if data.shape[0] != config.batch_size是什么意思
时间: 2023-12-27 18:02:22 浏览: 34
这个if语句的意思是,如果data的行数不等于config中指定的batch_size,则执行下面的语句。其中,data是一个数据矩阵或数组,而config是一个配置文件或类,其中包含了程序运行的各种参数。在机器学习中,通常会按照batch_size批量读取数据,每次读取一个batch_size大小的数据,对其进行处理或训练,以加快计算速度和提高模型性能。因此,这个if语句的作用就是确保读取的数据量是batch_size的整数倍。
相关问题
怎么加快这段代码的运行速度num_samples = x_data.shape[0] features = tf.Variable(initial_value=tf.zeros((1, 0)), dtype=tf.float32) batch_size = 32 for i in range(0, num_samples, batch_size): batch = x_data[i:i + batch_size] batch = tf.expand_dims(batch, axis=0) if i + batch_size > num_samples: batch_num = num_samples - i if batch_num == 1: feature_batch = model.predict(batch.reshape(1, *input_shape), batch_size=batch_num) else: feature_batch = model.predict(batch, batch_size=batch_num) features = tf.concat([features, feature_batch], axis=1) else: feature_batch = model.predict(batch, batch_size=batch_size) features = tf.concat([features, feature_batch], axis=1) print(features.shape)
这段代码的运行速度可以通过以下几种方式进行优化:
1. 使用更快的模型:如果模型的计算速度较慢,可以尝试使用更快的模型,例如MobileNet、EfficientNet等。
2. 使用GPU加速:如果你的电脑有GPU,可以使用GPU加速模型的计算。可以使用`tf.config.experimental.set_memory_growth()`方法来设置GPU内存分配策略,以避免内存溢出问题。
3. 使用TensorFlow Dataset:TensorFlow Dataset是一种高效的数据输入管道,可以帮助提高训练速度。可以使用`tf.data.Dataset.from_tensor_slices()`方法来创建一个Dataset对象,然后使用`batch()`方法和`prefetch()`方法来设置批量大小和预取数据。
4. 使用更大的批量大小:如果你的电脑有足够的内存,可以尝试使用更大的批量大小,以提高训练速度。但是需要注意,批量大小过大可能会导致内存溢出问题。
5. 使用多线程预处理数据:如果你的电脑有多个CPU核心,可以使用多线程预处理数据,以加快数据处理速度。可以使用`tf.data.Dataset.map()`方法来定义一个数据预处理函数,并使用`num_parallel_calls`参数来设置线程数。
根据你的代码,可以使用方法1、2和4来进行优化,具体代码如下:
```python
# 方法1:使用更快的模型
from tensorflow.keras.applications import MobileNetV2
model = MobileNetV2(input_shape=input_shape, include_top=False, weights='imagenet')
# 方法2:使用GPU加速
gpus = tf.config.list_physical_devices('GPU')
if gpus:
try:
tf.config.experimental.set_memory_growth(gpus[0], True)
except RuntimeError as e:
print(e)
# 方法4:使用更大的批量大小
batch_size = 64
# 修改后的代码
features = tf.Variable(initial_value=tf.zeros((1, 0)), dtype=tf.float32)
for i in range(0, num_samples, batch_size):
batch = x_data[i:i + batch_size]
batch = tf.expand_dims(batch, axis=0)
if i + batch_size > num_samples:
batch_num = num_samples - i
if batch_num == 1:
feature_batch = model.predict(batch.reshape(1, *input_shape), batch_size=batch_num)
else:
feature_batch = model.predict(batch, batch_size=batch_num)
features = tf.concat([features, feature_batch], axis=1)
else:
feature_batch = model.predict(batch, batch_size=batch_size)
features = tf.concat([features, feature_batch], axis=1)
print(features.shape)
```
希望这些方法能够帮助你加快代码的运行速度!
请问是如下修改吗 : data1 = Data_1H(data,config.Hx) trainX1,trainX2,_ = data1._slice_multi() data2 = Data_1H(data_y,config.Hx) _ , _, trainY = data2._slice_multi() trainY = np.expand_dims(trainY, axis=-1) # Y 的形状就由(155,5)变成了 (155, 5, 1),可以用于训练和预测模型 print("trainX Y shape is:",trainX1.shape,trainX2.shape,trainY.shape, "Hx=",config.Hx ) if len(trainY.shape) == 1: trainY = trainY.reshape(-1,1) train_data = [(trainX1[i], trainX2[i], trainY[i]) for i in range(trainX1.shape[0])] batch_size = 32 dataset = tf.data.Dataset.from_generator(lambda: iter(train_data), output_types=(tf.float32, tf.float32, tf.float32), output_shapes=((None, trainX1.shape[1]), (None, trainX2.shape[1]), (None, 1))) dataset = dataset.batch(batch_size) # 进行训练 #model = trainModel(trainX1, trainX2 , trainY, config) model = LSTNetAttention(trainX1,trainX2,trainY,config) model.summary() model.compile(optimizer=config.optimizer, loss=config.loss_metric) #model.compile(optimizer=Adam(lr=0.001), loss=config.loss_metric) model.fit([trainX1,trainX2], trainY, epochs=config.epochs, steps_per_epoch=len(train_data) ,batch_size=config.lstm_batch_size, verbose=config.verbose,validation_split=0.2, callbacks=[my_early_stopping]) #=====================================
这段代码主要是用于处理数据并训练模型的。首先对数据进行预处理,使用`Data_1H`类对数据进行处理,得到`trainX1`、`trainX2`和`trainY`。然后对`trainY`进行维度扩展,使其变为`(155, 5, 1)`的形状,方便后续训练和预测模型。接着将数据转化为`tf.data.Dataset`类型,并设置批次大小为`batch_size`。最后使用`LSTNetAttention`模型对数据进行训练,使用`my_early_stopping`回调函数进行早停操作。
相关推荐
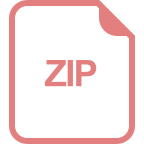
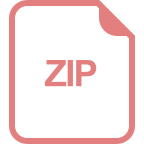












