def actionIncreasePointSize(self): self.vtk_widget.PointSize +=1 self.vtk_widget.PointSize %= 10 self.vtk_widget.actor.GetProperty().SetPointSize(self.vtk_widget.PointSize) self.vtk_widget.render_window.Render()
时间: 2024-04-05 20:33:49 浏览: 100
这是一个Python方法的代码实现,名为`actionIncreasePointSize`,它没有返回值。该方法的作用是增加字形的大小。具体实现步骤如下:
1. 将`vtk_widget`对象的`PointSize`属性加1。
2. 将`vtk_widget`对象的`PointSize`属性对10取模,以保证大小在0-9之间循环。
3. 调用`vtk_widget`对象的`actor`属性的`GetProperty`方法获取属性对象,然后调用`SetPointSize`方法设置字形的大小。
4. 调用`vtk_widget`对象的`render_window`属性的`Render`方法更新渲染窗口。
相关问题
# self.actor = vtk.vtkLODActor() self.actor = vtk.vtkActor() self.actor.SetMapper(mapper) # self.actor.SetNumberOfCloudPoints(1000) self.actor.GetProperty().SetPointSize(self.PointSize) # self.actor.PickableOff() outline = vtk.vtkOutlineFilter() outline.SetInputConnection(vertex.GetOutputPort()) mapper2 = vtk.vtkPolyDataMapper() mapper2.SetInputConnection(outline.GetOutputPort())
vtkLODActor和vtkActor都是VTK中的可视化对象,可以用于渲染vtk数据。vtkLODActor是vtkActor的一个特殊子类,可以根据距离调整渲染的细节,从而提高渲染速度。而vtkActor是最常用的可视化对象,它可以渲染任何类型的vtk数据。
vtkOutlineFilter是将vtk数据集转换为表示其边界的多边形数据集的过程。mapper2则是将vtkPolyData转换为可供渲染的图形元素的过程,与之前的mapper类似。在这段代码中,vtkOutlineFilter用于创建一个边框,以便在渲染时更好地显示数据的范围。mapper2则将vtkOutlineFilter的输出连接到vtkActor中,以便vtkActor可以渲染边框。
void QtWidgetsApplication2::pt_clicked(QString data1, QString data2) { pcl::console::TicToc time; // --------------------------------读取点云------------------------------------ pcl::PointCloud<pcl::PointXYZ>::Ptr cloud(new pcl::PointCloud<pcl::PointXYZ>); if (pcl::io::loadPCDFile<pcl::PointXYZ>("opened_cloud.pcd", *cloud) == -1) { PCL_ERROR("Cloudn't read file!"); } //cout << "滤波前点的个数为:" << cloud->size() << endl; // --------------------------------直通滤波------------------------------------ float a = data1.toFloat(); float b = data2.toFloat(); pcl::PointCloud<pcl::PointXYZ>::Ptr filtered(new pcl::PointCloud<pcl::PointXYZ>); std::string fv = "z"; // 滤波字段 filtered = pcl_filter_passthrough(cloud, a, b, fv); //cout << "直通滤波用时:" << time.toc() << " ms" << endl; pcl::io::savePCDFileASCII("opened_cloud.pcd", *filtered); ui.textBrowser->clear(); QString Pointsize = QString("%1").arg(cloud->points.size()); ui.textBrowser->insertPlainText(QStringLiteral("点云数量:") + Pointsize); QString Pointsize1 = QString("%1").arg(filtered->points.size()); ui.textBrowser->insertPlainText(QStringLiteral("\n滤波后点云数量:") + Pointsize1); auto renderer2 = vtkSmartPointer<vtkRenderer>::New(); auto renderWindow2 = vtkSmartPointer<vtkGenericOpenGLRenderWindow>::New(); renderWindow2->AddRenderer(renderer2); viewer.reset(new pcl::visualization::PCLVisualizer(renderer2, renderWindow2, "viewer", false)); ui.openGLWidget->setRenderWindow(viewer->getRenderWindow()); viewer->setupInteractor(ui.openGLWidget->interactor(), ui.openGLWidget->renderWindow()); viewer->setBackgroundColor(0, 0, 0); //设置背景 pcl::visualization::PointCloudColorHandlerGenericField<pcl::PointXYZ> fildColor(filtered, "z"); viewer->addPointCloud<pcl::PointXYZ>(filtered, fildColor, "sample cloud"); viewer->setPointCloudRenderingProperties(pcl::visualization::PCL_VISUALIZER_POINT_SIZE, 1, "sample cloud"); viewer->resetCamera(); update(); };这段代码存在内存泄漏的问题
在这段代码中,存在内存泄漏的问题,主要是在以下两个地方:
1. 在使用`pcl_filter_passthrough`函数时,返回的`filtered`点云指针被赋值给了`filtered`智能指针,但是在函数结束时,`filtered`智能指针没有被释放,导致内存泄漏。
2. 在使用`viewer`对象时,通过`reset`函数将其重新初始化,但是在此之前并没有释放其之前的内存,也就是说,之前分配的内存没有被正确释放,导致内存泄漏。
为了解决这个问题,可以在使用`pcl_filter_passthrough`函数时,将返回的点云指针直接赋值给`filtered`智能指针,这样在函数结束时,智能指针会自动释放其所指向的内存。同时,在使用`viewer`对象时,可以先调用`removeAllPointClouds`函数,将之前的点云对象从视图中移除,然后再调用`reset`函数重新初始化`viewer`对象,这样可以避免内存泄漏。修改后的代码如下:
```
void QtWidgetsApplication2::pt_clicked(QString data1, QString data2) {
pcl::console::TicToc time;
// --------------------------------读取点云------------------------------------
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud(new pcl::PointCloud<pcl::PointXYZ>);
if (pcl::io::loadPCDFile<pcl::PointXYZ>("opened_cloud.pcd", *cloud) == -1)
{
PCL_ERROR("Cloudn't read file!");
}
//cout << "滤波前点的个数为:" << cloud->size() << endl;
// --------------------------------直通滤波------------------------------------
float a = data1.toFloat();
float b = data2.toFloat();
std::string fv = "z"; // 滤波字段
pcl::PointCloud<pcl::PointXYZ>::Ptr filtered = pcl_filter_passthrough(cloud, a, b, fv);
//cout << "直通滤波用时:" << time.toc() << " ms" << endl;
pcl::io::savePCDFileASCII("opened_cloud.pcd", *filtered);
ui.textBrowser->clear();
QString Pointsize = QString("%1").arg(cloud->points.size());
ui.textBrowser->insertPlainText(QStringLiteral("点云数量:") + Pointsize);
QString Pointsize1 = QString("%1").arg(filtered->points.size());
ui.textBrowser->insertPlainText(QStringLiteral("\n滤波后点云数量:") + Pointsize1);
viewer->removeAllPointClouds(); //移除之前的点云对象
viewer->resetCamera();
pcl::visualization::PointCloudColorHandlerGenericField<pcl::PointXYZ> fildColor(filtered, "z");
viewer->addPointCloud<pcl::PointXYZ>(filtered, fildColor, "sample cloud");
viewer->setPointCloudRenderingProperties(pcl::visualization::PCL_VISUALIZER_POINT_SIZE, 1, "sample cloud");
ui.openGLWidget->update();
};
```
阅读全文
相关推荐
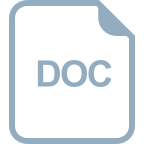
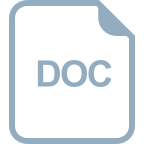
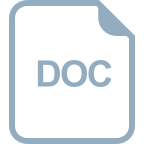
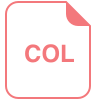
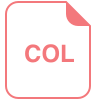
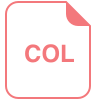










