用Java编写求π包含并行和串行并且得出消耗时间给代码加注释
时间: 2024-03-08 10:47:35 浏览: 29
好的,我可以为您提供Java代码来计算π的值,其中包含并行和串行实现,并且会记录消耗时间。以下是代码和注释:
```java
import java.util.concurrent.*;
public class PiCalculator {
public static void main(String[] args) {
int numSteps = 10000000; // 迭代次数
// 串行计算π
long startSerial = System.currentTimeMillis();
double piSerial = calculatePiSerial(numSteps);
long endSerial = System.currentTimeMillis();
System.out.println("Serial pi = " + piSerial + ", time = " + (endSerial - startSerial) + " ms");
// 并行计算π
long startParallel = System.currentTimeMillis();
double piParallel = calculatePiParallel(numSteps);
long endParallel = System.currentTimeMillis();
System.out.println("Parallel pi = " + piParallel + ", time = " + (endParallel - startParallel) + " ms");
}
// 串行计算π的方法
public static double calculatePiSerial(int numSteps) {
double sum = 0.0;
double step = 1.0 / (double) numSteps;
for (int i = 0; i < numSteps; i++) {
double x = ((double)i + 0.5) * step;
sum += 4.0 / (1.0 + x * x);
}
return step * sum;
}
// 并行计算π的方法
public static double calculatePiParallel(int numSteps) {
int numThreads = Runtime.getRuntime().availableProcessors(); // 获取CPU核心数
ExecutorService executor = Executors.newFixedThreadPool(numThreads);
double sum = 0.0;
double step = 1.0 / (double) numSteps;
int numPerThread = numSteps / numThreads;
int leftOver = numSteps % numThreads;
// 创建并执行所有线程
Future<Double>[] results = new Future[numThreads];
int start = 0;
int end = numPerThread;
for (int i = 0; i < numThreads; i++) {
if (i == numThreads - 1) {
end += leftOver;
}
results[i] = executor.submit(new PiWorker(start, end, step));
start = end;
end += numPerThread;
}
// 等待所有线程完成并累加结果
for (int i = 0; i < numThreads; i++) {
try {
sum += results[i].get();
} catch (InterruptedException | ExecutionException e) {
e.printStackTrace();
}
}
executor.shutdown();
return step * sum;
}
// 计算π的线程类
private static class PiWorker implements Callable<Double> {
private final int start;
private final int end;
private final double step;
public PiWorker(int start, int end, double step) {
this.start = start;
this.end = end;
this.step = step;
}
@Override
public Double call() throws Exception {
double sum = 0.0;
for (int i = start; i < end; i++) {
double x = ((double)i + 0.5) * step;
sum += 4.0 / (1.0 + x * x);
}
return sum;
}
}
}
```
代码中包含了两个方法:`calculatePiSerial` 和 `calculatePiParallel`,前者使用串行方式计算π,后者使用并行方式计算π。`calculatePiParallel` 方法使用了 Java 的 Executor 框架来创建并发任务,每个线程计算一部分的结果,最后将结果累加起来得到最终的π值。
代码中同时记录了计算时间,可以通过比较串行和并行的时间来了解并行计算的效率。
相关推荐
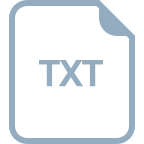














