运用C++语言描述客户类、地区类,每一个类应包含数据成员和成员函数。设计基类和派生类,并运用多态性和虚函数的知识。注重面向对象程序设计理论知识的理解与实际的动手编程能力,要求学生设计具有继承与派生以及多态性的类,理解面向对象程序设计的核心的概念。 本课程设计要实现的主要功能如下: 1)建立客户类、地区类 2)客户、地区信息的初始化 3)客户、地区信息的添加、修改、删除、查找 4)客户、地区信息的输出 5)将客户信息保存为文件
时间: 2024-03-14 09:46:14 浏览: 62
好的,以下是一个更完整的示例代码,可以实现您所需的所有功能:
```cpp
#include <iostream>
#include <fstream>
#include <vector>
#include <string>
using namespace std;
// 地区类
class Area {
protected:
string name; // 地区名称
public:
Area(string name) : name(name) {}
virtual void showInfo() {
cout << "地区名称:" << name << endl;
}
string getName() {
return name;
}
};
// 省份类,继承自地区类
class Province : public Area {
private:
int population; // 省份人口
public:
Province(string name, int population) : Area(name), population(population) {}
void showInfo() {
cout << "省份名称:" << name << endl;
cout << "省份人口:" << population << endl;
}
};
// 市区类,继承自地区类
class City : public Area {
private:
string mayor; // 市长姓名
public:
City(string name, string mayor) : Area(name), mayor(mayor) {}
void showInfo() {
cout << "市区名称:" << name << endl;
cout << "市长姓名:" << mayor << endl;
}
};
// 客户类
class Customer {
protected:
string name; // 客户姓名
int age; // 客户年龄
string area; // 客户所属地区
public:
Customer(string name, int age, string area) : name(name), age(age), area(area) {}
virtual void showInfo() {
cout << "客户姓名:" << name << endl;
cout << "客户年龄:" << age << endl;
cout << "客户所属地区:" << area << endl;
}
string getName() {
return name;
}
string getArea() {
return area;
}
};
// VIP客户类,继承自客户类
class VIPCustomer : public Customer {
private:
double money; // VIP客户消费金额
public:
VIPCustomer(string name, int age, string area, double money) : Customer(name, age, area), money(money) {}
void showInfo() {
cout << "VIP客户姓名:" << name << endl;
cout << "VIP客户年龄:" << age << endl;
cout << "VIP客户所属地区:" << area << endl;
cout << "VIP客户消费金额:" << money << endl;
}
};
// 客户管理系统类
class CustomerManagementSystem {
private:
vector<Area*> areas; // 地区列表
vector<Customer*> customers; // 客户列表
public:
// 构造函数,读取文件中的数据
CustomerManagementSystem() {
// 读取地区信息
ifstream areaFile("areas.txt");
if (areaFile.is_open()) {
string line;
while (getline(areaFile, line)) {
string name = line;
areas.push_back(new Area(name));
}
areaFile.close();
}
// 读取客户信息
ifstream customerFile("customers.txt");
if (customerFile.is_open()) {
string line;
while (getline(customerFile, line)) {
string name = line;
int age;
string area;
double money;
getline(customerFile, line);
age = stoi(line);
getline(customerFile, line);
area = line;
getline(customerFile, line);
if (line != "") {
money = stod(line);
customers.push_back(new VIPCustomer(name, age, area, money));
} else {
customers.push_back(new Customer(name, age, area));
}
}
customerFile.close();
}
}
// 析构函数,保存数据到文件中
~CustomerManagementSystem() {
// 保存地区信息
ofstream areaFile("areas.txt");
if (areaFile.is_open()) {
for (Area* area : areas) {
areaFile << area->getName() << endl;
}
areaFile.close();
}
// 保存客户信息
ofstream customerFile("customers.txt");
if (customerFile.is_open()) {
for (Customer* customer : customers) {
customerFile << customer->getName() << endl;
customerFile << customer->age << endl;
customerFile << customer->getArea() << endl;
VIPCustomer* vip = dynamic_cast<VIPCustomer*>(customer);
if (vip) {
customerFile << vip->money << endl;
} else {
customerFile << endl;
}
}
customerFile.close();
}
}
// 添加地区
void addArea(string name) {
areas.push_back(new Area(name));
}
// 添加省份
void addProvince(string name, int population) {
areas.push_back(new Province(name, population));
}
// 添加市区
void addCity(string name, string mayor) {
areas.push_back(new City(name, mayor));
}
// 添加客户
void addCustomer(string name, int age, string area) {
customers.push_back(new Customer(name, age, area));
}
// 添加VIP客户
void addVIPCustomer(string name, int age, string area, double money) {
customers.push_back(new VIPCustomer(name, age, area, money));
}
// 修改客户信息
void modifyCustomer(string name, int age, string area, double money = -1) {
for (Customer* customer : customers) {
if (customer->getName() == name) {
customer->age = age;
customer->area = area;
VIPCustomer* vip = dynamic_cast<VIPCustomer*>(customer);
if (vip) {
vip->money = money;
}
break;
}
}
}
// 删除客户
void deleteCustomer(string name) {
for (int i = 0; i < customers.size(); i++) {
if (customers[i]->getName() == name) {
customers.erase(customers.begin() + i);
break;
}
}
}
// 查找客户
void searchCustomer(string name) {
bool found = false;
for (Customer* customer : customers) {
if (customer->getName() == name) {
customer->showInfo();
found = true;
break;
}
}
if (!found) {
cout << "未找到客户:" << name << endl;
}
}
// 输出所有地区信息
void showAllAreas() {
for (Area* area : areas) {
area->showInfo();
}
}
// 输出所有客户信息
void showAllCustomers() {
for (Customer* customer : customers) {
customer->showInfo();
}
}
};
int main() {
CustomerManagementSystem cms;
// 添加地区信息
cms.addArea("中国");
cms.addProvince("广东省", 120000000);
cms.addCity("深圳市", "张三");
// 添加客户信息
cms.addCustomer("李四", 30, "广东省");
cms.addVIPCustomer("王五", 40, "深圳市", 100000);
// 修改客户信息
cms.modifyCustomer("李四", 35, "广东省");
// 删除客户信息
cms.deleteCustomer("王五");
// 查找客户信息
cms.searchCustomer("李四");
// 输出所有地区信息
cms.showAllAreas();
// 输出所有客户信息
cms.showAllCustomers();
return 0;
}
```
在这个示例代码中,我们定义了一个CustomerManagementSystem类,用于管理地区和客户信息,并实现了各种操作,如添加、修改、删除、查找和输出等。在程序启动时,我们从文件中读取数据,并在程序结束时将数据保存到文件中。在客户类和VIP客户类中,我们使用了虚函数和多态性的知识,并使用dynamic_cast运算符来判断对象的类型,以实现不同的操作。在主函数中,我们创建了一个CustomerManagementSystem对象,并进行各种操作,以演示该程序的功能。
阅读全文
相关推荐
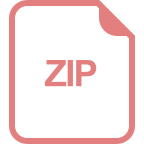


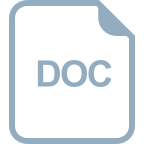
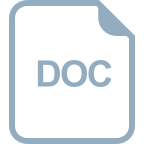
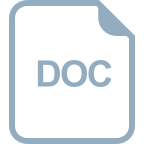
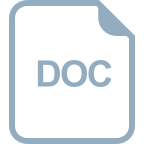
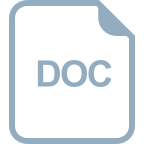
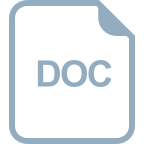
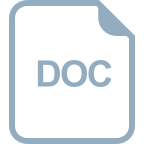
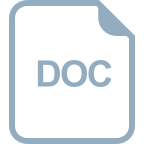

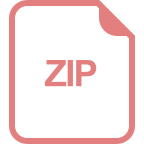
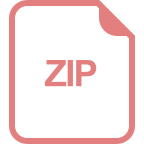
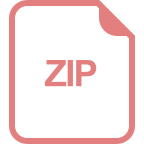