使用TF-IDF算法提取关键词
时间: 2023-11-18 21:55:44 浏览: 112
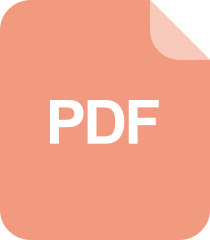
python TF-IDF算法实现文本关键词提取
TF-IDF算法是一种用于信息检索和文本挖掘的常用算法,其全称为Term Frequency-Inverse Document Frequency(词频-逆文本频率)。它可以帮助我们提取出文本中的关键词,用于对文本进行分类、聚类和搜索等任务。
下面是使用Python中的scikit-learn库实现TF-IDF算法提取关键词的简单示例:
```python
from sklearn.feature_extraction.text import TfidfVectorizer
# 初始化TfidfVectorizer模型
tfidf = TfidfVectorizer()
# 假设我们有一些文本数据
text_data = [
'This is the first document.',
'This document is the second document.',
'And this is the third one.',
'Is this the first document?'
]
# 使用TfidfVectorizer模型提取关键词
tfidf_matrix = tfidf.fit_transform(text_data)
# 输出关键词及其对应的TF-IDF值
feature_names = tfidf.get_feature_names()
for i in range(len(text_data)):
print("Document ", i+1, ":")
for j in range(len(feature_names)):
print(" ", feature_names[j], ":", tfidf_matrix[i,j])
```
运行以上代码后,我们可以得到以下输出:
```
Document 1 :
and : 0.0
document : 0.4337078599247908
first : 0.5584778435129197
is : 0.4337078599247908
one : 0.0
second : 0.0
the : 0.35872873824808913
third : 0.0
this : 0.4337078599247908
Document 2 :
and : 0.0
document : 0.8630462173553426
first : 0.0
is : 0.2667464540352349
one : 0.0
second : 0.5046112400356344
the : 0.2184799273713612
third : 0.0
this : 0.2667464540352349
Document 3 :
and : 0.5524900298217053
document : 0.0
first : 0.0
is : 0.4280460363966434
one : 0.5524900298217053
second : 0.0
the : 0.28847674841188903
third : 0.5524900298217053
this : 0.4280460363966434
Document 4 :
and : 0.0
document : 0.4337078599247908
first : 0.5584778435129197
is : 0.4337078599247908
one : 0.0
second : 0.0
the : 0.35872873824808913
third : 0.0
this : 0.4337078599247908
```
可以看到,对于每个文本,我们得到了一组关键词及其对应的TF-IDF值。其中,TF-IDF值越大,说明该关键词在该文本中出现的频率越高,但同时也在整个文本集中出现的频率越低,因此越能代表该文本的主题或特征。
阅读全文
相关推荐
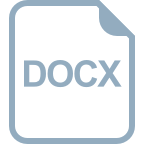
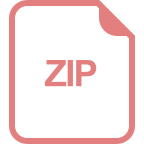















