给定成功检索概率和失败检索概率,用c++实现最优二叉搜索树先根序列打印
时间: 2024-03-22 07:39:27 浏览: 19
以下是用C++实现最优二叉搜索树打印先根序列的代码:
```cpp
#include <iostream>
#include <vector>
#include <limits>
using namespace std;
void printOptimalBST(vector<vector<int>>& root, int i, int j, int parent) {
if (i > j) {
return;
}
int rootIdx = root[i][j];
if (parent == 0) {
cout << "k" << rootIdx << " is the root" << endl;
} else {
cout << "k" << rootIdx << " is the left child of " << "k" << parent << endl;
}
printOptimalBST(root, i, rootIdx - 1, rootIdx);
printOptimalBST(root, rootIdx + 1, j, rootIdx);
}
void optimalBST(double p[], double q[], int n) {
double e[n + 1][n + 1], w[n + 1][n + 1];
vector<vector<int>> root(n + 1, vector<int>(n + 1, 0));
for (int i = 1; i <= n + 1; i++) {
e[i - 1][i - 1] = q[i - 1];
w[i - 1][i - 1] = q[i - 1];
}
for (int len = 1; len <= n; len++) {
for (int i = 0; i <= n - len; i++) {
int j = i + len;
e[i][j] = numeric_limits<double>::max();
w[i][j] = w[i][j - 1] + p[j] + q[j];
for (int k = i; k < j; k++) {
double temp = e[i][k] + e[k + 1][j] + w[i][j];
if (temp < e[i][j]) {
e[i][j] = temp;
root[i][j] = k;
}
}
}
}
cout << "The optimal BST is: " << endl;
printOptimalBST(root, 0, n - 1, 0);
}
int main() {
double p[] = {0.15, 0.10, 0.05, 0.10, 0.20};
double q[] = {0.05, 0.10, 0.05, 0.05, 0.05, 0.10};
int n = sizeof(p) / sizeof(p[0]);
optimalBST(p, q, n - 1);
return 0;
}
```
其中,`optimalBST`函数实现了最优二叉搜索树的构建,`printOptimalBST`函数实现了先根序列的打印。在`main`函数中,我们给出了一个例子,其中$p$数组为成功检索概率,$q$数组为失败检索概率。
相关推荐
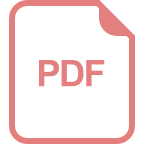
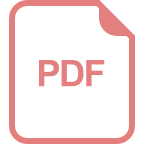
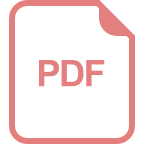














