tf.nn.softmax
时间: 2023-11-06 18:45:51 浏览: 24
The tf.nn.softmax function is a mathematical function used in deep learning and machine learning models for classification problems. It takes in one or more inputs and produces a probability distribution over a set of possible outcomes. Specifically, it applies an exponential function to each input value to ensure that the output probabilities sum up to 1.0. It is commonly used as the final activation function in neural networks for multi-class classification tasks.
相关问题
tf.nn.softmax与tf.layer.softmax有区别吗
tf.nn.softmax和tf.layers.softmax都是TensorFlow中实现softmax函数的方法,但它们的使用方式和一些功能上有一些区别。
tf.nn.softmax是一个比较底层的函数,它接受一个Tensor作为输入,返回对应的softmax值。tf.nn.softmax不会创建任何变量或层级结构,也不会进行任何参数的初始化或学习,因此它需要手动设置输入输出的shape。
tf.layers.softmax是在较高层次上实现的softmax函数,它可以很方便地嵌入到神经网络中。tf.layers.softmax不仅可以进行softmax计算,还可以对输入数据进行一些预处理操作,如dropout等。tf.layers.softmax还能自动处理变量的初始化和管理,并且会根据需要自动调整输入输出的shape。但是,需要注意的是,tf.layers.softmax在某些情况下可能会与其他层的激活函数冲突。
综上所述,虽然tf.nn.softmax和tf.layers.softmax都可以实现softmax函数,但在使用时需要根据具体情况选择合适的方法。如果需要底层的操作控制和灵活性,则选择使用tf.nn.softmax;如果需要快速构建神经网络并且不需要太多控制,则选择使用tf.layers.softmax。
tf.nn.softmax是什么作用
tf.nn.softmax是TensorFlow中的一个函数,它用于计算softmax激活函数。softmax函数可以将一个K维的实数向量转换为K维的概率分布,使每个元素的值在0到1之间,并且所有元素的和为1。
在机器学习中,softmax函数通常被用于多分类问题,将模型的输出转换为类别的概率分布。例如,对于一个3类分类问题,模型的输出是一个长度为3的向量,通过softmax函数将其转换为3个类别的概率分布,然后可以选择概率最大的类别作为预测结果。
在TensorFlow中,可以使用tf.nn.softmax函数来计算softmax激活函数,它的输入是一个张量,输出也是一个张量,表示softmax函数的输出结果。
相关推荐
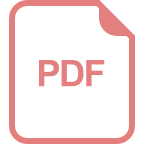
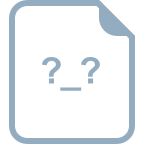
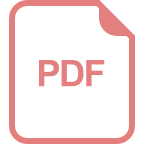













