]
i -= 1
play_area = new_area
# 主循环
def main_loop():
global current_shape, next_shape, game_over
clock = pygame.time.Clock()
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
if can_move(-block_size, 0):
current_shape.move(-block_size, 0)
elif event.key == pygame.K_RIGHT:
if can_move(block_size, 0):
current_shape.move(block_size, 0)
elif event.key == pygame.K_DOWN:
if can_move(0, block_size):
current_shape.move(0, block_size)
elif event.key == pygame.K_UP:
current_shape.rotate()
# 移动方块组合
if can_move(0, block_size):
current_shape.move(0, block_size)
else:
occupy_area()
clear_rows()
if current_shape.blocks[0].y <= 0:
game_over = True
break
current_shape = next_shape
current_shape.x = 3 * block_size
current_shape.y = 0
next_shape = Shape(play_width + block_size, block_size)
next_shape.create()
# 绘制游戏界面
draw_game()
# 设置游戏帧率
clock.tick(10)
# 启动游戏
init_game()
main_loop()
```
希望这个示例代码能够帮助你实现俄罗斯方块游戏。
阅读全文
相关推荐
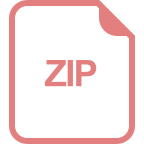








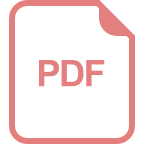
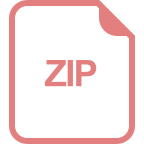
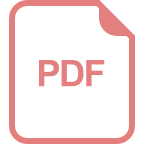
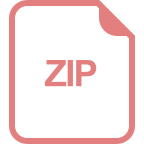



