Write a loop to read as many as numbers till you enter '-99' (if your input is -99 go out from the loop), and find a) how many numbers Less than 50. b) how many numbers Greater than 100. c) how many +ve numbers d) how many -ve numbers e) how many numbers in between 51 and 99.use java
时间: 2024-03-06 09:50:02 浏览: 44
Sure, here's the Java code to solve your problem:
```java
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
// Initializing variables
int numLessThan50 = 0;
int numGreaterThan100 = 0;
int numPositive = 0;
int numNegative = 0;
int numBetween51And99 = 0;
// Loop to read input numbers
while (true) {
System.out.print("Enter a number (or -99 to exit): ");
int num = input.nextInt();
if (num == -99) {
break;
}
// Checking conditions
if (num < 50) {
numLessThan50++;
} else if (num > 100) {
numGreaterThan100++;
}
if (num > 0) {
numPositive++;
} else if (num < 0) {
numNegative++;
}
if (num > 51 && num < 99) {
numBetween51And99++;
}
}
// Printing the results
System.out.println("Numbers less than 50: " + numLessThan50);
System.out.println("Numbers greater than 100: " + numGreaterThan100);
System.out.println("Positive numbers: " + numPositive);
System.out.println("Negative numbers: " + numNegative);
System.out.println("Numbers between 51 and 99: " + numBetween51And99);
}
}
```
You can run this code and enter numbers as many times as you want until you enter -99. The program will then display how many numbers are less than 50, greater than 100, positive, negative, and between 51 and 99.
相关推荐
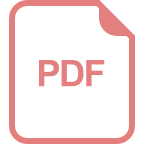
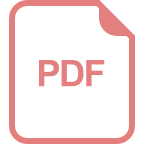
















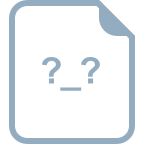